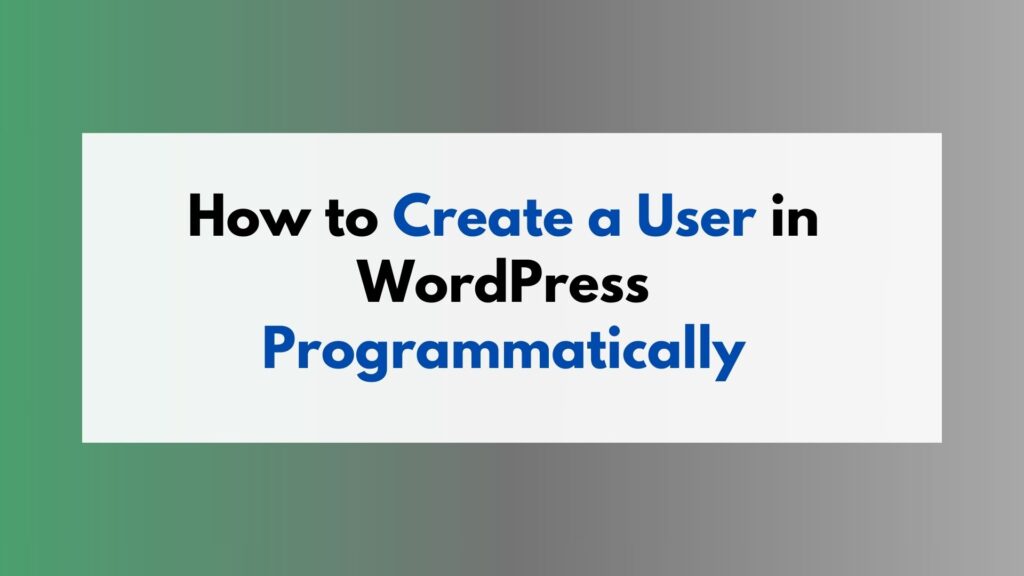
In this article, we will explore the process of creating a user in WordPress programmatically. We will discuss the importance of this feature and provide step-by-step instructions along with real use cases and example codes to help you understand its practical application.
Creating users in WordPress programmatically allows you to automate the user registration process, saving time and effort. It gives you the flexibility to create users with specific roles, assign custom capabilities, and even integrate user creation into your own custom plugins or themes.
Table of Contents
Why Create Users Programmatically?
There are several reasons why you might want to create users programmatically in WordPress. For example:
- Bulk User Creation: If you need to create multiple users with similar roles and capabilities, doing it manually can be time-consuming. By using a programmatic approach, you can quickly generate a large number of users.
- Custom Registration Process: If you have a custom registration form on your website, you can use the programmatic method to handle user registration behind the scenes. This allows you to add additional checks, and validations, or even integrate with third-party services.
- Integration with Other Systems: When integrating WordPress with other systems or platforms, creating users programmatically can streamline the process. This is particularly useful when synchronizing user data between different applications.
Step-by-Step Process to Create a User in WordPress Programmatically
Now let’s dive into the step-by-step process of creating a user in WordPress programmatically:
- Initialize the WordPress Environment: Before interacting with WordPress functions, make sure to include the necessary WordPress files and initialize the environment using
wp-load.php
.
<?php require_once(ABSPATH . 'wp-load.php');
- Create User Data: Prepare the user data that will be used to create the user. This includes information such as username, email address, password, and any additional user metadata.
$username = 'exampleuser'; $email = 'example@example.com'; $password = wp_generate_password(); $user_data = array( 'user_login' => $username, 'user_email' => $email, 'user_pass' => $password, );
- Check for Existing User: It’s important to check if the user already exists before creating a new one. Use the
username_exists()
oremail_exists()
functions to verify if the username or email is already registered.
if (username_exists($username) || email_exists($email)) { // User already exists, handle accordingly } else { // Proceed with creating the user }
- Generate a Random Password: If you are creating a temporary user or want to generate a random password for security reasons, you can use the
wp_generate_password()
function to create a strong password.
$password = wp_generate_password();
- Create the User: Once all the necessary checks have been performed, create the user using the
wp_insert_user()
function. This function returns the user ID if successful, allowing you to perform further actions if needed.
$user_id = wp_insert_user($user_data); if (!is_wp_error($user_id)) { // User creation successful } else { // User creation failed, handle accordingly }
- Assign Roles and Capabilities: If you want to assign specific roles or capabilities to the user, use the
wp_update_user()
function along with the appropriate parameters. This allows you to define whether the user should be an administrator, editor, author, contributor, or subscriber.
$role = 'author'; // Set the desired role here $user = new WP_User($user_id); $user->set_role($role);
Real Use Cases with Example Codes
To better understand the practical application of creating users programmatically in WordPress, let’s explore some real use cases along with example codes:
Use Case 1: Membership Websites
Membership websites often require automated user creation. By creating users programmatically, you can streamline the registration process for new members, assign appropriate membership roles, and grant access to restricted content.
// Example code for membership website use case // Create a new user with 'subscriber' role $user_data = array( 'user_login' => 'newuser', 'user_email' => 'newuser@example.com', 'user_pass' => wp_generate_password(), ); $user_id = wp_insert_user($user_data); if (!is_wp_error($user_id)) { $user = new WP_User($user_id); $user->set_role('subscriber'); // Additional actions for membership setup } else { // User creation failed, handle accordingly }
Use Case 2: Multisite Networks
In a multisite network, where multiple websites are managed under a single WordPress installation, programmatic user creation can simplify the onboarding process for new site owners. You can automatically generate an administrator account for each new site created.
// Example code for multisite network use case // Create an administrator account for a new site $site_id = get_current_blog_id(); // Get the ID of the current site $new_user_data = array( 'user_login' => 'admin', 'user_email' => 'admin@example.com', 'user_pass' => wp_generate_password(), ); $new_user_id = wp_insert_user($new_user_data); if (!is_wp_error($new_user_id)) { add_user_to_blog($site_id, $new_user_id, 'administrator'); // Additional actions for multisite setup } else { // User creation failed, handle accordingly }
Use Case 3: Custom User Registration Forms
If you have a custom user registration form on your website, programmatic user creation allows you to handle form submissions efficiently. You can validate user inputs, sanitize data, and customize the registration flow according to your specific requirements.
// Example code for custom user registration form use case // Create a new user with custom form data $username = sanitize_text_field($_POST['username']); $email = sanitize_email($_POST['email']); $password = wp_generate_password(); $user_data = array( 'user_login' => $username, 'user_email' => $email, 'user_pass' => $password, ); $user_id = wp_insert_user($user_data); if (!is_wp_error($user_id)) { // Additional actions for custom registration form processing } else { // User creation failed, handle accordingly }
Conclusion
Creating a user in WordPress programmatically provides flexibility and efficiency in managing user registrations. By following the step-by-step guide outlined in this article and exploring real use cases with example codes, you can easily implement this functionality in your WordPress projects. Remember to keep your code organized and secure when implementing programmatic user creation and always test thoroughly before deploying it in a production environment.
Happy programming!