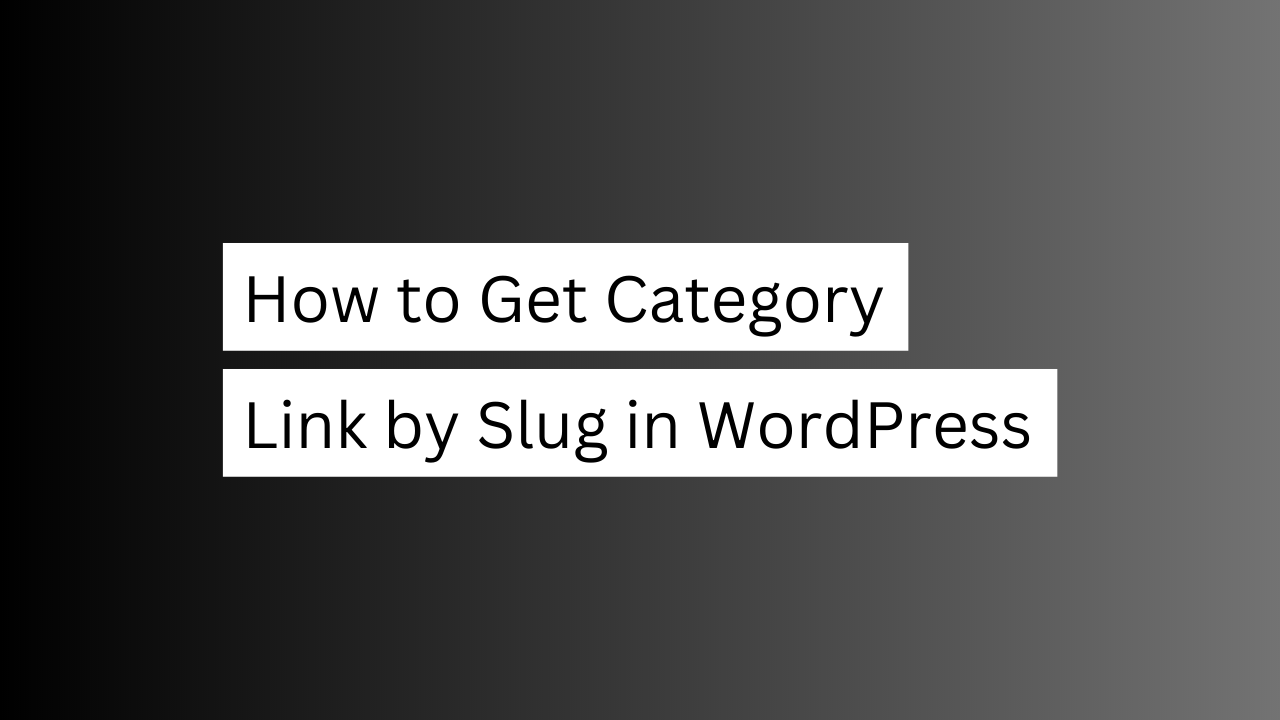
WordPress is a versatile content management system (CMS) that powers millions of websites across the internet. It provides a wide range of features and functionality to help users effectively manage their content. One common task in WordPress is obtaining category links by their slugs. In this article, we will explore different methods to achieve this goal and provide step-by-step instructions to assist you in getting category links by slug in WordPress.
Method 1: Using get_category_link() function:
WordPress offers a built-in function called get_category_link()
that allows you to retrieve the URL of a category based on its slug. This method is straightforward and requires minimal coding knowledge. Follow the steps below to implement this approach:
Step 1: Open the WordPress theme file where you want to display the category link.
Step 2: Identify the category slug you wish to retrieve the link for.
Step 3: Add the following code snippet in the appropriate location within your theme file:
$category = get_category_by_slug('category-slug');
if ($category) {
$category_link = get_category_link($category->term_id);
echo '<a href="' . esc_url($category_link) . '">' . $category->name . '</a>';
}
Replace 'category-slug'
with the actual slug of your desired category. The code snippet retrieves the category object based on the slug and generates the category link using get_category_link()
. Finally, it displays the link using the <a>
HTML tag.
Method 2: Utilizing WP_Term_Query:
Another approach involves using the WP_Term_Query
class to fetch the category link. This method provides more flexibility and can be used to retrieve multiple category links simultaneously. Follow these steps to implement this approach:
Step 1: Open the WordPress theme file where you want to display the category links.
Step 2: Add the following code snippet in the appropriate location within your theme file:
$args = array(
'slug' => 'category-slug',
'taxonomy' => 'category',
);
$categories = new WP_Term_Query($args);
if (!empty($categories->terms)) {
foreach ($categories->terms as $category) {
$category_link = get_category_link($category->term_id);
echo '<a href="' . esc_url($category_link) . '">' . $category->name . '</a>';
}
}
Replace 'category-slug'
with the desired category slug. The code snippet creates a new WP_Term_Query
object with the specified arguments, including the slug and the taxonomy (in this case, ‘category’). It then loops through the retrieved categories, generates their links, and displays them.
Conclusion
Obtaining category links by slug in WordPress is a common requirement when customizing themes or developing plugins. By utilizing the get_category_link()
function or the WP_Term_Query
class, you can easily retrieve category links based on their slugs. Whether you prefer a concise solution or need more flexibility, the methods presented in this article should provide you with the necessary tools to accomplish this task efficiently.