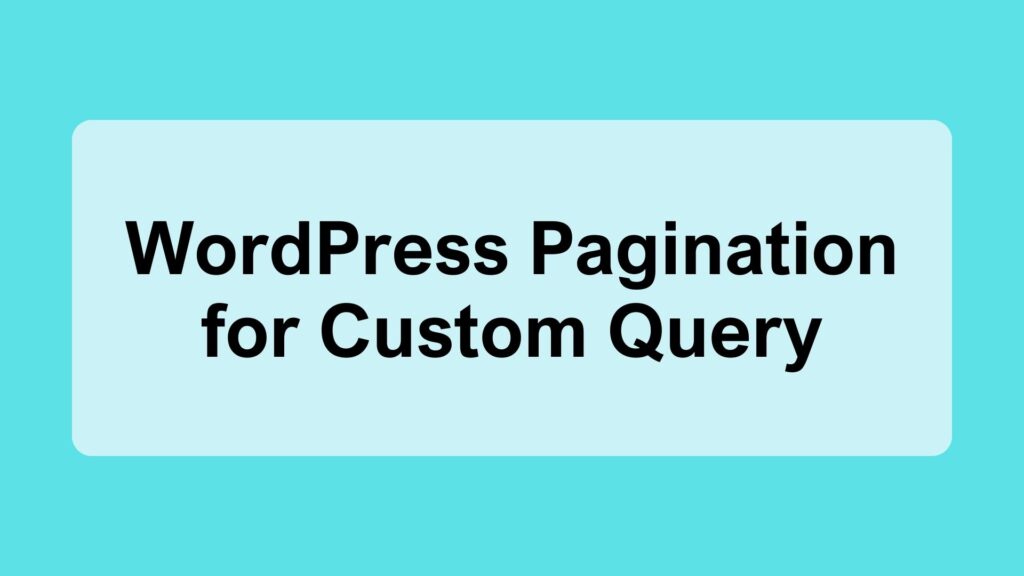
Pagination is a crucial feature when it comes to displaying a list of posts on your WordPress website. It ensures that long lists of content are broken down into manageable chunks, making it easier for your visitors to navigate and find what they’re looking for. While WordPress provides built-in pagination for standard post archives, you might need custom pagination for unique situations. In this article, we will explore how to implement WordPress pagination for a custom query.
Table of Contents
WordPress Pagination for Custom Query: Full Code Snippet
Before diving into the details, let’s take a look at the complete code snippet that enables custom pagination for a specific query:
global $paged; if ( get_query_var( 'paged' ) ) { $paged = get_query_var( 'paged' ); } elseif ( get_query_var( 'page' ) ) { $paged = get_query_var( 'page' ); } else { $paged = 1; } $query = new WP_Query( array( 'post_type' => array( 'post' ), 'posts_per_page' => $postsperpage, 'paged' => $paged, )); if ($query->have_posts()) : while($query->have_posts()) : $query->the_post(); the_title(); the_content(); endwhile; endif; echo paginate_links( array( 'total' => $query->max_num_pages, 'type' => 'list', 'current' => max( 1, $paged ), 'prev_text' => '<i class="fa fa-angle-left"></i>', 'next_text' => '<i class="fa fa-angle-right"></i>' ) );
Now, let’s break down this code snippet section by section to understand how it works.
Deconstructing the Code Snippet: A Section-by-Section Explanation
1. Setting the $paged
Variable
The first section of the code is responsible for determining the current page number and setting it to the $paged
variable. This variable represents the current page within the pagination.
global $paged; if ( get_query_var( 'paged' ) ) { $paged = get_query_var( 'paged' ); } elseif ( get_query_var( 'page' ) ) { $paged = get_query_var( 'page' ); } else { $paged = 1; }
In this section, we check if the ‘paged’ query variable is set and assign its value to the $paged
variable. If ‘paged’ is not set, we check for the ‘page’ query variable and assign its value to $paged
. If neither ‘paged’ nor ‘page’ is set, we default to the first page with $paged = 1
.
2. Custom Query
$query = new WP_Query( array( 'post_type' => array( 'post' ), 'posts_per_page' => $postsperpage, 'paged' => $paged, ));
The posts_per_page
parameter controls how many posts are displayed per page, and the paged
parameter is set to the previously determined $paged
value.
3. Displaying Pagination Links
The final section of the code generates and displays the pagination links using the paginate_links
function. This function takes an array of parameters to customize the pagination display, including the total number of pages, the type of links, and the text for the previous and next buttons.
echo paginate_links( array( 'total' => $query->max_num_pages, 'type' => 'list', 'current' => max( 1, $paged ), 'prev_text' => '<i class="fa fa-angle-left"></i>', 'next_text' => '<i class="fa fa-angle-right"></i>' ) );
total
: The total number of pages, which is retrieved from themax_num_pages
property of theWP_Query
object.type
: Specifies the type of pagination links. In this example, it’s set to ‘list’ for a list of page numbers.current
: The current page, ensuring it’s never less than 1.prev_text
: The HTML for the “previous” button, with a left arrow icon.next_text
: The HTML for the “next” button, with a right arrow icon.
Learn More About paginate_links
function.
By following this code structure and explanation, you can easily implement custom pagination for your WordPress custom queries, providing a more tailored and user-friendly experience for your website visitors.
Conclusion
Custom pagination in WordPress allows you to take control of how your content is presented to your audience. By using the code snippet provided in this article, you can create a customized pagination system for your custom queries, giving your website a more professional and user-friendly appearance. This code empowers you to divide and display your content in a structured and organized manner, enhancing the overall user experience and making it easier for visitors to find the information they seek. So, the next time you need to implement custom pagination in your WordPress project, you have the knowledge and code snippet to get you started.