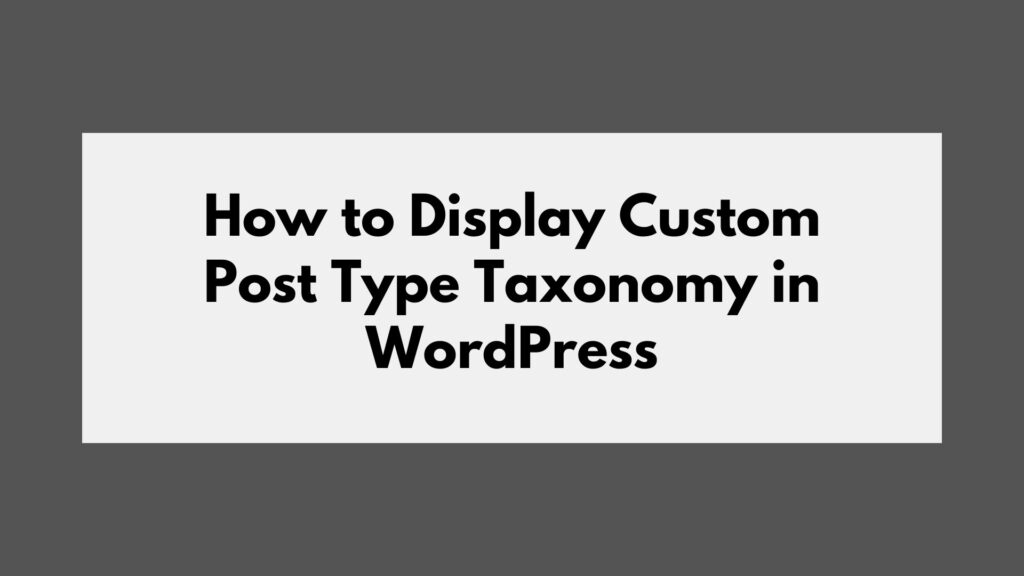
In this article, we will explore the steps involved in displaying custom post type taxonomy in WordPress. We will provide a comprehensive guide with examples to help you understand and implement this feature effectively.
Are you looking to enhance your WordPress website by creating custom post types and taxonomies? Custom post types and taxonomies allow you to organize and display content in a more structured manner. In this article, we will focus on the process of displaying custom post type taxonomy in WordPress.
Table of Contents
Understanding Custom Post Types and Taxonomies
Before we delve into the details, let’s briefly understand what custom post types and taxonomies are in the context of WordPress.
Custom Post Types
WordPress provides default post types such as “Posts” and “Pages” for managing content. However, with custom post types, you can create your own content types with custom fields and settings. This allows you to have more control over the organization and presentation of your website’s content.
Taxonomies
Taxonomies are used to categorize and group content within custom post types. They allow you to create different classifications or tags for your content. By organizing your content using taxonomies, you can provide a better user experience and make it easier for visitors to find relevant information on your website.
Displaying Custom Post Type Taxonomy in WordPress
Now that we have a basic understanding of custom post types and taxonomies, let’s dive into the process of displaying custom post type taxonomy in WordPress.
Step 1: Register the Custom Post Type
To display custom post type taxonomy in WordPress, the first step is to register the custom post type. This involves defining the labels, settings, and capabilities for the new content type.
To register a custom post type, we can use the register_post_type()
function provided by WordPress. This function takes two parameters: the name of the custom post type and an array of arguments defining its properties.
Let’s take a closer look at an example of registering a custom post type:
// Registering a custom post type function create_custom_post_type() { $args = array( 'labels' => array( 'name' => 'Custom Post Type', 'singular_name' => 'Custom Post', ), // Add other settings and capabilities here ); register_post_type('custom_post', $args); } add_action('init', 'create_custom_post_type');
In this example, we define a function called create_custom_post_type()
which will be hooked to the init
action. Inside this function, we set up an array of arguments ($args
) that specify the labels for our custom post type. The name
parameter sets the plural name for the custom post type, while singular_name
defines the singular name.
You can customize other settings and capabilities for your custom post type by adding them to the $args
array. Some common settings include 'public'
(to make the post type publicly accessible), 'supports'
(to enable specific features like title, editor, etc.), and 'rewrite'
(to define the URL structure).
Once you have defined all the necessary settings, you can call the register_post_type()
function with the name of your custom post type (‘custom_post’ in this example) and the $args
array. This will register your custom post type in WordPress.
Step 2: Create the Taxonomy
After registering the custom post type, the next step is to create the taxonomy. Taxonomies allow you to categorize and group content within your custom post type, providing a way to organize and classify your data effectively.
To create a taxonomy, we can use the register_taxonomy()
function provided by WordPress. This function takes three parameters: the name of the taxonomy, the name of the custom post type it will be associated with, and an array of arguments defining its properties.
Let’s take a closer look at an example of creating a taxonomy:
// Creating a taxonomy function create_custom_taxonomy() { $args = array( 'labels' => array( 'name' => 'Custom Taxonomy', 'singular_name' => 'Custom Category', ), // Add other settings and capabilities here ); register_taxonomy('custom_taxonomy', 'custom_post', $args); } add_action('init', 'create_custom_taxonomy');
In this example, we define a function called create_custom_taxonomy()
which will be hooked to the init
action. Inside this function, we set up an array of arguments ($args
) that specify the labels for our taxonomy. The name
parameter sets the plural name for the taxonomy while singular_name
defining the singular name.
Just like when registering a custom post type, you can customize other settings and capabilities for your taxonomy by adding them to the $args
array. Some common settings include 'hierarchical'
(to enable parent-child relationships within the taxonomy), 'public'
(to make the taxonomy publicly accessible), and 'rewrite'
(to define the URL structure).
Once you have defined all the necessary settings, you can call the register_taxonomy()
function with the name of your taxonomy (‘custom_taxonomy’ in this example), the name of the custom post type it will be associated with (‘custom_post’ in this example), and the $args
array. This will create the taxonomy in WordPress and associate it with your custom post type.
Step 3: Assign Taxonomy to Custom Post Type
Now that we have both the custom post type and taxonomy created, we need to associate them together. This can be achieved using the register_taxonomy_for_object_type()
function. Here’s an example:
// Associating taxonomy with custom post type function assign_taxonomy_to_post_type() { register_taxonomy_for_object_type('custom_taxonomy', 'custom_post'); } add_action('init', 'assign_taxonomy_to_post_type');
Step 4: Displaying the Taxonomy Terms
After creating the taxonomy and associating it with your custom post type, the next step is to display the taxonomy terms on your WordPress website. This allows users to easily navigate and filter content based on different categories or tags.
To display the taxonomy terms, we can utilize various WordPress functions and template tags to retrieve and output the desired information. Let’s consider an example where we want to display all the terms of our custom taxonomy:
// Displaying taxonomy terms $terms = get_terms('custom_taxonomy'); foreach ($terms as $term) { echo '<a href="' . get_term_link($term) . '">' . $term->name . '</a>'; }
In this example, we use the get_terms()
function to retrieve all the terms of our custom taxonomy (‘custom_taxonomy’). The function returns an array of term objects, which we store in the $terms
variable.
We then iterate through each term using a foreach loop and access its properties using the $term
variable. In this case, we output a link to each term’s archive page using the get_term_link()
function, along with the term’s name.
You can customize the output according to your specific requirements. For example, you might want to display additional information about each term, such as its description or count of associated posts.
By implementing this code snippet in your WordPress template files, you will be able to display the taxonomy terms associated with your custom post type on your website.
Remember to style the output using CSS to ensure it matches your website’s design and layout.
Congratulations! You have successfully completed all the steps required to display custom post type taxonomy in WordPress. By following these guidelines, you can organize and present your content in a structured manner, allowing users to easily navigate and explore your website.
Conclusion
In this article, we explored the process of displaying custom post type taxonomy in WordPress. We learned about custom post types, taxonomies, and how they can be used to organize and present content efficiently. By following the steps outlined above, you can successfully create and display custom post type taxonomy on your WordPress website.
Remember, implementing custom post types and taxonomies allows you to create a more structured and user-friendly website experience. So go ahead and start experimenting with these powerful features in WordPress!