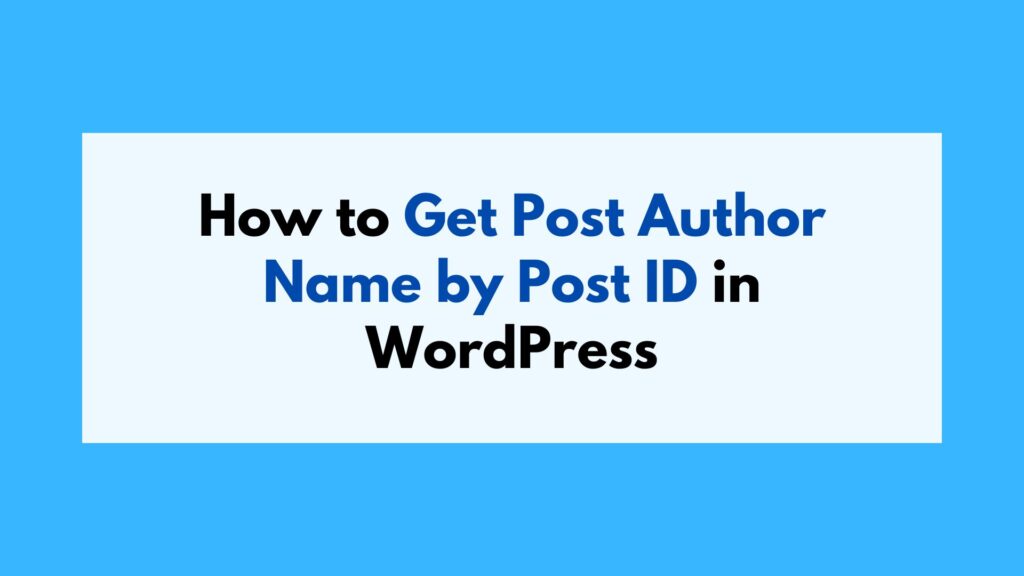
Have you ever wondered how to retrieve the author’s name of a WordPress post by its post ID? Whether you’re a developer or a WordPress enthusiast, there may come a time when you need to programmatically access and display the author’s name for a specific post. In this article, we will explore different methods to achieve this task effectively. So let’s dive in!
To get the post author’s name by post ID in WordPress, you can use the get_the_author_meta()
function along with the display_name
parameter. Alternatively, you can utilize the get_userdata()
function to retrieve the author’s information and then extract the desired name fields such as first name, last name, full name, nickname, or username.
Table of Contents
How to Get Post Author Name by Post ID:
Method 1: Using get_the_author_meta()
Function:
One straightforward method to obtain the post author’s name is by utilizing the get_the_author_meta()
function. This function retrieves the specified metadata of an author based on their user ID. To get the author’s name, we can pass the display_name
parameter to this function.
$post_id = 123; // Replace with your desired post ID $author_name = get_the_author_meta('display_name', get_post_field('post_author', $post_id)); echo "Author Name: " . $author_name;
Method 2: Using get_userdata()
Function:
Another approach to retrieve the author’s name is by using the get_userdata()
function. This function returns a WP_User object that contains all user data associated with a given user ID. We can then extract specific name fields from this object.
$post_id = 123; // Replace with your desired post ID $user_info = get_userdata(get_post_field('post_author', $post_id)); $first_name = $user_info->first_name; $last_name = $user_info->last_name; $full_name = $user_info->first_name . ' ' . $user_info->last_name; $nickname = $user_info->nickname; $username = $user_info->user_login; echo "First Name: " . $first_name; echo "Last Name: " . $last_name; echo "Full Name: " . $full_name; echo "Nickname: " . $nickname; echo "Username: " . $username;
Examples:
Let’s explore a few examples to better understand how these methods work.
Example 1: Retrieving the Author’s Name for a Single Post:
Suppose we have a post with the ID of 123, and we want to display the author’s full name in our template file. We can use Method 1 as follows:
$post_id = 123; $author_name = get_the_author_meta('display_name', get_post_field('post_author', $post_id)); echo "Author Name: " . $author_name;
Example 2: Displaying Multiple Name Fields for an Author:
In some cases, you may need to display multiple name fields for an author, such as first name, last name, and username. Method 2 allows us to extract these details easily:
$post_id = 123; $user_info = get_userdata(get_post_field('post_author', $post_id)); $first_name = $user_info->first_name; $last_name = $user_info->last_name; $username = $user_info->user_login; echo "First Name: " . $first_name; echo "Last Name: " . $last_name; echo "Username: " . $username;
Conclusion:
Retrieving the author’s name by post ID in WordPress can be achieved using various methods. In this article, we covered two effective approaches using the get_the_author_meta()
and get_userdata()
functions. By following the provided examples and instructions, you can now easily display different name fields for a post author in your WordPress projects.
Remember to replace the post ID with your own desired ID when implementing these methods in your code. Happy coding!