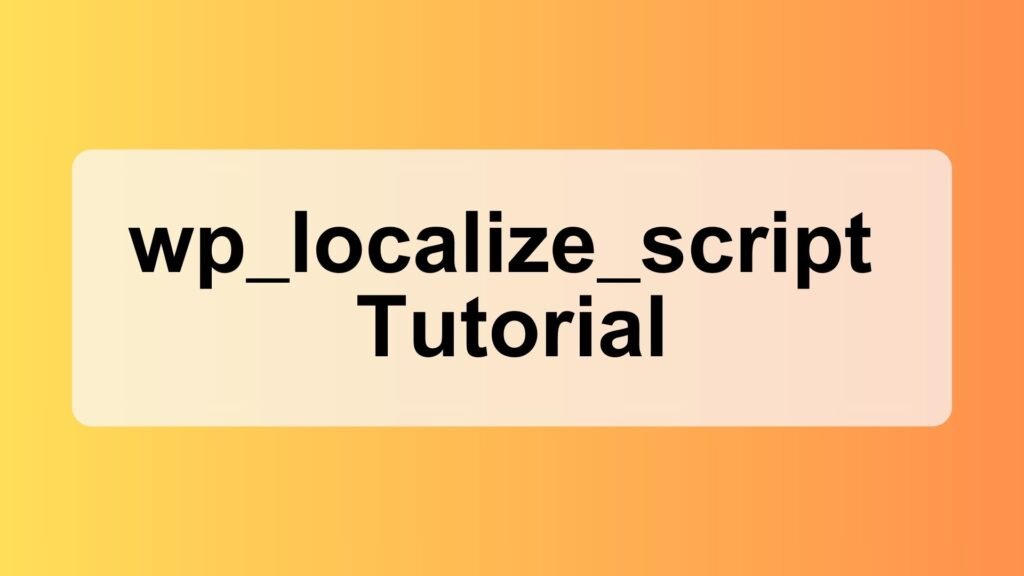
If you’re a WordPress developer or have ever delved into the world of WordPress theme or plugin development, you’ve probably come across the term “wp_localize_script.” This function plays a crucial role in WordPress development, enabling developers to pass data from the server-side to the client-side JavaScript. In this article, we’ll explore what wp_localize_script is, what it does, and how to use it effectively.
Table of Contents
What is wp_localize_script?
wp_localize_script
is a powerful and essential function in WordPress that allows developers to pass localized data from the server side (PHP) to the client side (JavaScript). It is used primarily for enqueuing and localizing scripts in your WordPress theme or plugin.
In simpler terms, wp_localize_script helps bridge the gap between your PHP code and JavaScript, enabling you to pass dynamic data, such as configuration settings, nonces, or even content, from your WordPress backend to the frontend scripts. This is particularly useful for creating interactive and dynamic web applications within the WordPress ecosystem.
What does wp_localize_script do?
The primary purpose of wp_localize_script is to make it easy to send data from the server to your JavaScript files while maintaining the separation of concerns between PHP and JavaScript. Here’s what wp_localize_script does:
- Data Localization: It takes an array of data in PHP and makes it accessible as a JavaScript object. This allows you to use PHP-generated variables in your JavaScript files, providing dynamic and contextual content to your scripts.
- Data Security: By localizing data using this function, you can ensure that the data is properly sanitized and escaped, reducing the risk of security vulnerabilities. WordPress takes care of these security measures for you.
- Dependency Management: wp_localize_script also takes care of script dependency management. It ensures that your localized data is available when the target script is enqueued, avoiding potential timing issues.
How to use wp_localize_script
To use wp_localize_script effectively in your WordPress development projects, follow these steps:
Step 1) Enqueue Your Script:
First, you need to enqueue your JavaScript file using the wp_enqueue_script
function. You should do this in your theme’s functions.php file or your plugin’s main file.
wp_enqueue_script('your-script-handle', 'path-to-your-js-file.js', array('jquery'), '1.0', true);
Replace ‘your-script-handle’ with your script’s handle, and ‘path-to-your-js-file.js’ with the actual path to your JavaScript file.
Step 2) Define Your Data Array
Create an array of data that you want to pass from PHP to JavaScript. This could include settings, variables, or any dynamic content you need in your script.
$data = array( 'some_setting' => get_option('your_option_name'), 'another_setting' => 'Some dynamic content', );
Customize the array based on your project’s specific requirements.
Step 3) Localize Your Script:
Now, use the wp_localize_script
function to pass the data to your script.
wp_localize_script('your-script-handle', 'yourLocalizedObject', $data);
'your-script-handle'
should match the handle used when enqueuing the script.'yourLocalizedObject'
is the name of the JavaScript object that will contain your data.$data
is the array of data you want to pass.
Step 4) Access Data in JavaScript:
In your JavaScript file, you can access the localized data using the yourLocalizedObject
object.
alert(yourLocalizedObject.some_setting);
You can now use the data within your JavaScript code, making it dynamic and responsive to server-side changes.
Conclusion
In the world of WordPress development, wp_localize_script
is a valuable tool for seamlessly passing data from the server side to the client side, enhancing the dynamic capabilities of your WordPress themes and plugins. By following the steps outlined in this article, you can effectively use wp_localize_script to create interactive and data-driven web applications that leverage the power of WordPress. This function simplifies the process of managing and localizing data, making your development tasks more efficient and secure.