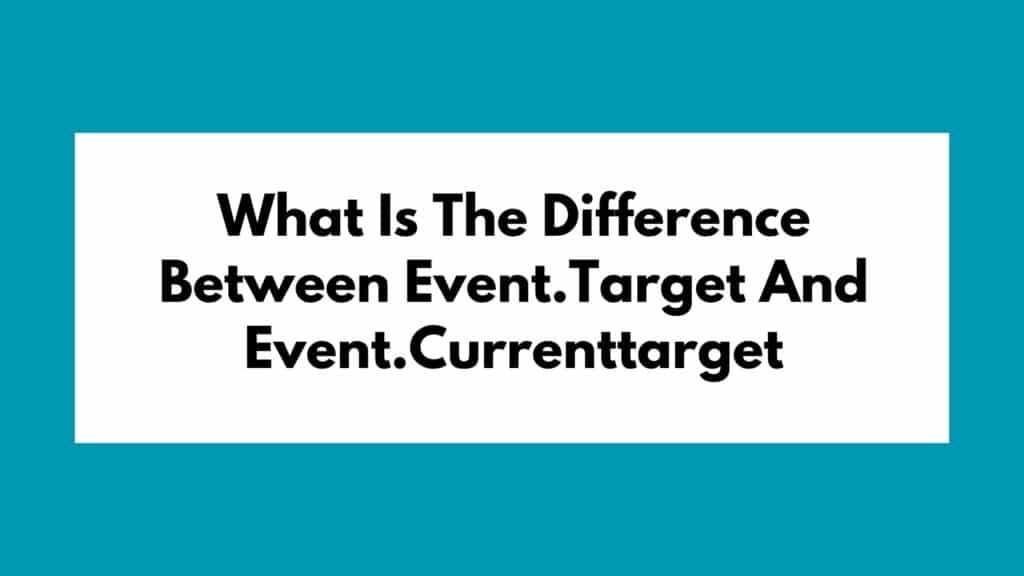
In the world of web development, understanding the nuances between event.target
and event.currentTarget
is essential for building robust and interactive web applications. These two properties are commonly encountered when dealing with events in JavaScript, especially in the context of event delegation. Let’s delve into the differences between these two properties to gain a clearer understanding.
Table of Contents
What is event.target?
The event.target
property refers to the element that triggered the event. It points to the element where the event listener was originally attached or the element that actually dispatched the event. This property remains constant throughout the event propagation and does not change.
Example:
document.querySelector('.parent').addEventListener('click', function(event) { console.log(event.target); });
In this example, if a child element within an element with the class parent
is clicked, event.target
will refer to the clicked child element.
What is event.currentTarget?
On the other hand, event.currentTarget
refers to the element to which the event handler is currently attached. Unlike event.target
, event.currentTarget
can change as the event propagates through nested elements.
Example:
document.querySelector('.parent').addEventListener('click', function(event) { console.log(event.currentTarget); });
In this example, regardless of which child element within the element with the class parent
is clicked, event.currentTarget
will always refer to the element with the class parent
.
Key Differences
event.target
: Refers to the element that triggered the event.event.currentTarget
: Refers to the element to which the event handler is currently attached.
Example Use Case:
Suppose we have a list of items where each item has a click event listener. When an item is clicked, we want to highlight only that specific item. In this scenario, understanding the difference between event.target
and event.currentTarget
becomes crucial.
Example Code Snippet:
document.querySelectorAll('.item').forEach(item => { item.addEventListener('click', function(event) { event.currentTarget.style.backgroundColor = 'yellow'; }); });
In this code snippet, when an item is clicked, only the clicked item will be highlighted with a yellow background, demonstrating how event.currentTarget
can be used to target the specific element where the event listener is attached.
Conclusion
Understanding the distinctions between event.target
and event.currentTarget
is vital for effective event handling in JavaScript. By utilizing these properties appropriately, developers can enhance user interactions and create more dynamic and responsive web applications. Remembering to leverage event.target
for identifying the triggering element and event.currentTarget
for targeting the current event handler attachment can significantly improve your web development workflow. Incorporating these concepts into your projects will help you build more interactive and engaging web experiences for users.