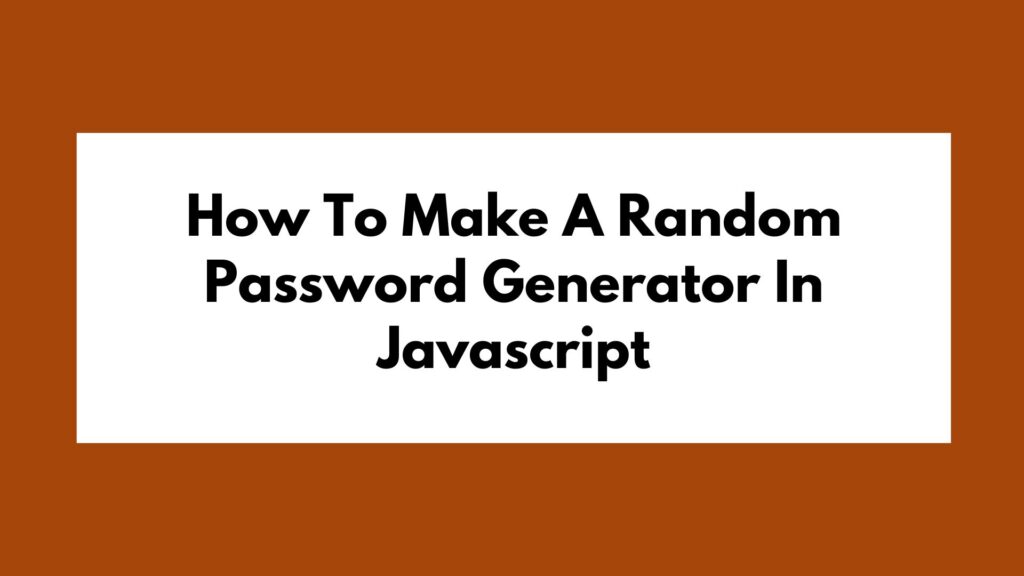
In today’s digital landscape, safeguarding data is paramount, making a secure random password generator in JavaScript a vital tool. This article guides you through the creation of a robust password generator using two innovative methods. Dive into code snippets and detailed explanations to elevate your password complexity and variability, ensuring enhanced security. Join us on this journey to craft strong and diverse passwords that fortify your online defenses.
Follow These Two Methods To Make A Random Password Generator In Javascript
Method 1: Generating a Random Password String
To generate a random password string in JavaScript, we utilized the Math.random()
function combined with basic string manipulation. The Math.random()
function generates a random floating-point number between 0 (inclusive) and 1 (exclusive). By multiplying this value with the length of our character set and using Math.floor()
, we can obtain random indices to select characters from the set.
function generateRandomPassword(length) { const charset = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789!@#$%^&*()_+~`|}{[]\:;?><,./-='; let password = ''; for (let i = 0; i < length; i++) { const randomIndex = Math.floor(Math.random() * charset.length); password += charset[randomIndex]; } return password; }
Code Snippet Explanation:
- We define a function
generateRandomPassword
that takes alength
parameter. - A character set
charset
is created including uppercase letters, lowercase letters, numbers, and special characters. - A loop generates random indices to select characters from the charset and constructs the password string.
- The function returns the generated random password.
Method 2: Adding Complexity with Entropy
In the second method for generating complex passwords, we leveraged the Math.random()
function alongside conditional statements to select characters from different character sets based on a randomly generated index. This approach allows us to introduce entropy and complexity into the generated passwords, enhancing their security.
function generateComplexPassword(length) { const lowerCaseChars = 'abcdefghijklmnopqrstuvwxyz'; const upperCaseChars = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ'; const numbers = '0123456789'; const specialChars = '!@#$%^&*()'; const allChars = lowerCaseChars + upperCaseChars + numbers + specialChars; let password = ''; for (let i = 0; i < length; i++) { const randomSetIndex = Math.floor(Math.random() * 4); const charSet = randomSetIndex === 0 ? lowerCaseChars : randomSetIndex === 1 ? upperCaseChars : randomSetIndex === 2 ? numbers : specialChars; const randomCharIndex = Math.floor(Math.random() * charSet.length); password += charSet[randomCharIndex]; } return password; }
Code Snippet Explanation:
- We define a function
generateComplexPassword
that takes alength
parameter. - Separate character sets for lowercase letters, uppercase letters, numbers, and special characters are defined.
- Random sets are chosen based on an index to add complexity to the generated password.
- The function returns the generated complex password.
Conclusion
By considering these methods in text generation, we can create secure and diverse passwords that are less susceptible to attacks. Implementing these approaches will help you develop a robust random password generator in JavaScript that prioritizes both complexity and variability.