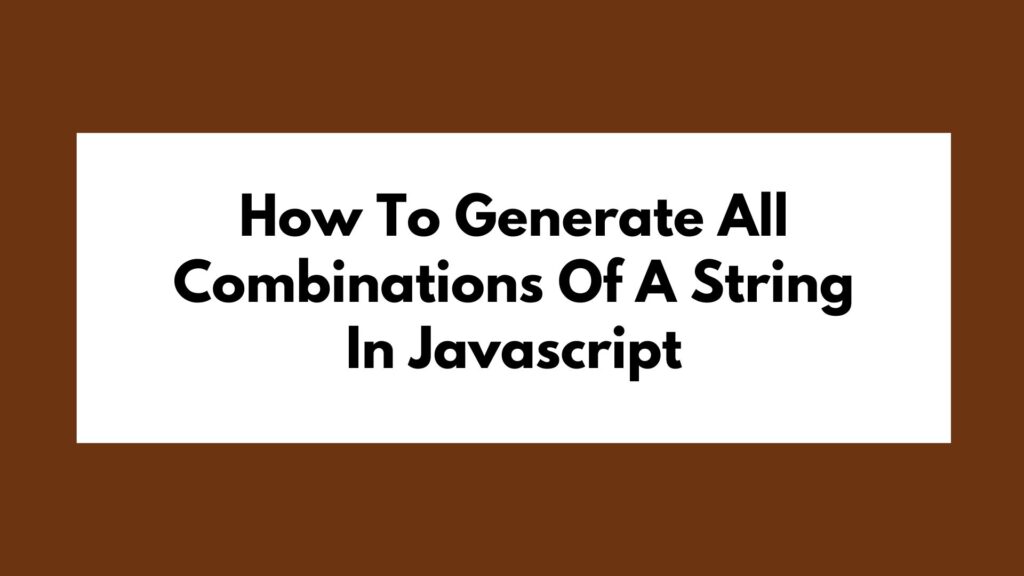
In the realm of programming, the ability to generate all combinations of a string is a powerful and essential skill. Whether you are tackling coding challenges or developing complex algorithms, understanding how to create these combinations efficiently can greatly enhance your problem-solving capabilities. This article will explore two methods in JavaScript for generating all possible combinations of a given string. By following these methods, you can master the art of manipulating strings and unlock new possibilities in your coding journey.
Table of Contents
Understanding the Basics
Before we delve into the methods for generating all combinations of a string in JavaScript, let’s first establish a solid foundation by defining what exactly we mean by “combinations.” In this context, combinations refer to all possible arrangements of the characters within a given string. For example, the combinations of the string “abc” would include “a,” “b,” “c,” “ab,” “ac,” “bc,” and “abc.”
Method 1: Using Recursion
One of the most common approaches to generating all combinations of a string is through recursion. Recursion is a programming technique where a function calls itself within its definition. Here’s a step-by-step breakdown of how you can implement this method in JavaScript:
const getAllCombinations = (str) => { const results = []; const generateCombinations = (current, index) => { if (index === str.length) { results.push(current); return; } generateCombinations(current + str[index], index + 1); generateCombinations(current, index + 1); }; generateCombinations("", 0); return results; }; const inputString = "abc"; const allCombinations = getAllCombinations(inputString); console.log(allCombinations);
In this code snippet, we define a function getAllCombinations
that takes a string str
as input and returns an array of all combinations of the characters in the input string. We initialize an empty array results
to store the generated combinations. Inside the function, we define another function generateCombinations
, which uses recursion to generate the combinations. It takes two parameters: current
(the current combination being built) and index
(the current index of the character in the input string).
The generateCombinations
function checks if the index is equal to the length of the input string. If it is, it means we have generated a complete combination, so we push it to the results
array. Otherwise, the function recursively calls itself twice: once by adding the next character to the current combination and once without adding the character. This process continues until all possible combinations are generated. Finally, we call generateCombinations
with an empty string and index 0 to start the recursion process and return the results
array containing all combinations.
Method 2: Using Iteration
Another approach to generating all combinations of a string is through iteration. Instead of relying on recursive calls, this method involves using loops to systematically generate the combinations. Here’s how you can achieve this in JavaScript:
const getAllCombinationsIterative = (str) => { const results = []; for (let i = 0; i < Math.pow(2, str.length); i++) { let combination = ""; for (let j = 0; j < str.length; j++) { if ((i & (1 << j)) > 0) { combination += str[j]; } } results.push(combination); } return results; }; const inputString = "abc"; const allCombinationsIterative = getAllCombinationsIterative(inputString); console.log(allCombinationsIterative);
In this code snippet, we define a function getAllCombinationsIterative
that takes a string str
as input and returns an array of all combinations of the characters in the input string using an iterative approach. We initialize an empty array results
to store the generated combinations.
We then iterate through all possible combinations using a nested loop. The outer loop runs from 0 to 2 raised to the power of the length of the input string. Within this loop, we construct each combination by checking the bits set in the binary representation of the loop counter i
. For each character position in the input string, if the corresponding bit is set in i
, we append that character to the current combination.
After constructing each combination, we push it to the results
array. Once all combinations are generated, we return the results
array containing all possible combinations of the input string “abc”. Finally, we log the generated combinations to the console for display.
Conclusion
In conclusion, generating all combinations of a string in JavaScript can be achieved through various methods, each with its own advantages and considerations. By leveraging the power of recursion or iteration, you can efficiently create these combinations and apply them to your coding projects effectively.
By mastering these techniques and understanding the intricacies of string manipulation in JavaScript, you can enhance your programming skills and tackle complex challenges with confidence. So go ahead, experiment with these methods, and unlock the full potential of generating string combinations in your code!