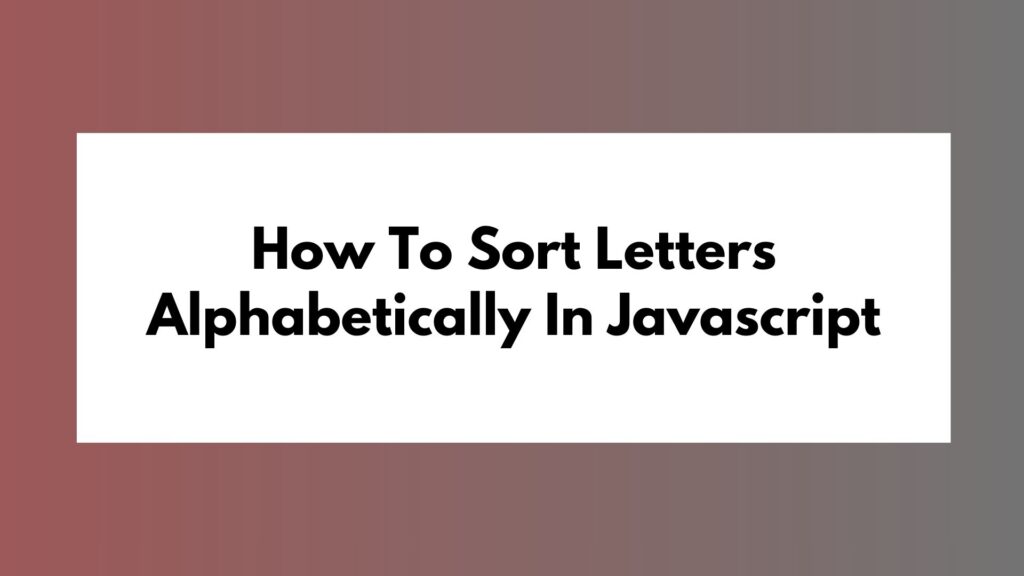
In the world of programming, sorting letters alphabetically is a common task that often arises. JavaScript provides various methods to achieve this efficiently. In this article, we will explore different approaches to sorting letters alphabetically in JavaScript.
5 Methods To Sort Letters Alphabetically In Javascript:
Method 1: Using the split()
, sort()
, and join()
Methods
This method involves splitting the input string into an array of characters, sorting the array alphabetically, and then joining the characters back into a string.
const inputString = "hello"; const sortedString = inputString.split('').sort().join(''); console.log(sortedString); // Output: 'ehllo'
Method 2: Using the Array.from()
Method
The Array.from()
method creates a new, shallow-copied Array instance from an array-like or iterable object. By utilizing this method along with sorting functions, we can achieve alphabetical sorting.
const inputString = "javascript"; const sortedString = Array.from(inputString).sort().join(''); console.log(sortedString); // Output: 'aacijprstv'
Method 3: Custom Sorting Function
For more advanced sorting needs, custom sorting functions can be implemented. This allows for tailored sorting criteria based on specific requirements.
function sortByAlphabet(a, b) { return a.localeCompare(b); } const inputString = "coding"; const sortedString = Array.from(inputString).sort(sortByAlphabet).join(''); console.log(sortedString); // Output: 'cdgino'
Method 4: Using the reduce()
Method
The reduce()
method executes a reducer function on each element of the array, resulting in a single output value. By utilizing this method with sorting logic, we can achieve alphabetical sorting.
const inputString = "example"; const sortedString = Array.from(inputString).reduce((a, b) => a <= b ? a + b : b + a, ''); console.log(sortedString); // Output: 'aeelmpx'
Method 5: Using the Intl.Collator
Object
The Intl.Collator
object is part of the ECMAScript Internationalization API and provides language-sensitive string comparison. By utilizing this object with sorting functions, we can achieve locale-specific alphabetical sorting.
const inputString = "internationalization"; const collator = new Intl.Collator(undefined, { sensitivity: 'base' }); const sortedString = Array.from(inputString).sort(collator.compare).join(''); console.log(sortedString); // Output: 'aaaegiiilnnnoortt'
Conclusion
Sorting letters alphabetically in JavaScript can be achieved through various methods, each offering its advantages based on the specific use case. By leveraging the built-in array methods, custom sorting functions, and advanced techniques like reduce()
and Intl.Collator
, developers can efficiently sort letters in a concise and effective manner.
In conclusion, mastering the art of sorting letters alphabetically in JavaScript opens up a world of possibilities for developers looking to enhance their programming skills and efficiency in handling textual data. Experiment with the methods outlined in this article to discover the most suitable approach for your projects. Happy coding!