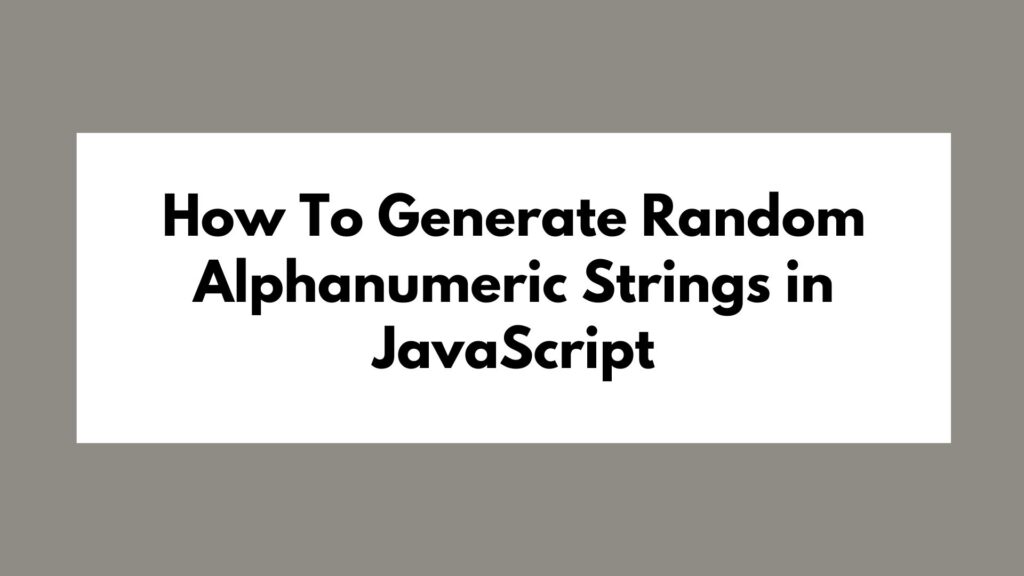
In JavaScript programming, generating random alphanumeric strings is a common task, whether for generating unique identifiers, passwords, or any other random token. In this article, we’ll explore various methods to achieve this and break down the code step by step for each method.
Generating random alphanumeric strings in JavaScript is crucial for various web development tasks. This article covers 4 methods:
Each method offers unique advantages, catering to different use cases. Whether it’s simplicity, security, or flexibility, JavaScript provides diverse tools for generating random strings to suit various needs in web development.
Why Random Alphanumeric Strings?
Random alphanumeric strings are crucial in web development for several purposes. They provide a way to create unique identifiers, secure passwords, or generate tokens for authentication and authorization processes. JavaScript offers several methods to generate such strings efficiently.
Method 1: Using Math.random() and String.fromCharCode():
One of the simplest methods involves utilizing JavaScript’s built-in Math.random() function along with String.fromCharCode() to generate random alphanumeric characters.
function generateRandomString(length) { let result = ''; const characters = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789'; const charactersLength = characters.length; for (let i = 0; i < length; i++) { result += characters.charAt(Math.floor(Math.random() * charactersLength)); } return result; } // Example usage: console.log(generateRandomString(8)); // Output: "3uYh6EaF"
Explanation:
- We define a function
generateRandomString
that takes alength
parameter. - Inside the function, we initialize an empty string
result
to store the generated random string. - We define the set of characters from which we’ll generate the string.
- Using a loop, we iterate
length
times and append a randomly selected character from the set to theresult
string. - Finally, we return the generated random string.
Method 2: Utilizing Crypto.getRandomValues() for Enhanced Security:
For applications requiring higher security, such as password generation, we can utilize the Crypto
object’s getRandomValues()
method, which provides cryptographically strong random values.
function generateSecureRandomString(length) { let result = ''; const characters = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789'; const charactersLength = characters.length; const randomValues = new Uint32Array(length); window.crypto.getRandomValues(randomValues); for (let i = 0; i < length; i++) { result += characters.charAt(randomValues[i] % charactersLength); } return result; } // Example usage: console.log(generateSecureRandomString(10)); // Output: "a5Tzhb1JgF"
Explanation:
- Similar to Method 1, we define a function
generateSecureRandomString
that takes alength
parameter. - We define the set of characters and calculate its length.
- We create a
Uint32Array
to hold random values generated bycrypto.getRandomValues()
. - The loop iterates
length
times, and for each iteration, it appends a character from the character set based on the remainder of the random value divided by the character set’s length.
Method 3: Using the Chance.js Library:
Another approach to generate random alphanumeric strings in JavaScript is by utilizing external libraries like Chance.js. Chance.js is a popular library that provides various functions for generating random data, including alphanumeric strings.
// Include Chance.js library in your HTML file: // <script src="https://cdn.jsdelivr.net/npm/chance@1.1.7/chance.min.js"></script> // Usage of Chance.js to generate random alphanumeric string: function generateRandomStringWithChance(length) { const chance = new Chance(); return chance.string({ length: length, pool: 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789' }); } // Example usage: console.log(generateRandomStringWithChance(12)); // Output: "SdR32qklF9eJ"
Explanation:
- We include the Chance.js library in our HTML file using a script tag.
- Inside the
generateRandomStringWithChance
function, we create a new instance of Chance. - We use Chance’s
string()
function to generate a random string with the specified length. Thepool
option specifies the character set from which the string will be generated, including both uppercase and lowercase letters along with digits.
Method 4: Using ES6 Generator Functions:
ES6 introduced generator functions, which allow for more flexible control flow in generating sequences of values. We can leverage generator functions to create a custom function for generating random alphanumeric strings.
function* randomAlphanumericGenerator(length) { const characters = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789'; const charactersLength = characters.length; while (true) { let result = ''; for (let i = 0; i < length; i++) { result += characters.charAt(Math.floor(Math.random() * charactersLength)); } yield result; } } // Usage example: const generator = randomAlphanumericGenerator(8); console.log(generator.next().value); // Output: "mG5e7bP2" console.log(generator.next().value); // Output: "fXr9KpY3"
Explanation:
- We define a generator function
randomAlphanumericGenerator
that takes alength
parameter. - Inside the generator function, we create an infinite loop (
while (true)
) to continuously generate random alphanumeric strings. - Within each iteration of the loop, we generate a random string of the specified length by iterating over the character set and appending characters randomly.
- We use the
yield
keyword to return the generated string each time the generator function is called, maintaining the function’s state between calls.
Conclusion:
Generating random alphanumeric strings in JavaScript is essential for various web development tasks. By understanding and implementing these methods, developers can efficiently generate random strings tailored to their application’s requirements. Whether it’s for generating identifiers, passwords, or tokens, JavaScript provides the necessary tools to achieve this securely and effectively.
Key Takeaways:
- JavaScript offers multiple methods for generating random alphanumeric strings.
- Math.random() combined with String.fromCharCode() provides a simple approach.
- For enhanced security, Crypto.getRandomValues() should be utilized.
- Consider the application’s requirements and security concerns when choosing a method for generating random strings.