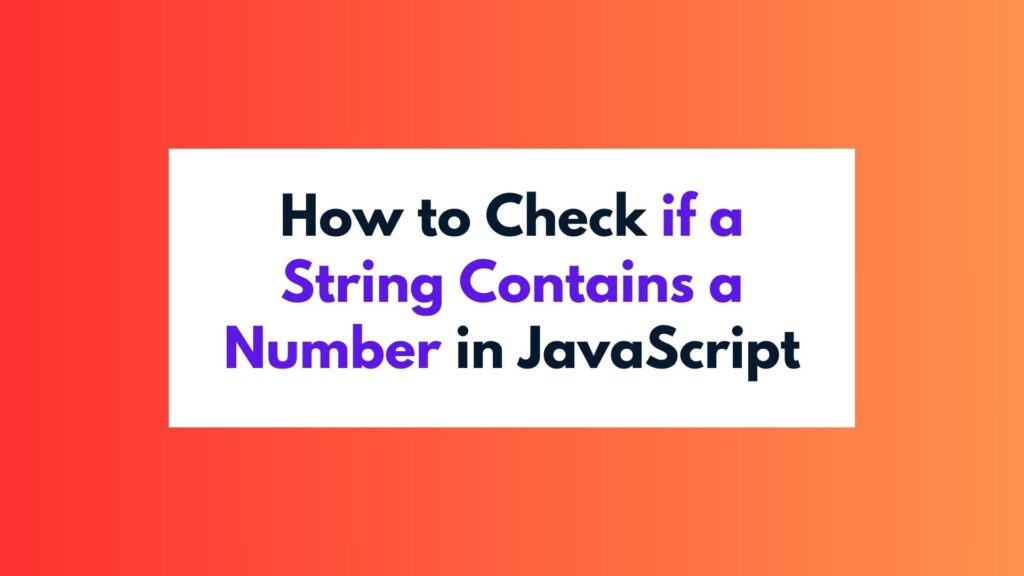
TL;DR: To check if a string contains a number in JavaScript, you can use regular expressions by using the /\d/
pattern or the test()
method. Alternatively, you can use the isNaN()
function in combination with parseFloat()
or parseInt()
. Lastly, you can utilize the match()
method with the /\d+/
pattern. Choose the method that best suits your needs and requirements.
When working with JavaScript, it’s common to come across situations where you need to check if a string contains a number. Fortunately, JavaScript provides several approaches to achieve this. In this article, we will explore different methods to check if a string contains a number in JavaScript, and discuss their pros and cons.
Method 1: Using Regular Expressions
Regular expressions are a powerful tool for pattern matching in JavaScript. You can utilize regular expressions to check if a string contains a number. The following code snippet demonstrates how to use regular expressions to achieve this:
function containsNumber(str) { // Check if the string contains any digit between 0 and 9 return /\d/.test(str); } // Example usage console.log(containsNumber("Hello World")); // Output: false console.log(containsNumber("Hello123")); // Output: true
In this example, the /\d/
regular expression pattern matches any digit from 0 to 9. The test()
method checks if the given string str
contains any digit and returns true
if it does.
Method 2: Using the isNaN() Function
Another approach to check if a string contains a number is by using the isNaN()
function. This function determines whether a value is NaN (Not-a-Number). By converting the string to a number using the parseFloat()
or parseInt()
functions, we can check if the resulting value is NaN or not.
Here’s an example that demonstrates this method:
function containsNumber(str) { return !isNaN(parseFloat(str)) && isFinite(str); } // Example usage console.log(containsNumber("Hello World")); // Output: false console.log(containsNumber("Hello123")); // Output: true
In this example, parseFloat()
converts the string to a floating-point number, while parseInt()
converts it to an integer. The isNaN()
function checks if the resulting value is NaN, and isFinite()
checks if it is a finite number.
Method 3: Using the match() Method
JavaScript strings have a built-in match()
method that allows you to search for a pattern within a string. By using regular expressions, you can check if a string contains a number by utilizing the match()
method as shown in the following example:
function containsNumber(str) { return str.match(/\d+/) !== null; } // Example usage console.log(containsNumber("Hello World")); // Output: false console.log(containsNumber("Hello123")); // Output: true
In this example, the /\d+/
regular expression pattern matches one or more consecutive digits. If the match()
method returns a non-null value, it indicates that the string contains a number.
Conclusion
In this article, we explored three different methods to check if a string contains a number in JavaScript. Regular expressions provide a powerful and flexible way to perform pattern matching, while the isNaN()
function and the match()
method offers alternative approaches. Choose the method that best suits your specific requirements and use case.