When working with strings in JavaScript, it’s common to need a way to check whether a string contains only letters and numbers. In this article, we will explore different methods to achieve this task. Let’s dive into it!
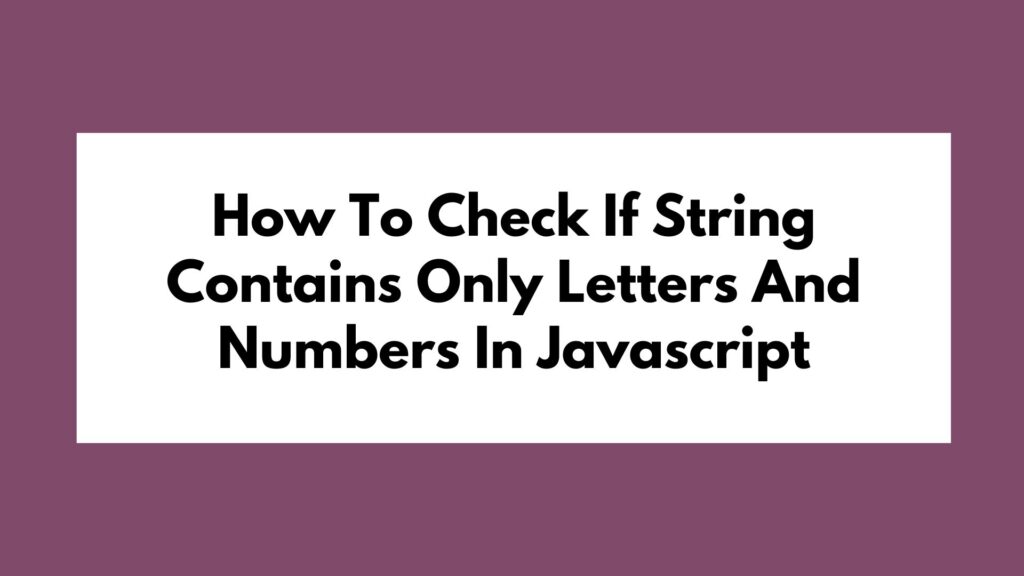
Methods Check If String Contains Only Letters And Numbers In Javascript
Method 1: Using Regular Expressions
One of the most straightforward ways to check if a string contains only letters and numbers is by using regular expressions. Regular expressions provide a concise and powerful way to match patterns in strings.
function isAlphaNumeric(input) { return /^[a-zA-Z0-9]+$/.test(input); } // Test the function console.log(isAlphaNumeric("abc123")); // Output: true console.log(isAlphaNumeric("abc123!")); // Output: false
In the code snippet above, we define a function isAlphaNumeric
that uses a regular expression ^[a-zA-Z0-9]+$
to test if the input string consists of only letters and numbers.
Method 2: Iterating Through Characters
Another approach is to iterate through each character in the string and check if it is either a letter or a number. This method provides more control over the validation process.
function isAlphaNumericIterative(input) { for (let i = 0; i < input.length; i++) { const charCode = input.charCodeAt(i); if (!(charCode >= 48 && charCode <= 57) && !(charCode >= 65 && charCode <= 90) && !(charCode >= 97 && charCode <= 122)) { return false; } } return true; } // Test the function console.log(isAlphaNumericIterative("abc123")); // Output: true console.log(isAlphaNumericIterative("abc123!")); // Output: false
Method 3: Using isNaN
We can also leverage the isNaN
function in JavaScript to check if a string contains only numbers.
function containsOnlyNumbers(input) { return !isNaN(input); } // Test the function console.log(containsOnlyNumbers("123")); // Output: true console.log(containsOnlyNumbers("123abc")); // Output: false
Method 4: Using replace
Another method involves using the replace
method along with a regular expression to remove non-alphanumeric characters from the string and then comparing it with the original string.
function isAlphaNumericReplace(input) { return input === input.replace(/[^a-zA-Z0-9]/g, ''); } // Test the function console.log(isAlphaNumericReplace("abc123")); // Output: true console.log(isAlphaNumericReplace("abc123!")); // Output: false
Conclusion
In this article, we have explored four different methods to check if a string contains only letters and numbers in JavaScript. Each method offers a unique approach, from using regular expressions to leveraging built-in functions like isNaN
and replace
.
By understanding and implementing these methods, you can effectively validate strings in your JavaScript applications and ensure they contain only the desired characters. Experiment with these approaches and choose the one that best fits your specific requirements.