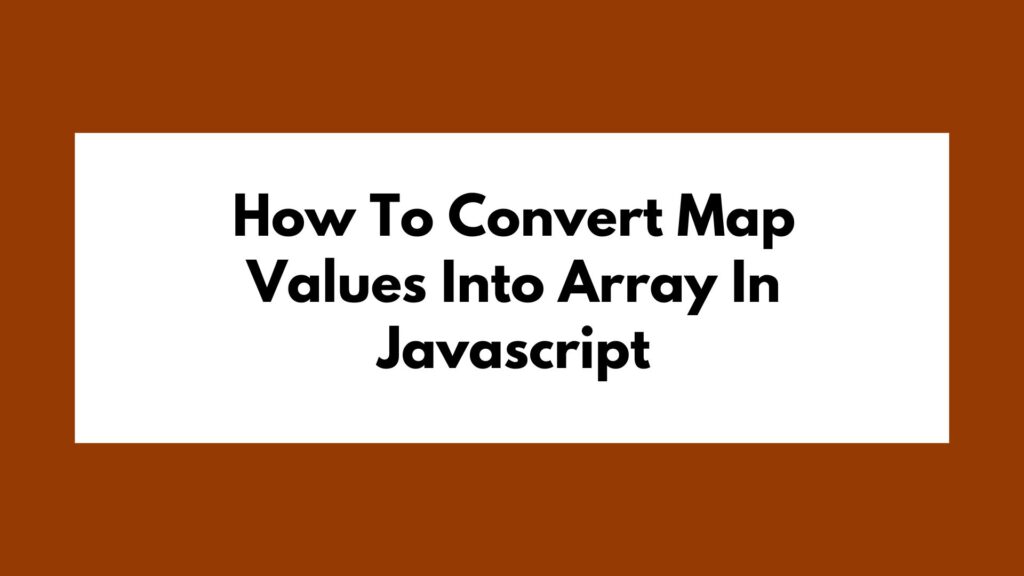
In the world of JavaScript programming, there often arises a need to convert map values into an array for various data manipulation tasks. This process can be quite valuable and is essential for efficient data handling. In this article, we will delve into the intricacies of converting map values into an array, providing you with a step-by-step guide and multiple examples to ensure a clear understanding.
Table of Contents
Step-by-Step Guide to Converting Map Values into Array
Step 1: Creating a Map Object
Firstly, we need to create a Map object in JavaScript to store key-value pairs efficiently. Let’s initialize a sample map for demonstration purposes:
let myMap = new Map([ ['key1', 'value1'], ['key2', 'value2'], ['key3', 'value3'] ]);
Step 2: Converting Map Values into an Array
To convert the values from the map into an array, we can utilize the map.values()
method in JavaScript:
let mapValuesArray = Array.from(myMap.values());
By executing the above code snippet, we can successfully convert the map values into an array.
Step 3: Accessing the Converted Array
Now that we have our array containing the map values, we can easily access and manipulate them as needed:
console.log(mapValuesArray); // Output: ['value1', 'value2', 'value3']
Example Demonstrations
Let’s further illustrate this conversion process with a practical example:
let exampleMap = new Map([ ['name', 'John Doe'], ['age', 30], ['occupation', 'Developer'] ]); let exampleArray = Array.from(exampleMap.values()); console.log(exampleArray); // Output: ['John Doe', 30, 'Developer']
Wrapping Up
In conclusion, converting map values into an array in JavaScript is a fundamental operation that can greatly enhance data manipulation capabilities. By following the simple steps outlined in this article and exploring the provided examples, you can successfully master this process and leverage it in your projects effectively.