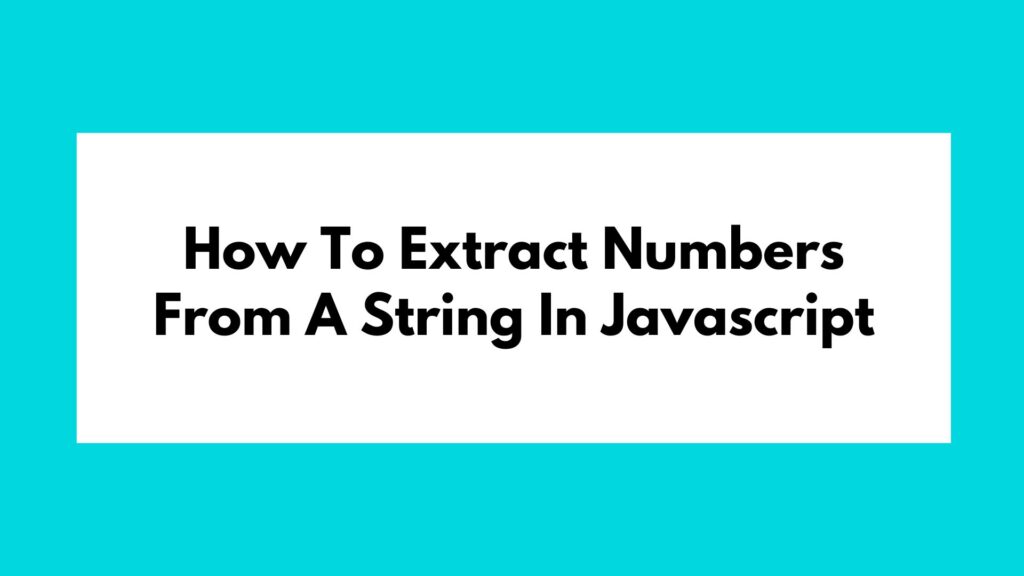
When working with JavaScript, extracting numbers from a string can be a common task. In this article, we will explore various methods to achieve this efficiently. By following these methods, you can enhance your data manipulation capabilities in JavaScript.
Methods To Extract Numbers From A String In Javascript
Method 1: Using Regular Expressions
One of the most common ways to extract numbers from a string in JavaScript is by using regular expressions. Below is a step-by-step breakdown of how you can implement this method:
- Define a regular expression pattern to match numbers in the string.
- Use the
match()
method with the regular expression pattern to extract numbers from the string. - Iterate through the matched numbers to access each extracted number individually.
Here’s a sample code snippet demonstrating the implementation of this method:
const str = 'Extract numbers 123 from this string 456'; const numbers = str.match(/\d+/g); numbers.forEach(number => { console.log(number); });
Method 2: Using JavaScript Functions
Another approach to extracting numbers from a string is by leveraging JavaScript functions. This method involves iterating through each character in the string and identifying numeric characters. Here’s a breakdown of how you can implement this method:
- Create a function that takes a string as input.
- Iterate through each character in the string.
- Check if the character is a numeric value using the
isNaN()
function. - Accumulate numeric characters to form a number.
Below is an example code snippet illustrating the implementation of this method:
function extractNumbers(str) { let result = ''; for (let i = 0; i < str.length; i++) { if (!isNaN(parseInt(str[i]))) { result += str[i]; } } return result; } const str = 'There are 456 numbers in this string.'; const extractedNumbers = extractNumbers(str); console.log(extractedNumbers);
Method 3: Using Split and Filter
Another method to extract numbers from a string is by using the split()
and filter()
methods in JavaScript. This approach involves splitting the string based on non-numeric characters and then filtering out non-numeric parts. Here’s how you can implement this method:
- Use the
split()
method with a regular expression to split the string into an array of substrings. - Apply the
filter()
method to keep only the numeric substrings. - Join the filtered numeric substrings to get the extracted numbers.
Here’s a code snippet demonstrating this method:
const str = 'Extract 123 numbers 456 from this string'; const numbers = str.split(/\D+/).filter(num => num !== '').join(''); console.log(numbers);
Method 4: Using ASCII Values
You can also extract numbers from a string by utilizing ASCII values of characters. By checking the ASCII values, you can identify numeric characters and extract them from the string. Here’s an overview of how you can implement this method:
- Iterate through each character in the string.
- Check the ASCII value of each character to determine if it is a numeric character.
- Accumulate numeric characters to form the extracted numbers.
Below is a code snippet demonstrating the extraction of numbers using ASCII values:
function extractNumbersASCII(str) { let result = ''; for (let i = 0; i < str.length; i++) { if (str.charCodeAt(i) >= 48 && str.charCodeAt(i) <= 57) { result += str[i]; } } return result; } const str = 'This string contains 789 numbers.'; const extractedNumbersASCII = extractNumbersASCII(str); console.log(extractedNumbersASCII);
By incorporating these additional methods into your JavaScript toolkit, you can efficiently extract numbers from strings and optimize your data processing tasks.
Conclusion
In conclusion, mastering the art of extracting numbers from strings in JavaScript opens up new possibilities for data manipulation and analysis. By applying the techniques outlined in this article, you can enhance your coding skills and tackle numerical challenges with confidence and efficiency.