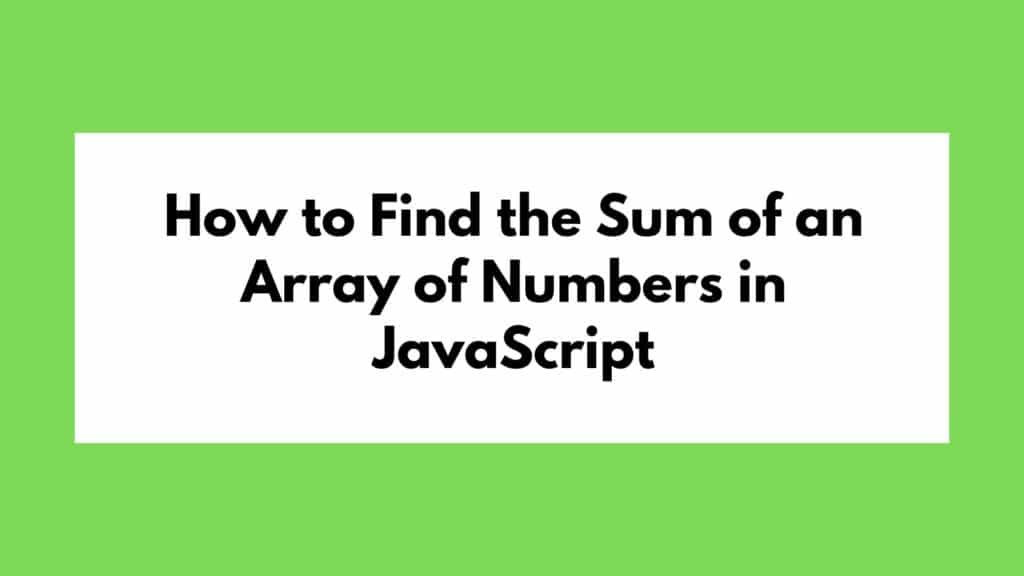
When working with JavaScript, one common task is finding the sum of an array of numbers. This operation is fundamental in programming and can be achieved using various techniques. In this article, we will explore different methods to calculate the sum of an array in JavaScript.
To Find the Sum of an Array of Numbers in JavaScript, We Can Follow These Methods:
Method 1: Using a For Loop
One of the simplest ways to find the sum of an array of numbers is by using a for loop. Below is a code snippet demonstrating this approach:
const numbers = [1, 2, 3, 4, 5]; let sum = 0; for (let i = 0; i < numbers.length; i++) { sum += numbers[i]; } console.log('The sum of the array is: ' + sum);
In this code snippet:
- We initialize a variable
sum
to store the cumulative sum. - We iterate through each element in the
numbers
array using a for loop. - We add each element to the
sum
variable. - Finally, we display the total sum on the console.
Method 2: Using the Reduce Method
Another elegant way to calculate the sum of an array in JavaScript is by utilizing the reduce
method. Here’s how you can achieve this:
const numbers = [1, 2, 3, 4, 5]; const sum = numbers.reduce((acc, curr) => acc + curr, 0); console.log('The sum of the array is: ' + sum);
In this code snippet:
- We use the
reduce
method to accumulate the sum of all elements in the array. - The initial value of the accumulator (
acc
) is set to 0. - The reducer function adds each element (
curr
) to the accumulator.
Method 3: Using the forEach Method
The forEach
method allows you to iterate over an array and perform an operation on each element. You can use this method to calculate the sum of an array as shown below:
const numbers = [1, 2, 3, 4, 5]; let sum = 0; numbers.forEach(function(number) { sum += number; }); console.log('The sum of the array is: ' + sum);
In this code snippet:
- We initialize the
sum
variable to store the cumulative sum. - We use the
forEach
method to iterate over each element in thenumbers
array. - For each element, we add its value to the
sum
variable. - Finally, we display the total sum on the console.
By utilizing the forEach
method, you can achieve the same result of finding the sum of an array in a concise and readable manner. This method offers an alternative approach to handling array operations in JavaScript and further enhances your programming skills.
Conclusion
In conclusion, finding the sum of an array of numbers in JavaScript is a common task that can be approached using different methods. In this article, we explored two techniques: using a for loop and leveraging the reduce
method. By understanding these approaches and practicing with examples, you can enhance your JavaScript programming skills.