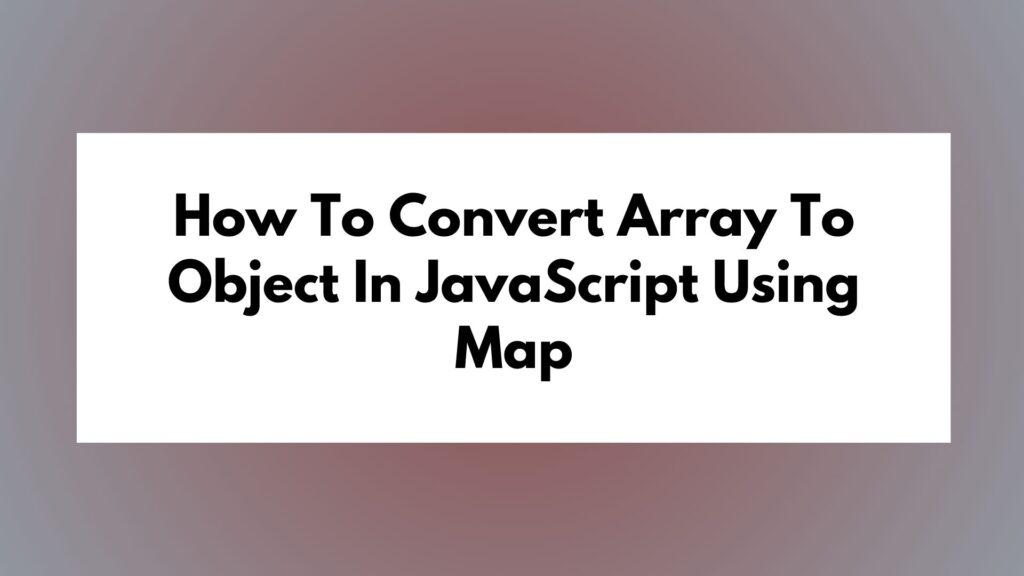
In the realm of JavaScript programming, the conversion of arrays to objects is a task that often arises. This process can be efficiently achieved using the map
method, a powerful tool in the JavaScript developer’s arsenal. By understanding and implementing this method effectively, you can streamline your code and enhance its readability. In this article, we will explore various methods of converting an array to an object in JavaScript using the map
method.
Understanding the map
Method
Before we delve into the specifics of converting arrays to objects, let’s first grasp the essence of the map
method in JavaScript. The map
method is used to iterate over an array and transform each element within it based on a provided callback function. This function is applied to each element, and a new array containing the results is returned.
Method 1: Using map
Method
const array = [1, 2, 3, 4, 5]; const object = Object.assign({}, array.map((value, index) => ({ ['key' + index]: value })));
Let’s break down the code step by step:
- We define an array containing numeric values.
- We use the
map
method to iterate over each element of the array. - Inside the callback function, we create an object with dynamically generated keys (‘key0’, ‘key1’, etc.) and assign each element of the array as the corresponding value.
- Finally, we use
Object.assign
to merge all key-value pairs into a single object.
Method 2: Using Object.fromEntries
const array = [['key1', 'value1'], ['key2', 'value2']]; const object = Object.fromEntries(array.map(([key, value]) => [key, value]));
In this alternative method:
- We define an array containing sub-arrays with key-value pairs.
- We use the
map
method to transform each sub-array into an array of key-value pairs. - We then apply
Object.fromEntries
to convert this array of key-value pairs into an object.
Method 3: Using Reduce Method
const array = [1, 2, 3, 4, 5]; const object = array.reduce((acc, cur, index) => ({ ...acc, ['key' + index]: cur }), {});
In this approach:
- We utilize the
reduce
method to accumulate key-value pairs into a single object. - The initial value of
{}
signifies an empty object. - For each element in the array, we incrementally build the object with dynamically generated keys.
Method 4: Using ForEach Loop
const array = [1, 2, 3, 4, 5]; const object = {}; array.forEach((value, index) => { object['key' + index] = value; });
Here:
- We iterate over each element of the array using a
forEach
loop. - Within the loop, we assign each element to the object with dynamically generated keys.
Conclusion
Mastering different methods of converting arrays to objects using the map
method empowers you to manipulate data structures effectively in JavaScript. By experimenting with these techniques and understanding their nuances, you can enhance your coding proficiency and tackle diverse programming challenges with ease. Embrace the versatility of JavaScript and continue honing your skills to become a proficient developer in this dynamic language. Happy coding!