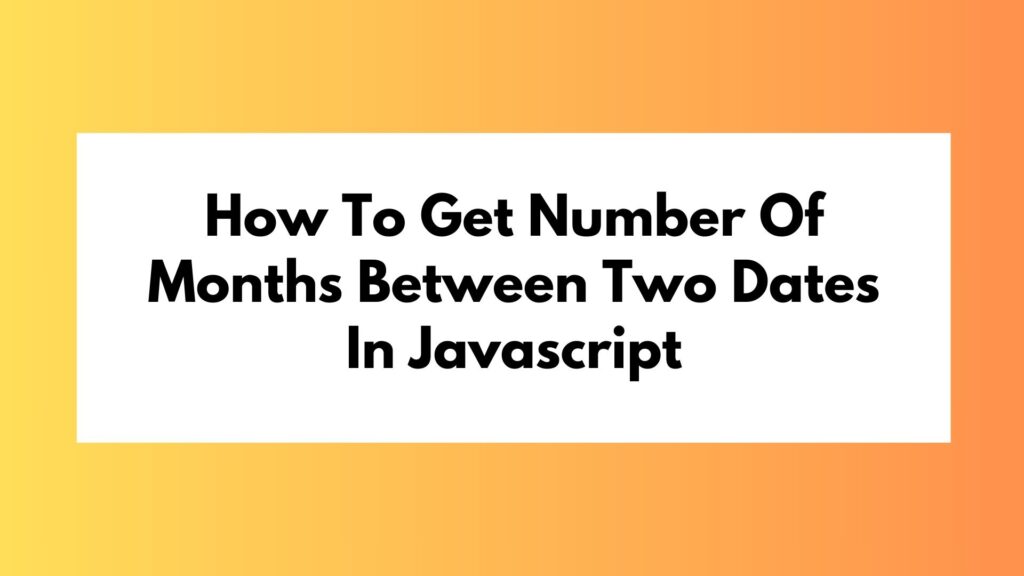
In JavaScript, determining the number of months between two dates is a common task that can be approached through various methods. In this article, we will explore multiple techniques to achieve this calculation effectively.
Methods To Get Number Of Months Between Two Dates In Javascript
Method 1: Utilizing Date Objects
Learn how to calculate the number of months between two dates using JavaScript’s Date objects.
Step 1: Initialize the Dates
Initialize Date objects for the two dates you want to compare.
const date1 = new Date('2023-01-15'); const date2 = new Date('2024-03-20');
Step 2: Calculate the Difference
Compute the difference in months between the two dates.
const diffYears = date2.getFullYear() - date1.getFullYear(); const diffMonths = (diffYears * 12) + (date2.getMonth() - date1.getMonth());
Step 3: Display the Result
Output the number of months between the two dates.
console.log('Number of months between the two dates:', diffMonths);
By following these steps, you can accurately determine the number of months between two dates using JavaScript’s native Date objects.
Method 3: Using Moment.js Library
Explore how to leverage the Moment.js library for efficient date calculations and determining the number of months between two dates in JavaScript.
Step 1: Initialize Moment.js
Include Moment.js library in your project.
const moment = require('moment');
Step 2: Initialize Dates with Moment
Create Moment objects for the two dates you want to compare.
const date1 = moment('2023-01-15'); const date2 = moment('2024-03-20');
Step 3: Calculate the Difference
Find the difference in months between the two dates using Moment.js methods.
const diffMonths = date2.diff(date1, 'months');
Step 4: Display the Result
Display the number of months between the two dates.
console.log('Number of months between the two dates:', diffMonths);
By incorporating Moment.js into your project, you can leverage its powerful features to simplify date calculations effectively in JavaScript applications.
Conclusion
Exploring different methods to calculate the number of months between two dates in JavaScript provides a well-rounded understanding of date manipulation techniques. Whether you choose to work with native Date objects or utilize external libraries like Moment.js, selecting the appropriate method based on your project requirements can streamline your development process and improve code efficiency.