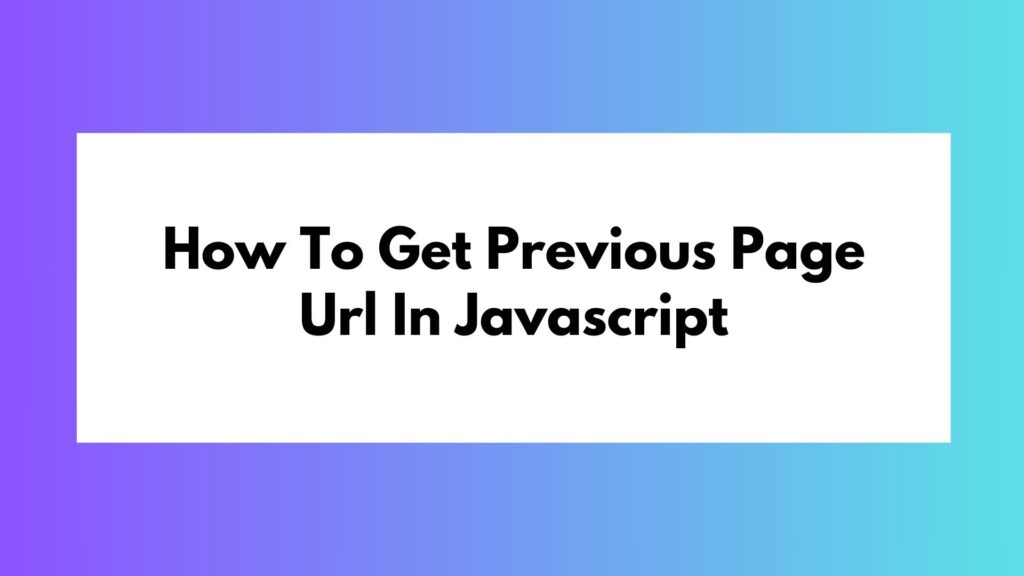
In the dynamic world of web development, JavaScript plays a pivotal role in enhancing user experience. One common requirement is to retrieve the URL of the previous page, which can be useful for various purposes such as navigation, analytics, or personalization. In this article, we’ll delve into different methods to accomplish this task using JavaScript
Methods To Get Previous Page Url In Javascript
Method 1: Utilizing document.referrer Property
The simplest method to obtain the URL of the previous page is by accessing the document.referrer
property. This property returns the URL of the document that loaded the current document. Let’s break down the code:
const previousPageUrlMethod1 = document.referrer; console.log("Method 1 - Previous Page URL:", previousPageUrlMethod1);
Explanation:
- We directly access the
document.referrer
property to retrieve the URL of the previous page. - This method is straightforward but may not always provide the desired result, especially if the browser settings or security protocols restrict access to the referrer information.
Method 2: Leveraging History Object
Another approach involves utilizing the history
object provided by the browser. Specifically, we can use the history.back()
method to navigate to the previous page and then access the URL. Here’s the code:
// Test Method 2: Leveraging History Object function getPreviousPageUrl() { history.back(); return location.href; } const previousPageUrlMethod2 = getPreviousPageUrl(); console.log("Method 2 - Previous Page URL:", previousPageUrlMethod2);
Explanation:
- The
history.back()
method navigates to the previous page in the session history. - After navigating back, we retrieve the URL of the current page using
location.href
. - This method provides more control over the navigation but may not be suitable for all scenarios, especially if the user doesn’t have control over the navigation flow.
Method 3: Storing Previous Page URL in Session Storage
For scenarios where precise control over the previous page URL is required, we can store the URL in session storage when navigating between pages. Here’s how we can implement it:
// On the previous page sessionStorage.setItem('previousPageUrl', window.location.href); // On the current page const previousPageUrlMethod3 = sessionStorage.getItem('previousPageUrl'); console.log("Method 3 - Previous Page URL:", previousPageUrlMethod3);
Explanation:
- We store the URL of the previous page in session storage using
sessionStorage.setItem()
before navigating away from the page. - On the subsequent page, we retrieve the stored URL using
sessionStorage.getItem()
.
Conclusion:
In this article, we explored various methods to retrieve the URL of the previous page using JavaScript. Whether it’s accessing the referrer information directly, leveraging the history object, or storing the URL in session storage, each method offers distinct advantages depending on the requirements of your web application. By understanding these techniques, you can enhance the functionality and usability of your web projects.