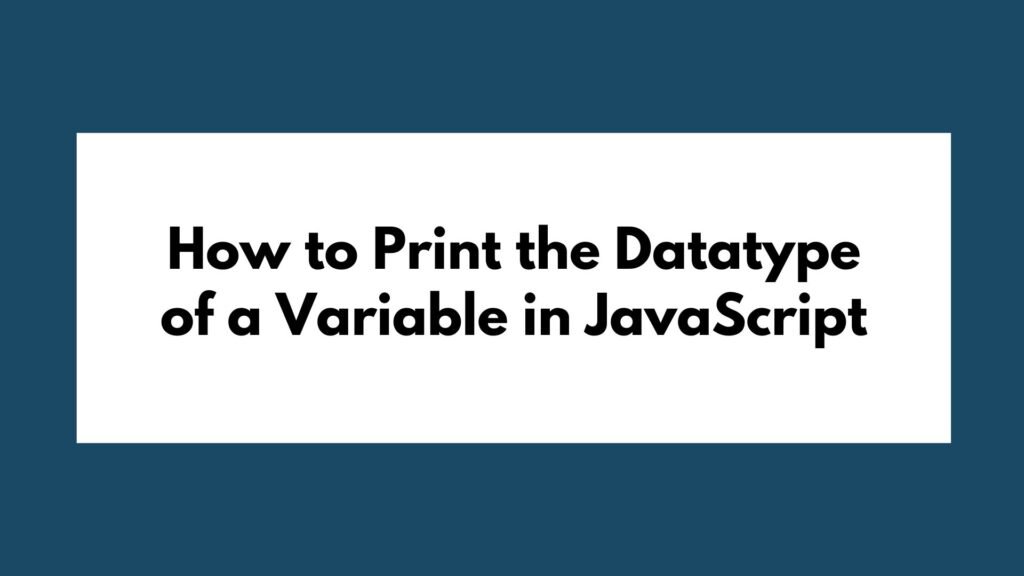
In the world of programming, understanding the datatype of a variable is fundamental. JavaScript, being a dynamically typed language, allows variables to hold values of different types during the execution of a program. In this article, we will explore various methods to print the datatype of a variable in JavaScript.
Methods to Print the Datatype of a Variable in JavaScript:
Method 1: Using the typeof Operator
The typeof
operator is a simple and quick way to determine the datatype of a variable in JavaScript. It returns a string indicating the type of the operand.
Here’s how you can use the typeof
operator:
let x = 10; console.log(typeof x); // Output: 'number'
Method 2: Using the Object.prototype.toString()
Another approach to determine the datatype of a variable is by using the Object.prototype.toString()
method. This method returns a string representing the object’s type.
Follow these steps to utilize Object.prototype.toString()
:
let y = 'Hello'; console.log(Object.prototype.toString.call(y)); // Output: '[object String]'
Method 3: Using instanceof Operator
The instanceof
operator checks if an object belongs to a specific class. This can be used to determine if a variable is an instance of a particular datatype.
Here’s an example of using the instanceof
operator:
let z = [1, 2, 3]; console.log(z instanceof Array); // Output: true
Method 4: Using the constructor Property
In JavaScript, each object has a constructor
property that refers to the constructor function used to create the object. By accessing the constructor
property of an object, you can determine the datatype of the variable.
Here’s how you can utilize the constructor
property:
let a = true; console.log(a.constructor); // Output: [Function: Boolean]
Method 5: Using the typeof Operator with Null
One interesting aspect of the typeof
operator is how it handles null
values. When using typeof
with null
, it returns 'object'
, which can be surprising to many developers.
Check out this example with null
:
let b = null; console.log(typeof b); // Output: 'object'
Summary
In this article, we explored five methods to print the datatype of a variable in JavaScript. By using the typeof
operator, Object.prototype.toString()
method, instanceof
operator, constructor
property, and considering null
values, you now have a diverse set of techniques to determine variable types in your JavaScript code.
Understanding these methods not only enhances your debugging skills but also contributes to writing more robust and maintainable code. Incorporate these techniques into your coding practices to elevate your proficiency as a JavaScript developer.
Stay tuned for more insightful articles on JavaScript and happy coding!