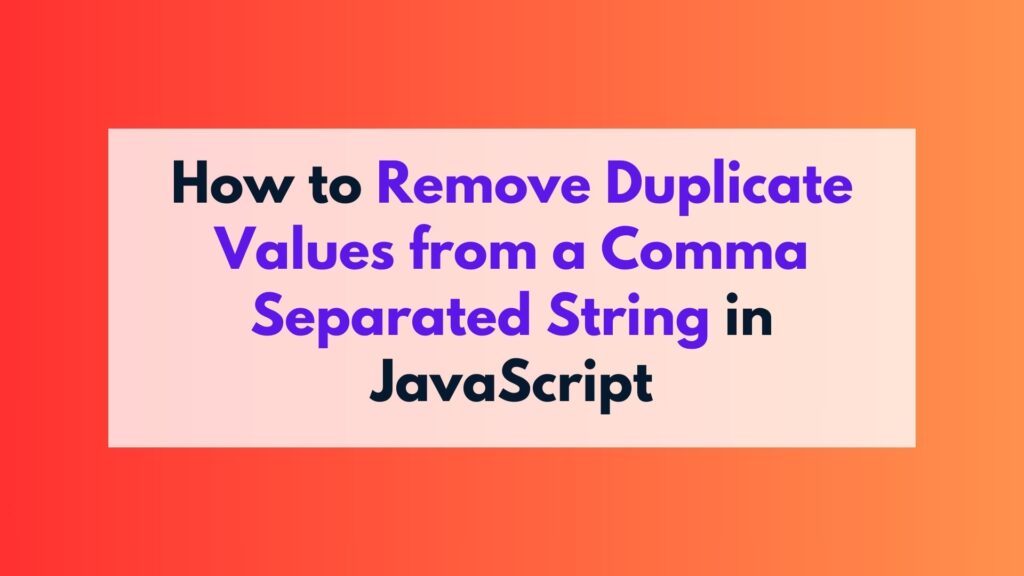
In this article, we will explore how to remove duplicate values from a comma-separated string using JavaScript. Duplicate values can often lead to unexpected behavior in our code, and it’s important to have a reliable method to eliminate them. We will dive into the implementation details and provide step-by-step examples to ensure a clear understanding of the process.
To remove duplicate values from a comma-separated string in JavaScript, we can follow these steps:
Step 1: Split the String into an Array
The first step is to split the comma-separated string into an array of individual values. We can achieve this using the split()
method. Let’s consider an example:
const inputString = "apple,banana,orange,apple,grape"; const arrayOfValues = inputString.split(",");
In this example, we have a comma-separated string containing multiple values. By splitting the string using the comma delimiter, we obtain an array arrayOfValues
that contains all the individual values.
Step 2: Remove Duplicate Values Using a Set
Once we have the array of values, we can use a Set to eliminate duplicate entries. A Set is a built-in JavaScript object that allows us to store unique values only. We can create a Set from our array and then convert it back to an array to remove any duplicates. Here’s an example:
const uniqueArray = [...new Set(arrayOfValues)];
In this example, we use the spread operator (...
) along with the Set
constructor to create a new Set object from arrayOfValues
. Then, by wrapping it within square brackets []
, we convert it back to an array uniqueArray
that contains only unique values.
Step 3: Convert the Array Back to a Comma-Separated String
Finally, we need to convert the unique array back into a comma-separated string. We can achieve this using the join()
method. Here’s an example:
const uniqueString = uniqueArray.join(",");
In this example, we use the join()
method with a comma as the delimiter to concatenate all elements of uniqueArray
into a single string uniqueString
, where each value is separated by a comma.
Examples
Let’s explore some additional examples to solidify our understanding:
Example 1:
const inputString = "apple,banana,orange,apple,grape"; const arrayOfValues = inputString.split(","); const uniqueArray = [...new Set(arrayOfValues)]; const uniqueString = uniqueArray.join(","); console.log(uniqueString);
Result:
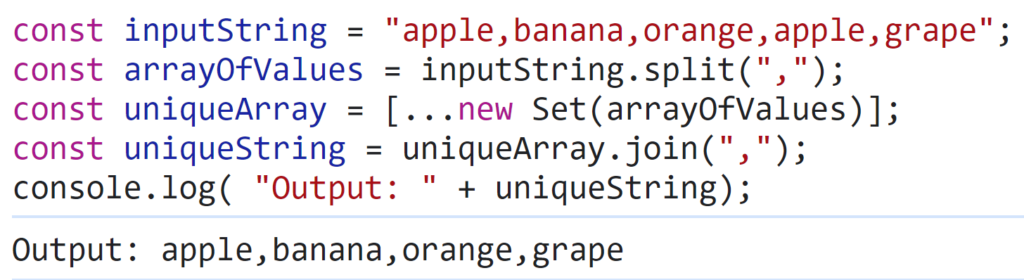
In this example, we start with a comma-separated string containing duplicate values. After applying the necessary steps, we obtain a new string without any duplicates.
Example 2:
const inputString = "red,green,blue,green,yellow,yellow"; const arrayOfValues = inputString.split(","); const uniqueArray = [...new Set(arrayOfValues)]; const uniqueString = uniqueArray.join(","); console.log(uniqueString);
Result:

Here, we have a comma-separated string with multiple duplicate values. The resulting string after removing duplicates only contains unique values.
Conclusion
In this article, we learned how to remove duplicate values from a comma-separated string in JavaScript. By following the outlined steps – splitting the string into an array, using a Set to remove duplicates, and converting it back into a string – we can ensure that our code handles duplicate values effectively. Remember to apply these techniques whenever you encounter comma-separated strings with duplicate entries in your JavaScript projects.