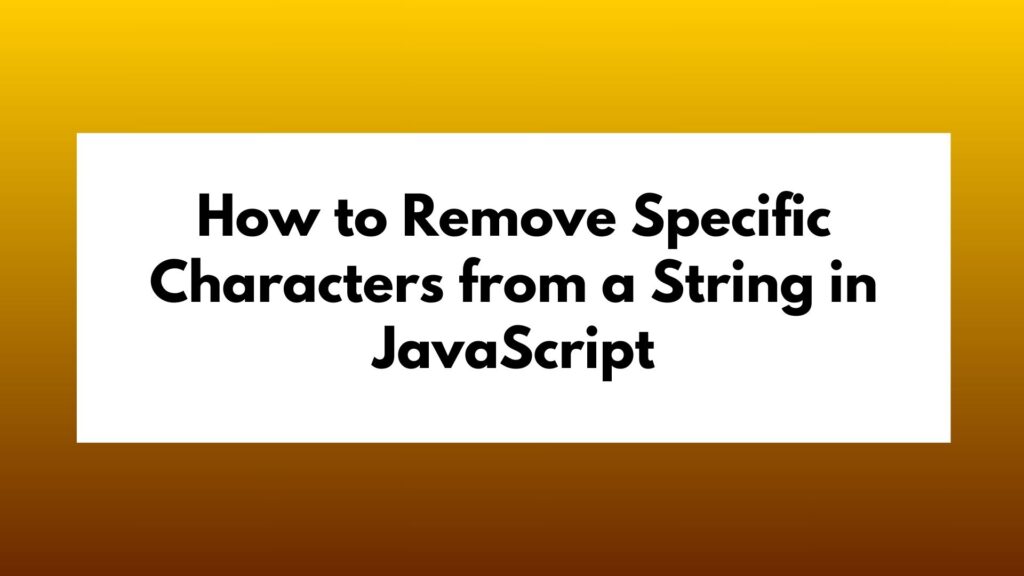
In the world of web development, JavaScript is a versatile language that empowers developers to manipulate strings efficiently. Handling multiple specific characters removal from a string is a common requirement in various programming scenarios. In this article, we will explore different methods to remove specific characters from a string in JavaScript.
Methods to Remove Specific Characters from a String in JavaScript
Method 1: Using the split()
and join()
Methods
One simple approach to remove a specific character from a string is by utilizing the split()
and join()
methods in JavaScript.
Step 1: Splitting the String
Use the split()
method to split the original string into an array of substrings based on the specified character.
let str = "Hello, World!"; let charToRemove = ","; let strArray = str.split(charToRemove);
Step 2: Joining the Array
Rejoin the array elements using the join()
method to create a new string without the specified character.
let newStr = strArray.join(""); console.log(newStr); // Output: "Hello World!"
Method 2: Utilizing Regular Expressions
Another effective method to remove a specific character from a string is by leveraging regular expressions.
Step 1: Using replace()
Method
Employ the replace()
method along with a regular expression to replace all instances of the specified character.
let str = "Hello, World!"; let charToRemove = ","; let newStr = str.replace(new RegExp(charToRemove, 'g'), ''); console.log(newStr); // Output: "Hello World!"
Method 3: Removing Multiple Specific Characters
Expanding on the use of regular expressions, we can address the removal of multiple specific characters from a string in JavaScript.
Step 1: Define an Array of Characters to Remove
Create an array containing all the specific characters you want to remove from the string.
let str = "Hello, World!"; let charsToRemove = [",", "!"];
Step 2: Construct Regular Expression Dynamically
Generate a regular expression pattern dynamically based on the characters to remove.
let pattern = new RegExp(charsToRemove.join("|"), 'g');
Step 3: Apply replace()
Method
Utilize the replace()
method with the dynamically created regular expression to remove all instances of the specified characters.
let newStr = str.replace(pattern, ''); console.log(newStr); // Output: "Hello World"
By understanding and implementing these methods, you can effectively handle various scenarios of removing specific characters from strings in JavaScript, enhancing your text manipulation capabilities.
Conclusion
Mastering string manipulation techniques like removing specific characters is essential for any JavaScript developer. With the methods covered in this article, you now have a solid foundation to tackle text processing tasks efficiently. Enhance your coding skills and elevate your programming capabilities with practical solutions for common programming challenges.