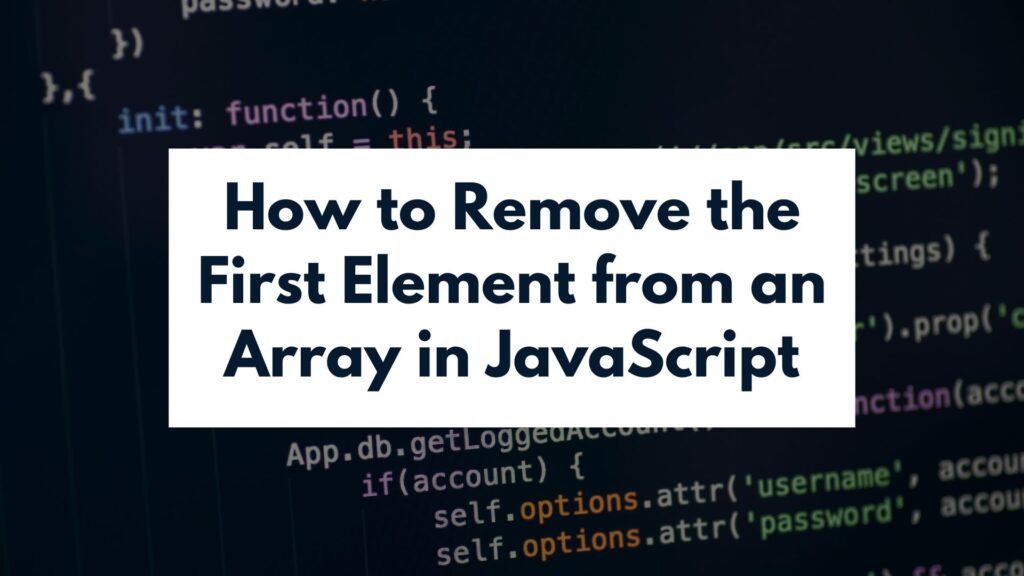
JavaScript is a versatile programming language widely used for web development. When working with arrays in JavaScript, it’s common to need to manipulate their contents. One frequent operation is removing the first element from an array. In this article, we’ll explore various methods to achieve this, accompanied by examples and in-depth explanations.
This article provides three methods to remove the first element from an array in JavaScript.
Method 1: Using Array.shift():
The shift()
method is specifically designed to remove the first element from an array. It modifies the original array and returns the removed element.
let fruits = ['apple', 'banana', 'orange', 'grape']; let removedFruit = fruits.shift(); console.log('Removed Fruit:', removedFruit); console.log('Updated Array:', fruits);
Result:
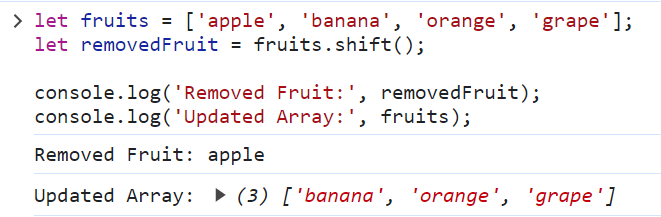
Explanation:
shift()
removes the first element (‘apple’) from the ‘fruits’ array and returns it.- The removed element is stored in the ‘removedFruit’ variable.
- The ‘fruits‘ array is now updated without the first element.
Method 2: Using Array.slice():
The slice()
method can be used to create a new array that excludes the first element.
let fruits = ['apple', 'banana', 'orange', 'grape']; let updatedFruits = fruits.slice(1); console.log('Updated Array:', updatedFruits); console.log('Original Array:', fruits);
Result
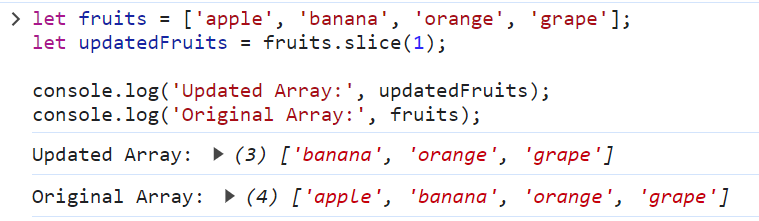
Explanation:
slice(1)
creates a new array containing all elements from index 1 to the end of the original ‘fruits’ array.- The ‘updatedFruits’ array now represents the original array without the first element.
- The original ‘fruits’ array remains unchanged.
Method 3: Using Array.splice():
The splice()
method can both remove and insert elements into an array. To remove the first element, set the start index to 0 and the delete count to 1.
let fruits = ['apple', 'banana', 'orange', 'grape']; fruits.splice(0, 1); console.log('Updated Array:', fruits);
Result
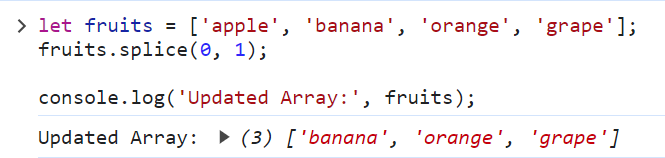
Explanation:
splice(0, 1)
removes one element starting from index 0 of the ‘fruits’ array.- The ‘fruits’ array is updated in place without the first element.
Conclusion
Removing the first element from an array in JavaScript can be accomplished through various methods. The choice between these methods depends on factors such as whether you want to modify the original array or create a new one. Understanding these techniques empowers developers to efficiently manipulate arrays in their JavaScript applications.