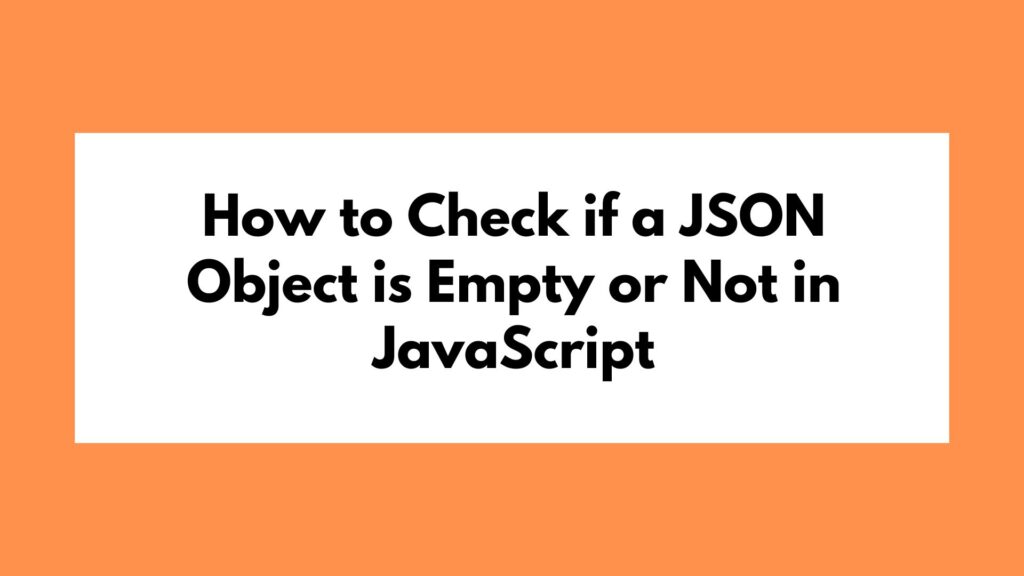
In the realm of JavaScript development, working with JSON objects is a common task. Knowing how to determine whether a JSON object is empty or contains data is crucial for robust data handling. In this article, we will explore effective methods to check the emptiness of a JSON object in JavaScript.
To Check If a Json Object Is Empty or Not in Javascript, We Can Follow These Methods:
Method 1: Using Object.keys()
To check if a JSON object is empty, we can leverage the Object.keys()
method. This method returns an array of a given object’s enumerable property names. By comparing the length of this array to zero, we can easily determine if the object is empty.
const isEmpty = (obj) => { return Object.keys(obj).length === 0; }; const myObject = {}; console.log(isEmpty(myObject)); // Output: true
Method 2: Using JSON.stringify()
Another approach involves using JSON.stringify()
to convert the object into a JSON string and then comparing it with an empty object. This method provides a straightforward way to check for an empty JSON object.
const isEmpty = (obj) => { return JSON.stringify(obj) === '{}'; }; const myObject = {}; console.log(isEmpty(myObject)); // Output: true
Method 3: Using a For…In Loop
By iterating through the keys of the object using a for...in
loop and checking for the presence of any keys, we can determine if the object is empty.
const isEmpty = (obj) => { for (let key in obj) { if (obj.hasOwnProperty(key)) { return false; } } return true; }; const myObject = {}; console.log(isEmpty(myObject)); // Output: true
Method 4: Using Object.values()
This method checks if an object is empty by getting the values of the object’s properties and verifying if the length of the values array is zero.
const isEmpty = (obj) => { return Object.values(obj).length === 0; }; const myObject = {}; console.log(isEmpty(myObject)); // Output: true
Method 5: Using lodash.isEmpty()
Utilizing the isEmpty()
function from the lodash library allows for a simple check to determine if an object is empty.
const _ = require('lodash'); const myObject = {}; console.log(_.isEmpty(myObject)); // Output: true
Method 6: Checking for Null or Undefined Values
This method includes a check to see if the object is null or undefined, along with verifying if the object has no enumerable properties.
const isEmpty = (obj) => { return obj === null || obj === undefined || Object.keys(obj).length === 0; }; const myObject = {}; console.log(isEmpty(myObject)); // Output: true
Conclusion
Incorporate these methods into your JavaScript projects to efficiently validate and handle empty JSON objects. By following the provided explanations and code snippets, you can enhance your data processing capabilities and ensure robust data management in your applications. Stay informed, stay efficient, and keep coding!