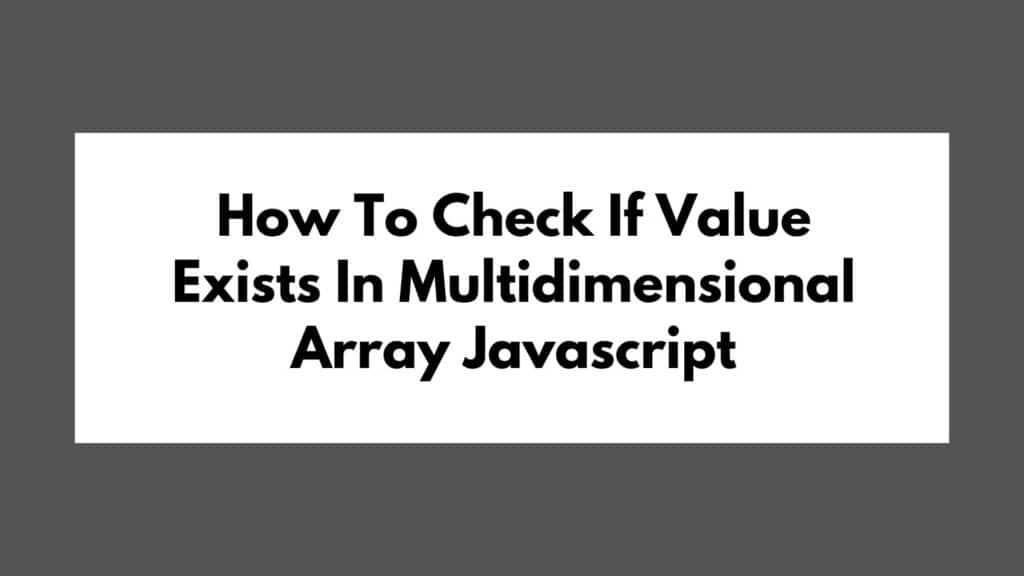
When working with multidimensional arrays in JavaScript, it is essential to check if a specific value exists within the array structure. This process involves traversing through the nested arrays and comparing the values to determine the presence of the target value. In this article, we will explore how to effectively check for the existence of a value in a multidimensional array using JavaScript.
Table of Contents
Understanding the Basics
Before we delve into the coding examples, let’s first establish a clear understanding of how multidimensional arrays work in JavaScript. A multidimensional array is an array that contains other arrays as its elements. This creates a nested structure, allowing for more complex data organization.
Checking for Value Existence
To check if a value exists in a multidimensional array, we need to iterate through each element of the array and perform a comparison to determine a match. We can achieve this using various methods like nested loops or recursion.
Using Nested Loops
One common approach is to use nested loops to traverse through the array elements. Here’s a simple example demonstrating this method:
function checkValueInArray(arr, target) { for (let i = 0; i < arr.length; i++) { for (let j = 0; j < arr[i].length; j++) { if (arr[i][j] === target) { return true; } } } return false; }
In the above code snippet, the checkValueInArray
function takes the multidimensional array arr
and the target value target
as parameters. It then iterates through each element of the array using nested loops and checks for a match with the target value.
Using Recursion
Another method to check for value existence is through recursion. Recursion can be a powerful tool for navigating complex data structures like multidimensional arrays. Here’s an example of a recursive function to check for a value:
function checkValueInArray(arr, target) { for (let i = 0; i < arr.length; i++) { if (Array.isArray(arr[i])) { if (checkValueInArray(arr[i], target)) { return true; } } else if (arr[i] === target) { return true; } } return false; }
In this recursive approach, the checkValueInArray
function calls itself for each nested array within the multidimensional array until a match is found or all elements have been traversed.
Conclusion
In conclusion, checking for the existence of a value in a multidimensional array in JavaScript requires traversing through the nested structure and comparing each element with the target value. By utilizing nested loops or recursion, you can efficiently determine whether a specific value is present within the array. Experiment with the provided code snippets and adapt them to suit your specific requirements when working with multidimensional arrays in JavaScript.