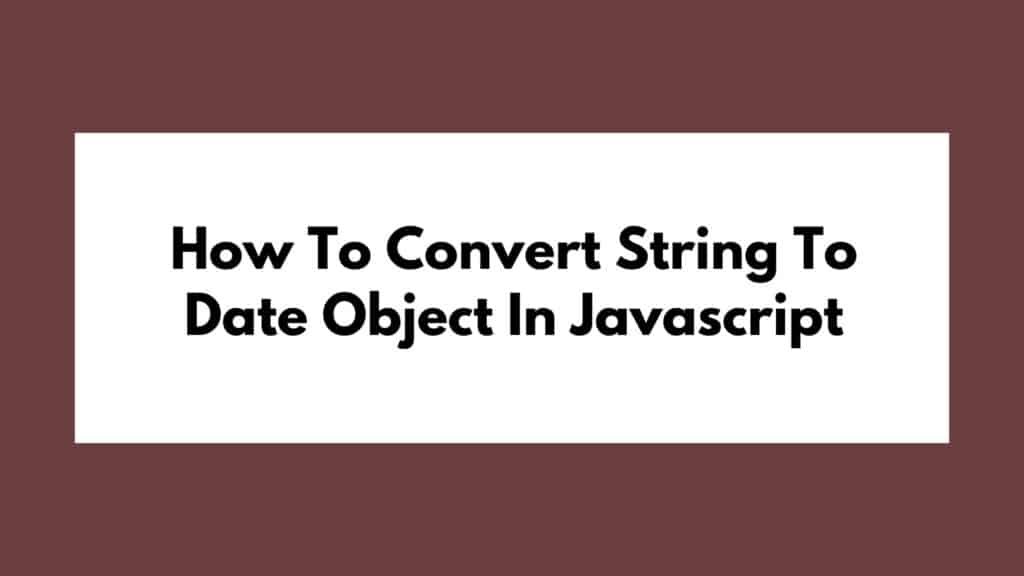
In JavaScript, converting a string to a date object is a common task that developers encounter. This process involves parsing a date string and creating a corresponding Date object that can be manipulated and formatted as needed. In this article, we will explore various methods and techniques to achieve this conversion effectively.
To Convert String To Date Object In Javascript we can follow these methods:
Understanding the Date Object in JavaScript
Before diving into the conversion process, it’s essential to understand the Date object in JavaScript. The Date object represents a date and time value and provides various methods for working with dates.
Method 1: Using the new Date()
Constructor
The most straightforward way to convert a string to a date object is by using the new Date()
constructor. This method automatically parses the date string and creates a Date object.
const dateString = '2024-03-15'; const dateObject = new Date(dateString); console.log(dateObject);
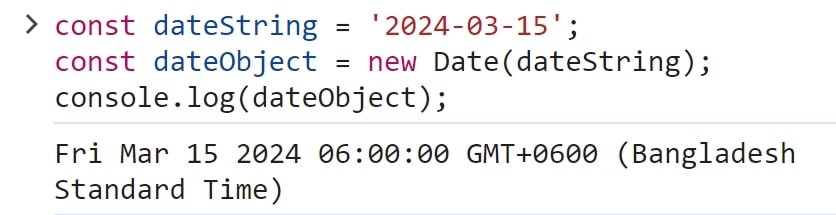
In this example, the dateString
variable contains the date string ‘2024-03-15,’ which is then passed to the new Date()
constructor to create a Date object.
Method 2: Parsing Date Components
Another approach to convert a string to a date object is by parsing the date components manually. This method allows for more flexibility in handling different date formats.
const dateParts = '2024-03-15'.split('-'); const year = parseInt(dateParts[0]); const month = parseInt(dateParts[1]) - 1; // Month is zero-based const day = parseInt(dateParts[2]); const customDateObject = new Date(year, month, day); console.log(customDateObject);
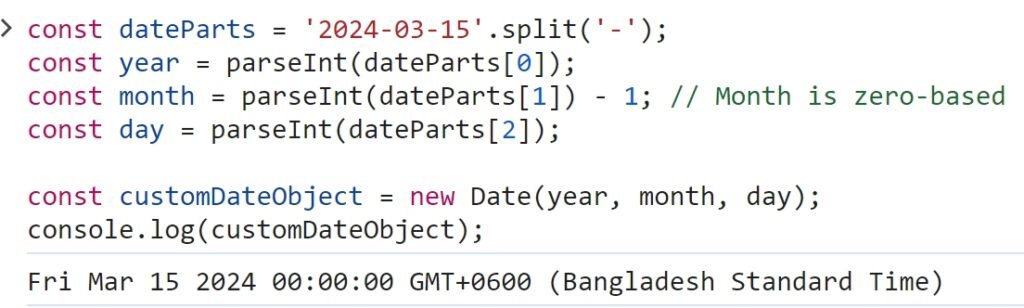
In this code snippet, the date string ‘2024-03-15’ is split into its components (year, month, day) and then used to create a custom Date object.
Conclusion
Converting a string to a date object in JavaScript is a fundamental operation with various methods and approaches. By understanding the Date object and utilizing techniques like the new Date()
constructor and manual parsing, developers can effectively work with dates in their applications.