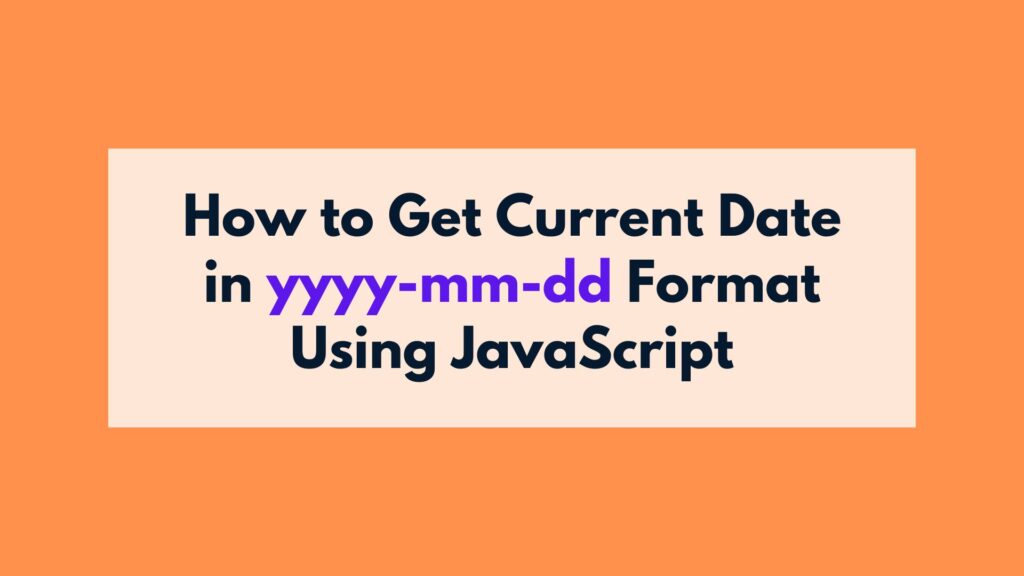
To obtain the current date in the yyyy-mm-dd format using JavaScript, you can leverage the built-in Date object and a few simple methods. Here’s an example code snippet:
const currentDate = new Date().toISOString().split('T')[0];
In JavaScript, obtaining the current date is a common requirement for many web applications. The yyyy-mm-dd format, also known as the ISO 8601 date format, is widely used in various systems and APIs. This article will guide you through the process of getting the current date in the desired format using JavaScript.
Table of Contents
Methodology
To get the current date in the yyyy-mm-dd format, you can follow these steps:
Step 1: Create a new Date object
Create a new Date object using the new Date()
constructor. This will initialize the object with the current date and time.
const currentDate = new Date();
Step 2: Utilize the toISOString()
method
Utilize the toISOString()
method of the Date object. This method returns a string representation of the date in the ISO 8601 format.
const currentDateISO = currentDate.toISOString();
Step 3: Split the string at ‘T’
Split the resulting string at the ‘T’ character using the split()
method. This will yield an array with two elements: the date part and the time part.
const currentDateArray = currentDateISO.split('T');
Step 4: Access the date part
Access the first element of the array, which represents the date part, using index 0.
const currentDatePart = currentDateArray[0];
Step 5: Get the yyyy-mm-dd format
Voilà! You now have the current date in the yyyy-mm-dd format.
const yyyy_mm_dd = currentDatePart;
Examples
Let’s explore a few examples to illustrate how to obtain the current date in yyyy-mm-dd format using JavaScript:
Example 1: Logging the current date
const currentDate = new Date().toISOString().split('T')[0]; console.log(currentDate); // Output: 2023-12-03
Explanation: In this example, we directly log the current date in yyyy-mm-dd format to the console using a one-liner code. The new Date().toISOString().split('T')[0]
expression gets the current date, converts it to ISO string format, splits it at ‘T’, and retrieves only the date part.
Example 2: Displaying the current date on a webpage
const currentDate = new Date().toISOString().split('T')[0]; document.getElementById("dateContainer").innerText = currentDate;
Explanation: In this example, we assign the current date in yyyy-mm-dd format to an HTML element with the id “dateContainer”. By accessing the element’s innerText property, we set its content to be the current date.
Example 3: Using a function to get the current date
function getCurrentDate() { const currentDate = new Date().toISOString().split('T')[0]; return currentDate; }
Explanation: In this example, we define a function called getCurrentDate()
. Inside the function, we follow the same steps as before to obtain the current date in yyyy-mm-dd format. Finally, we return the result.
Conclusion
In this article, we explored how to obtain the current date in the yyyy-mm-dd format using JavaScript. By leveraging the Date object’s toISOString()
method and simple string manipulation, we can easily extract the desired format. Remember to create a new Date object and apply the necessary methods to achieve the desired result. Now you have a handy solution for obtaining the current date in yyyy-mm-dd format for your JavaScript projects.