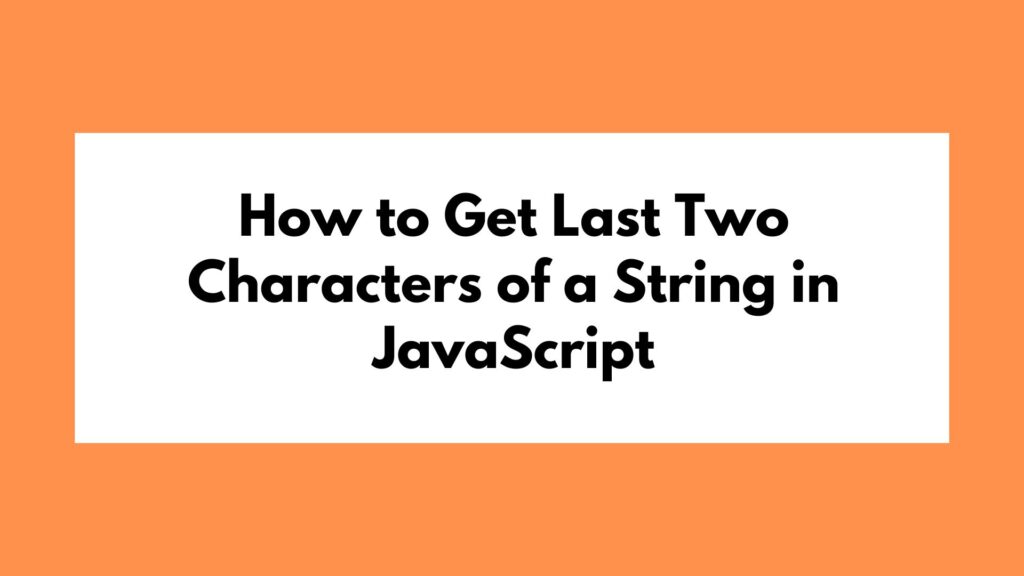
When working with strings in JavaScript, it’s common to need to extract specific parts of a string. In this article, we will explore various methods to get the last two characters of a string using JavaScript.
Method 1: Using substring
Method
One simple way to achieve this is by using the substring
method in JavaScript. Here’s how you can do it:
const str = "Hello World"; const lastTwoChars = str.substring(str.length - 2); console.log(lastTwoChars); // Outputs "ld"
Explanation:
- We start by defining the original string
str
. - By using
str.length - 2
, we can get the starting index for the last two characters. - Then, we use
substring
to extract the last two characters from the original string.
Method 2: Using Array Indexing
Another approach is to treat the string as an array of characters and use array indexing to retrieve the last two characters:
const str = "JavaScript"; const lastTwoChars = str.slice(-2); console.log(lastTwoChars); // Outputs "pt"
Explanation:
- The
slice
method can accept negative numbers as arguments, making it easy to extract the last two characters of a string.
Method 3: Using Regular Expressions
Regular expressions can also be used to solve this problem. Here’s how you can do it:
const str = "Coding"; const lastTwoChars = str.match(/.{0,2}$/)[0]; console.log(lastTwoChars); // Outputs "ng"
Explanation:
- We use a regular expression
/.{0,2}$/
to match the last two characters of the string.
Method 4: Using substr
Method
The substr
method can also be employed to extract the last two characters of a string:
const str = "Programming"; const lastTwoChars = str.substr(-2); console.log(lastTwoChars); // Outputs "ng"
Explanation:
- With a negative starting index, the
substr
method can retrieve the last two characters from the string.
Method 5: Converting String to Array and Slicing
By converting the string into an array and then using Array slicing, we can get the last two characters:
const str = "Array"; const lastTwoChars = str.split('').slice(-2).join(''); console.log(lastTwoChars); // Outputs "ay"
Explanation:
- We first split the string into an array of characters.
- Using
slice(-2)
on the array allows us to extract the last two elements. - Finally, we join the sliced array back into a string to get the last two characters.
Conclusion
In this article, we have explored five different methods for extracting the last two characters of a string in JavaScript. Each method offers a unique approach to achieving the desired outcome. By understanding and experimenting with these methods, you can enhance your string manipulation skills and efficiently handle string operations in your JavaScript projects. Happy coding!