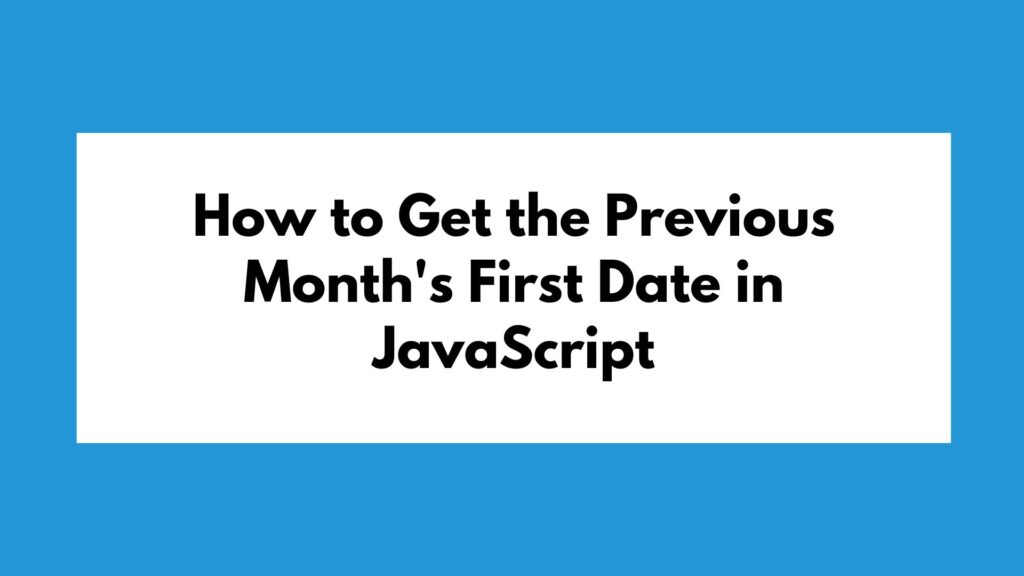
In the world of JavaScript programming, there are often tasks that require manipulating dates. One common task is finding the first date of the previous month. In this article, we will explore various methods to achieve this using JavaScript. By the end of this guide, you will have a clear understanding of how to get the previous month’s first date in JavaScript.
Methods to Get the Previous Month’s First Date in JavaScript
Method 1: Using JavaScript Date Object
One straightforward way to get the previous month’s first date is by utilizing the JavaScript Date object. Here’s a step-by-step breakdown of this method:
// Get the current date let currentDate = new Date(); // Set the date to the first day of the current month currentDate.setDate(1); // Subtract a day to go back to the last day of the previous month currentDate.setDate(0); // Get the first day of the previous month let firstDayOfPreviousMonth = new Date(currentDate.getFullYear(), currentDate.getMonth(), 1); console.log(firstDayOfPreviousMonth);
Method 2: Using Moment.js Library
Another approach is to use the Moment.js library, which provides powerful date manipulation functions. Here’s how you can achieve this using Moment.js:
// Install Moment.js library using npm or yarn // npm install moment // yarn add moment // Import Moment.js library const moment = require('moment'); // Get the first day of the previous month let firstDayOfPreviousMonth = moment().subtract(1, 'months').startOf('month'); console.log(firstDayOfPreviousMonth.format('YYYY-MM-DD'));
By following these methods, you can easily obtain the previous month’s first date in JavaScript for your programming needs. Remember to choose the method that best suits your project requirements and coding style.
Conclusion
In conclusion, mastering date manipulation in JavaScript is essential for any developer. Understanding how to retrieve specific dates, such as the first day of the previous month, can enhance the functionality and efficiency of your scripts. Keep experimenting and honing your skills to become a proficient JavaScript programmer.
Happy coding!