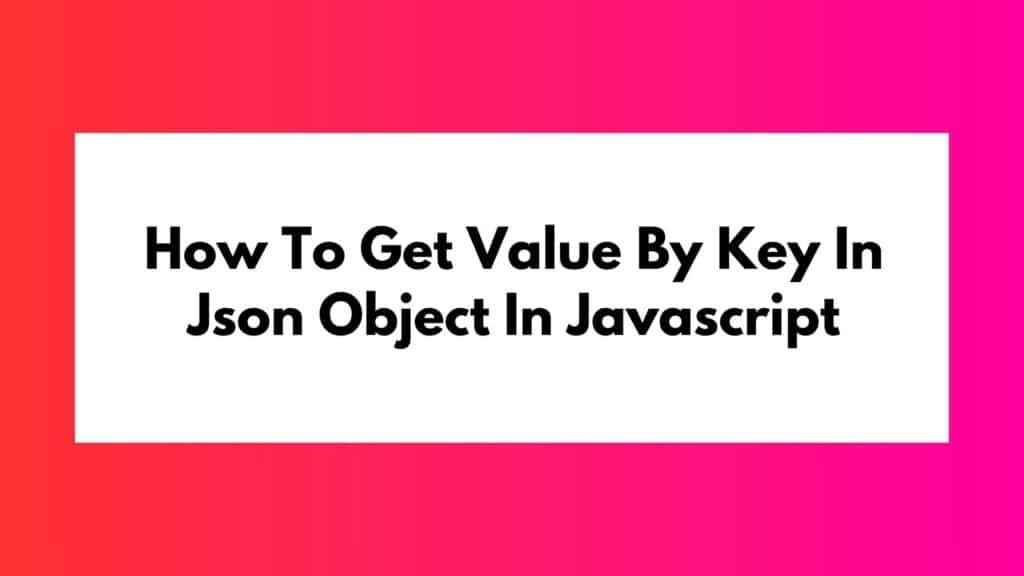
When working with JavaScript, dealing with JSON objects is a common task. JSON (JavaScript Object Notation) is a lightweight data-interchange format that is easy for humans to read and write and easy for machines to parse and generate. One frequent operation when working with JSON objects in JavaScript is retrieving a value by its key. In this article, we will delve into various methods to achieve this efficiently.
To Get Value By Key In Json Object In Javascript, We Can Follow These Methods:
Method 1: Using Dot Notation
One straightforward way to access a value in a JSON object is through dot notation. If you know the key name, you can directly access the value associated with it. Here’s an example:
const jsonObject = { key1: 'value1', key2: 'value2' }; const value = jsonObject.key1; console.log(value); // Output: value1
Method 2: Using Bracket Notation
Another method to retrieve a value from a JSON object is by using bracket notation. This method is useful when the key is dynamic or stored in a variable.
const jsonObject = { key1: 'value1', key2: 'value2' }; const key = 'key2'; const value = jsonObject[key]; console.log(value); // Output: value2
Method 3: Using JSON.parse()
If you have a JSON string and want to extract a value by key, you can first parse the JSON string using JSON.parse()
and then access the value as shown below:
const jsonString = '{"key1": "value1", "key2": "value2"}'; const jsonObject = JSON.parse(jsonString); const value = jsonObject.key1; console.log(value); // Output: value1
Method 4: Using Object Destructuring
Object destructuring can be a concise way to extract values from a JSON object by key.
const jsonObject = { key1: 'value1', key2: 'value2' }; const { key1, key2 } = jsonObject; console.log(key1); // Output: value1 console.log(key2); // Output: value2
Conclusion
In conclusion, accessing values by keys in a JSON object in JavaScript is a fundamental operation. By understanding the various methods available, you can efficiently retrieve the desired data from JSON structures. Whether using dot notation, bracket notation, JSON.parse()
, or object destructuring, JavaScript provides flexible ways to work with JSON objects and extract values based on keys.