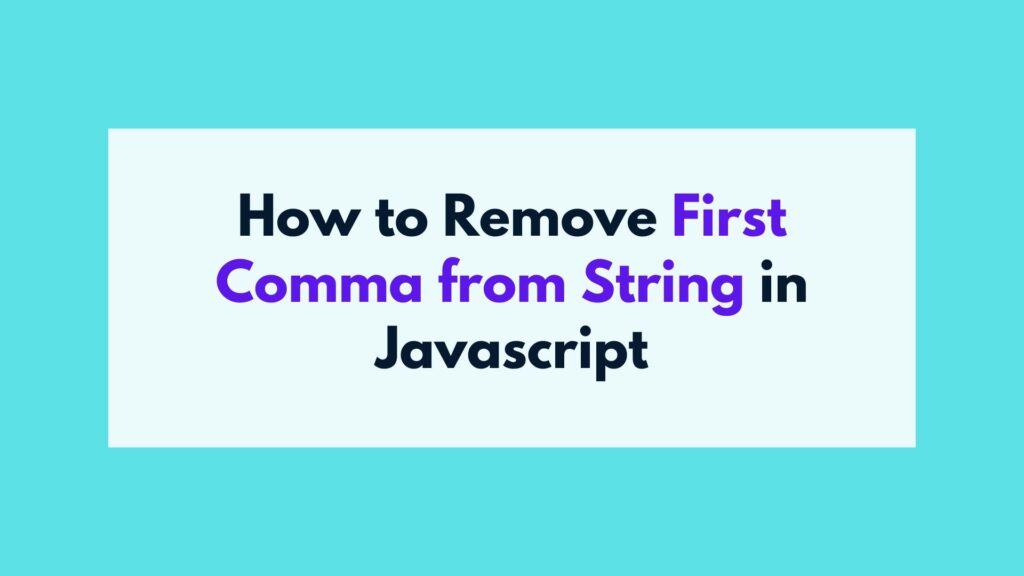
Have you ever encountered a situation where you needed to remove the first comma from a string in JavaScript? It may seem like a simple task, but there are a few considerations to keep in mind. In this article, we will explore various approaches to achieve this goal while maintaining code readability and efficiency.
How to Remove First Comma from String in Javascript
The Exact Solution
To remove the first comma from a string in JavaScript, we can make use of the replace()
method along with a regular expression. Here’s an example that demonstrates how it can be done:
let str = "Hello, World, how, are, you?"; let result = str.replace(/,/, ''); console.log(result); // Output: "Hello World, how, are, you?"
By using the replace()
method with the regular expression /,/
, we can replace the first occurrence of a comma with an empty string. This effectively removes the first comma from the given string.
Exploring Different Approaches
Now, let’s delve deeper into different approaches to removing the first comma from a string in JavaScript.
1) Using the indexOf
and substring
Methods
One way to achieve this is by utilizing the indexOf()
and substring()
methods. Here’s an example that illustrates this approach:
let str = "Hello, World, how, are, you?"; let index = str.indexOf(','); let result = str.substring(0, index) + str.substring(index + 1); console.log(result); // Output: "Hello World, how, are, you?"
In this approach, we first find the index of the first comma using the indexOf()
method. Then we concatenate the substring before the comma (str.substring(0, index)
) with the substring after the comma (str.substring(index + 1)
), effectively removing the first comma.
2. Splitting and Joining
Another approach is to split the string into an array using the split()
method, remove the unwanted element (in this case, the first element), and then join the array back into a string using the join()
method. Here’s an example:
let str = "Hello, World, how, are, you?"; let arr = str.split(','); arr.shift(); // Remove the first element let result = arr.join(','); console.log(result); // Output: "Hello World, how, are, you?"
In this approach, we split the string into an array using split(',')
. Then we use shift()
to remove the first element from the array. Finally, we join the array back into a string using join(',')
, effectively removing the first comma.
3. Regular Expressions
As mentioned earlier, we can also utilize regular expressions to achieve our goal. Here’s another example that demonstrates this approach:
let str = "Hello, World, how, are, you?"; let result = str.replace(/^([^,]+),/, '$1'); console.log(result); // Output: "Hello World, how, are, you?"
In this approach, we use a regular expression that matches everything before the first comma (/^([^,]+),/
). By capturing everything before the comma using parentheses (([^,]+)
), we can refer to it as $1
in the replacement string. This effectively removes the first comma from the given string.
Examples and Explanation
Let’s explore a few more examples to further understand how to remove the first comma from a string in JavaScript.
Example 1:
let str = ",Hello World"; let result = str.replace(/^(,+)/, ''); console.log(result); // Output: "Hello World"
In this example, we have a string that starts with one or more commas. The regular expression /^(,+)/
matches and captures these leading commas. By replacing them with an empty string (''
), we effectively remove them from the resulting string.
Example 2:
let str = "One,two"; let result = str.replace(/^(.+?),/, '$1'); console.log(result); // Output: "One,two"
In this example, we have a string with only one comma. The regular expression /^(.+?),/
matches and captures everything before the first comma. By referring to the captured group as $1
in the replacement string, we keep it as is and effectively remove only the first comma.
Conclusion
Removing the first comma from a string in JavaScript may seem like a simple task at first glance. However, by exploring different approaches and understanding their implications, we can ensure code readability and efficiency. In this article, we covered several methods including using the replace()
method with regular expressions, utilizing indexOf()
and substring()
, as well as splitting and joining arrays. By applying these techniques in your JavaScript projects, you can confidently handle scenarios where removing the first comma from a string is required.
Remember to always consider your specific use case and choose the approach that best suits your needs. Happy coding!