To remove the last comma from a string in JavaScript, you can use the slice()
, substring()
, or replace()
methods. Here’s a code snippet that demonstrates the slice()
method:
let str = "Hello, World,"; if (str.charAt(str.length - 1) === ',') { str = str.slice(0, -1); } console.log(str); // Output: Hello, World
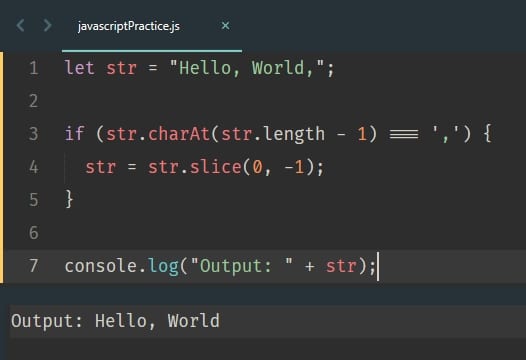
In JavaScript, there are various scenarios where you may need to remove the last comma from a string. Whether you are dealing with data manipulation or formatting, this article will guide you through the step-by-step process of accomplishing this task. By following the outlined coding guidelines and examples provided below, you will be able to remove the last comma from a string in JavaScript effectively.
To remove the last comma from a string in JavaScript, you can follow these simple steps:
Step 1: Identify the String
First, you need to identify the string from which you want to remove the last comma. This can be a variable or a hardcoded string.
Step 2: Check if the Last Character is a Comma
Next, you should check if the last character of the string is a comma. This can be done using the charAt()
method or by accessing the last character using index notation.
let str = "Hello, World,"; if (str.charAt(str.length - 1) === ',') { // Code to remove the last comma }
Step 3: Remove the Last Comma
If the last character is indeed a comma, you can proceed to remove it. There are multiple approaches to achieve this:
Approach 1: Using slice()
method
The slice()
method can be used to extract a portion of the string, excluding the last character.
let str = "Hello, World,"; if (str.charAt(str.length - 1) === ',') { str = str.slice(0, -1); } console.log(str); // Output: Hello, World
Approach 2: Using substring()
method
The substring()
method allows you to extract a substring from a string. By specifying the start and end indices, you can remove the last character.
let str = "Hello, World,"; if (str.charAt(str.length - 1) === ',') { str = str.substring(0, str.length - 1); } console.log(str); // Output: Hello, World
Approach 3: Using Regular Expressions
Regular expressions provide a powerful way to manipulate strings. You can use the replace()
method along with a regular expression pattern to remove the last comma.
let str = "Hello, World,"; str = str.replace(/,$/, ''); console.log(str); // Output: Hello, World
Example Code Snippets
Here are some additional example code snippets that demonstrate different scenarios and variations of removing the last comma from a string in JavaScript:
Example 1: Removing the Last Comma from Multiple Strings
let str1 = "Apple,"; let str2 = "Banana,"; let str3 = "Orange,"; let strings = [str1, str2, str3]; for (let i = 0; i < strings.length; i++) { if (strings[i].charAt(strings[i].length - 1) === ',') { strings[i] = strings[i].slice(0, -1); } } console.log(strings); // Output: ["Apple", "Banana", "Orange"]
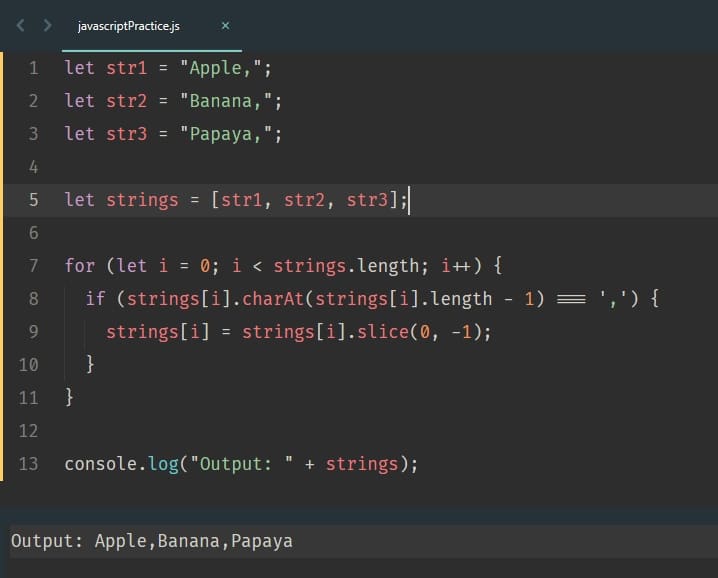
Example 2: Removing the Last Comma and Other Punctuation Marks
let str = "Hello, World!"; str = str.replace(/[\,\!\.\?]+$/, ''); console.log(str); // Output: "Hello World"
Conclusion
Removing the last comma from a string in JavaScript is a common task when dealing with data manipulation or formatting. By following the step-by-step coding guidelines and understanding the provided examples, you now have the knowledge to effectively remove the last comma from any given string. Remember to choose the approach that best suits your specific requirements. Happy coding!