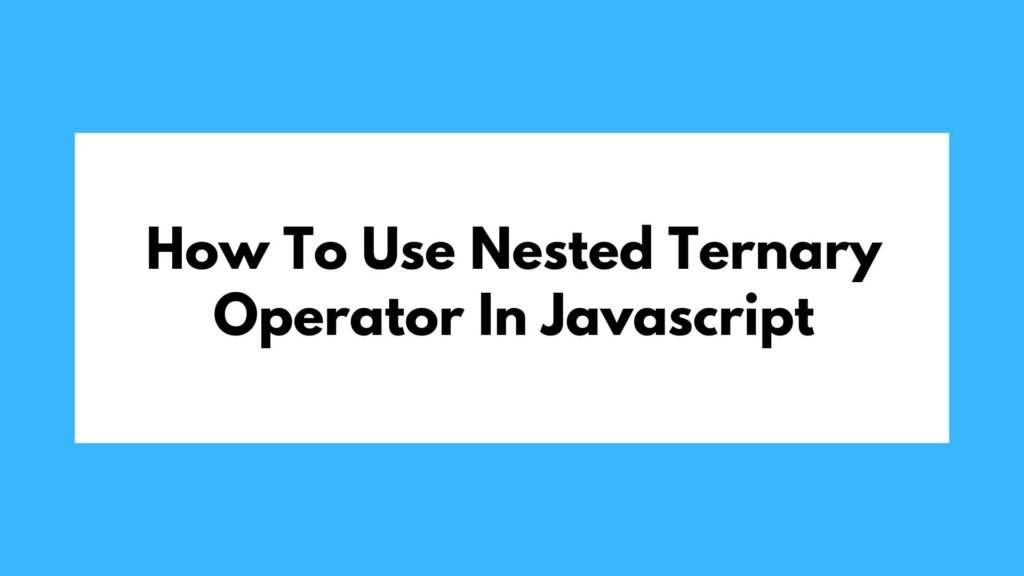
When it comes to JavaScript programming, nested ternary operators can be a powerful tool in writing concise and efficient code. In this article, we will delve into the concept of nested ternary operators, providing step-by-step explanations and multiple examples to help you grasp this technique effectively.
Table of Contents
What are Ternary Operators?
Ternary operators, also known as conditional expressions, are compact expressions used to evaluate conditions. The basic syntax of a ternary operator in JavaScript is:
condition ? value if true : value if false
This operator evaluates the condition; if the condition is true, it returns the value after the ‘?’ symbol; otherwise, it returns the value after the ‘:’ symbol.
Nesting Ternary Operators
Nested ternary operators involve using one or more ternary operators inside another ternary operator. This nesting allows for more complex conditional logic in a single line of code. However, it is essential to maintain clarity and readability when using nested ternary operators.
Let’s consider an example of using nested ternary operators to determine the sign of a number:
const number = -10; const result = number > 0 ? "Positive" : number < 0 ? "Negative" : "Zero"; console.log(result); // Output: Negative
In this example, the nested ternary operators evaluate the sign of the number and provide the corresponding result.
Benefits of Nested Ternary Operators
Nested ternary operators can be beneficial in scenarios where you need to make multiple conditional checks in a concise manner. By nesting ternary operators, you can avoid writing lengthy if-else statements and achieve a more streamlined code structure.
Example: Nested Ternary Operator in Action
Let’s explore a practical example of using nested ternary operators to determine the type of a variable:
const variable = 42; const type = typeof variable === 'number' ? "Number" : typeof variable === 'string' ? "String" : "Other"; console.log(type); // Output: Number
In this example, the nested ternary operators check the type of the variable and assign the appropriate label.
Conclusion
In conclusion, nested ternary operators offer a concise way to handle multiple conditional checks in JavaScript. By understanding their usage and best practices, you can enhance your coding efficiency and readability. Experiment with nested ternary operators in your projects to experience their benefits firsthand.