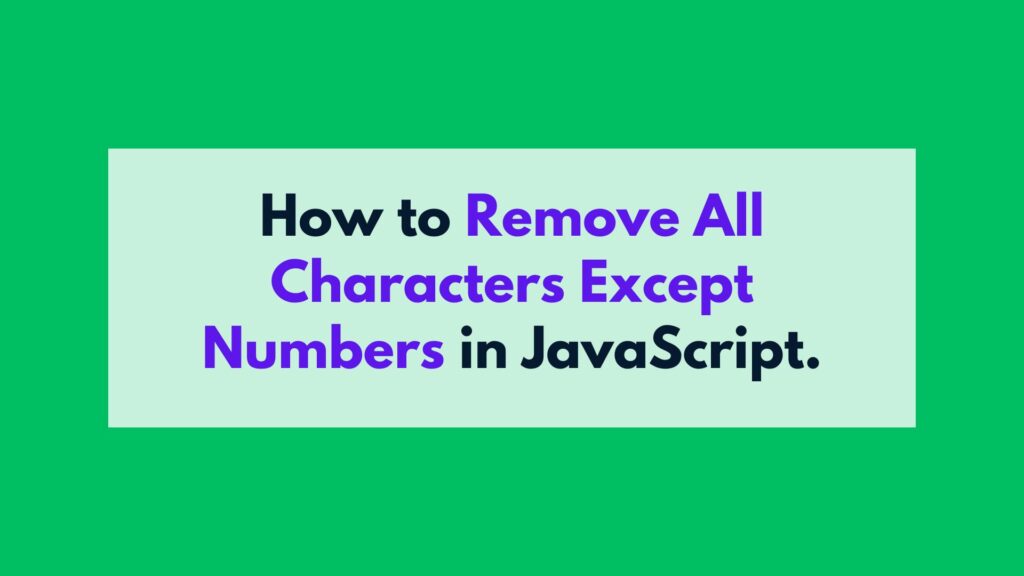
To remove all characters except numbers from a string in JavaScript, you can use regular expressions and the replace()
method. Here’s a quick example:
str = str.replace(/\D/g, '');
When working with strings in JavaScript, there may be occasions where you need to extract only the numerical values and remove any non-numeric characters. In this article, we will explore a simple and efficient method to achieve this using regular expressions and the replace()
method. By following the steps outlined below, you’ll be able to easily remove all characters except numbers in JavaScript.
Table of Contents
Step 1: Understanding Regular Expressions
Before we proceed, let’s take a moment to understand regular expressions. Regular expressions, often referred to as regex, are powerful patterns used to match and manipulate text. In our case, we’ll use regex to identify and remove non-numeric characters from a string.
Step 2: Using the replace()
Method
The replace()
method in JavaScript allows us to replace specific characters or patterns within a string. By combining this method with regular expressions, we can easily remove unwanted characters.
To remove all characters except numbers from a string, we can use the following code snippet:
str = str.replace(/\D/g, '');
Let’s break down this code:
- The regular expression
/ \D /g
matches all non-digit characters. - The
g
flag ensures that the replacement is applied globally throughout the string.
By replacing all non-digit characters with an empty string, we effectively remove them from the original string.
Examples
Let’s take a look at some examples to illustrate how this method works:
Example 1:
let str = "I have 123 apples!"; str = str.replace(/\D/g, ''); console.log(str); // Output: 123
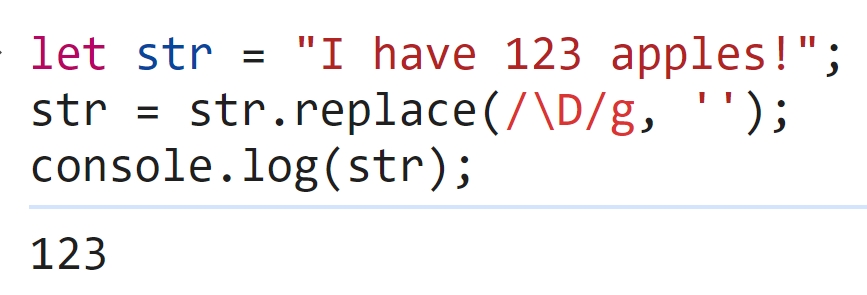
Example 2:
let str = "The price is $19.99"; str = str.replace(/\D/g, ''); console.log(str); // Output: 1999
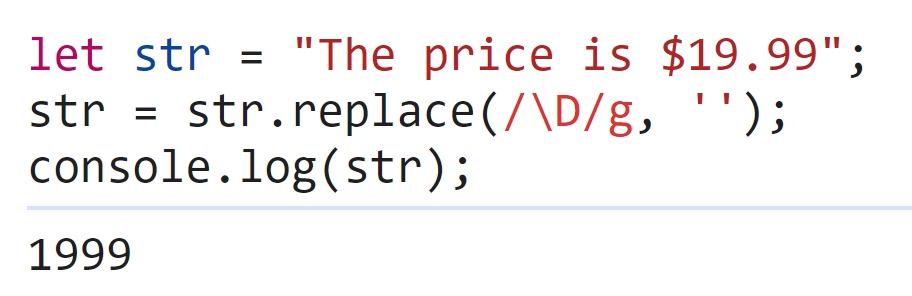
Example 3:
let str = "Phone: +1 (555) 123-4567"; str = str.replace(/\D/g, ''); console.log(str); // Output: 15551234567
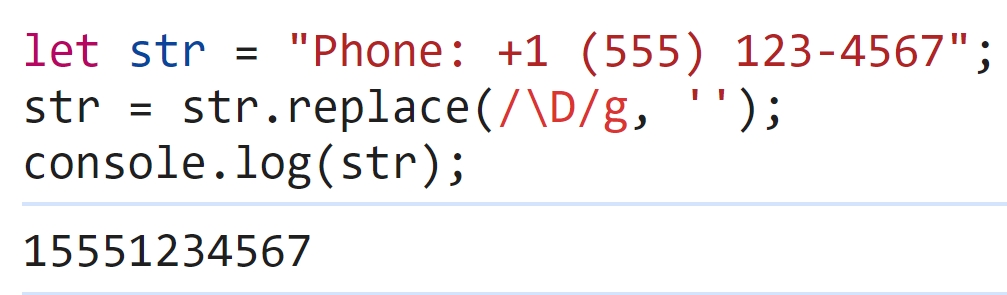
By applying the replace()
method with the regular expression / \D /g
, we remove all non-numeric characters from the given strings.
Conclusion
In this article, we have explored a simple yet effective way to remove all characters except numbers from a string in JavaScript. By utilizing regular expressions with the replace()
method, we can easily achieve this task. Remember to always test your code and consider edge cases when applying this technique. Now you can confidently extract numerical values from strings in your JavaScript projects.