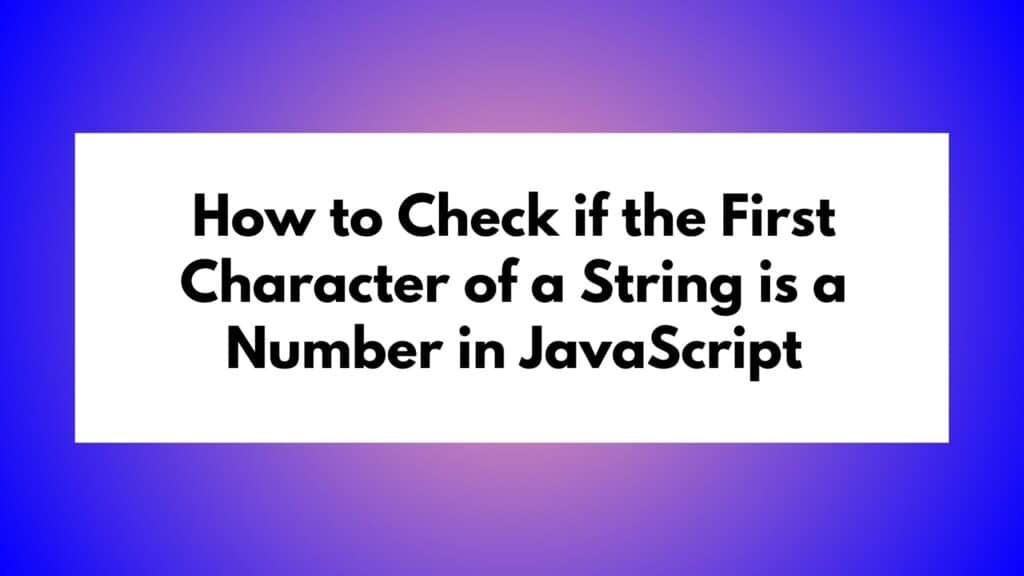
To check if a string starts with a number in JavaScript, you can use the charAt()
method to extract the first character and then apply the isNaN()
function to validate if it is not a number. Here’s a code snippet that demonstrates this:
function isFirstCharNumber(str) { const firstChar = str.charAt(0); return !isNaN(firstChar); } const exampleString = "7Hello"; console.log(isFirstCharNumber(exampleString));
In JavaScript, it is often necessary to determine whether the first character of a given string is a number. This can be useful in various scenarios, such as form validation or data processing. In this article, we will explore step-by-step coding techniques to achieve this using JavaScript.
To check if the first character of a string is a number in javascript we can follow these steps:
Step 1: Extracting the First Character
The first step in determining whether the first character of a string is a number is to extract that character. We can achieve this by using the charAt()
method in JavaScript. Let’s see an example:
const str = "7Hello"; const firstChar = str.charAt(0); console.log(firstChar); // Output: 7
In the above code snippet, we declare a variable str
which holds the string we want to check. We then use the charAt()
method to extract the first character, which is assigned to the variable firstChar
. Finally, we log the value of firstChar
to the console.
Step 2: Checking if the First Character is a Number
Once we have extracted the first character from the string, we can proceed to check if it is a number. JavaScript provides several approaches to achieve this. One simple way is by using the isNaN()
function, which stands for “is Not a Number”. Let’s see an example:
const str = "7Hello"; const firstChar = str.charAt(0); const isFirstCharNumber = !isNaN(firstChar); console.log(isFirstCharNumber); // Output: true
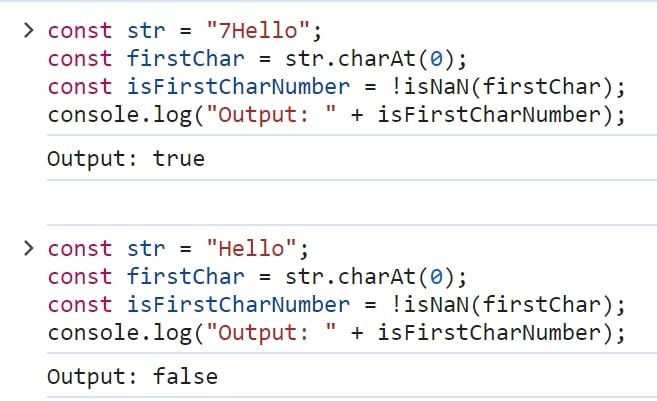
In the above code snippet, we use the isNaN()
function to check if firstChar
is not a number. The result is then assigned to the variable isFirstCharNumber
. Finally, we log the value of isFirstCharNumber
to the console.
Step 3: Putting it All Together
Now that we have seen how to extract the first character and check if it is a number, let’s combine these steps into a single function that can be easily reused. Here’s an example:
function isFirstCharNumber(str) { const firstChar = str.charAt(0); return !isNaN(firstChar); } const exampleString = "7Hello"; console.log(isFirstCharNumber(exampleString)); // Output: true
In the above code snippet, we define a function isFirstCharNumber()
that takes a string as an argument. Inside the function, we extract the first character and check if it is a number using the isNaN()
function. The result is then returned by the function. Finally, we call the function with an example string and log the result to the console.
Examples and Explanation
To further clarify the concept, let’s consider a few more examples:
Example 1: Checking a Numeric First Character
const str = "9Hello"; console.log(isFirstCharNumber(str)); // Output: true
In this example, the string "9Hello"
is passed to our isFirstCharNumber()
function. Since the first character is a number, the output will be true
.
Example 2: Checking an Alphabetic First Character
const str = "Hello"; console.log(isFirstCharNumber(str)); // Output: false
In this example, the string "Hello"
is passed to our isFirstCharNumber()
function. As the first character is not a number, the output will be false
.
Example 3: Checking an Empty String
const str = ""; console.log(isFirstCharNumber(str)); // Output: false
In this example, an empty string is passed to our isFirstCharNumber()
function. Since there is no first character present, the output will be false
.
By following these examples and explanations, you should now have a solid understanding of how to check if the first character of a string is a number in JavaScript.
Conclusion
In JavaScript, being able to determine whether the first character of a string is a number can be crucial for various programming tasks. By utilizing the charAt()
method and checking against isNaN()
, you can easily accomplish this task. Remember to extract the first character using charAt()
and then apply the isNaN()
function to validate if it is not a number. By following these step-by-step instructions and studying the provided examples, you should now have a comprehensive understanding of how to check if the first character of a string is a number in JavaScript.