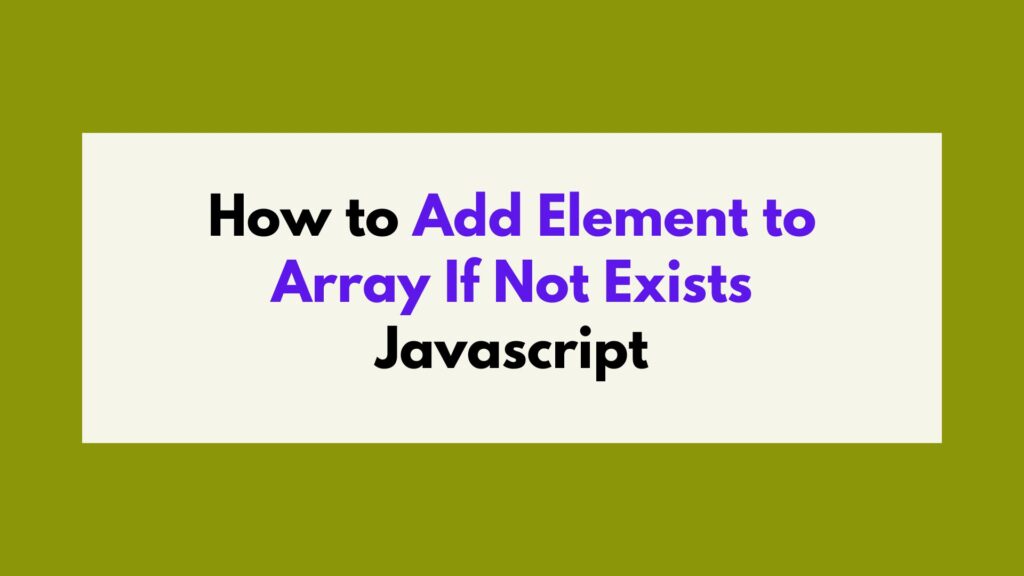
In this article, we will explore how to add an element to an array in JavaScript only if it doesn’t already exist. We’ll cover different methods and provide detailed examples for a better understanding.
When working with arrays in JavaScript, there may be times when you need to add an element to the array only if it doesn’t already exist. This can be a common requirement when dealing with data manipulation or filtering. In this article, we will discuss various approaches to achieve this functionality and provide practical examples to illustrate each method.
Table of Contents
Understanding the Problem
Before diving into the solutions, let’s understand the problem at hand. We want to add an element to an array, but only if that element doesn’t already exist within the array. This ensures that we avoid duplicate entries and maintain data integrity.
Method 1: Using the includes()
Method
One approach to solving this problem is by utilizing the includes()
method provided by JavaScript arrays. This method checks whether an element is present in an array and returns a boolean value. Let’s see how it works with an example:
const array = [1, 2, 3, 4, 5]; const elementToAdd = 3; if (!array.includes(elementToAdd)) { array.push(elementToAdd); } console.log(array); // Output: [1, 2, 3, 4, 5]
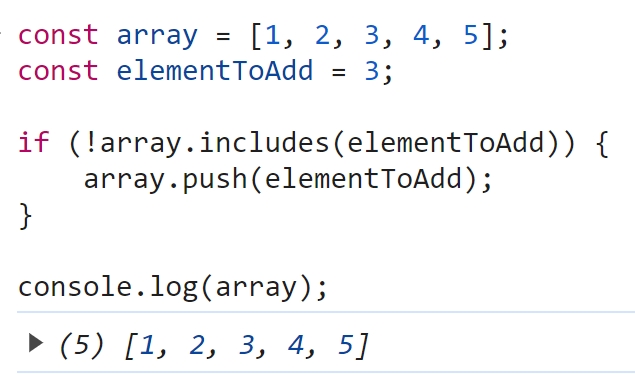
In this example, we check if the elementToAdd
already exists in the array
using includes()
. If it doesn’t exist, we add it using push()
. As a result, the array remains unchanged as 3
is already present.
Method 2: Utilizing the indexOf()
Method
Another approach is to utilize the indexOf()
method, which returns the first index at which a given element can be found in the array. If the element is not found, -1
is returned. Here’s an example:
const array = ['apple', 'banana', 'orange']; const elementToAdd = 'mango'; if (array.indexOf(elementToAdd) === -1) { array.push(elementToAdd); } console.log(array); // Output: ['apple', 'banana', 'orange', 'mango']

In this case, we use indexOf()
to check if elementToAdd
exists in the array
. If the returned index is -1
, we know that the element doesn’t exist, so we proceed to add it using push()
.
Method 3: Leveraging ES6’s Set
Object
ES6 introduced the Set
object, which allows us to store unique values of any type. By converting our array into a Set
, we can easily add elements while ensuring uniqueness. Let’s see how it works:
let array = [10, 20, 30]; const elementToAdd = 20; array = [...new Set([...array, elementToAdd])]; console.log(array); // Output: [10, 20, 30]

In this example, we create a Set
from the existing array
, add the desired elementToAdd
, and then convert it back into an array using the spread operator (...
). The resulting array will only contain unique elements.
Conclusion
In this article, we explored different methods for adding elements to an array in JavaScript only if they don’t already exist. We covered techniques such as using the includes()
method, leveraging the indexOf()
method, and utilizing ES6’s Set
object.
By applying these approaches, you can handle scenarios where you need to maintain uniqueness within your arrays while adding new elements. Remember to choose the method that best suits your specific use case.
By employing these techniques, you’ll have a solid foundation for manipulating arrays and adding elements efficiently in JavaScript.
Remember to keep practicing and experimenting with different scenarios to enhance your understanding of JavaScript arrays and their manipulation possibilities.
Happy coding!