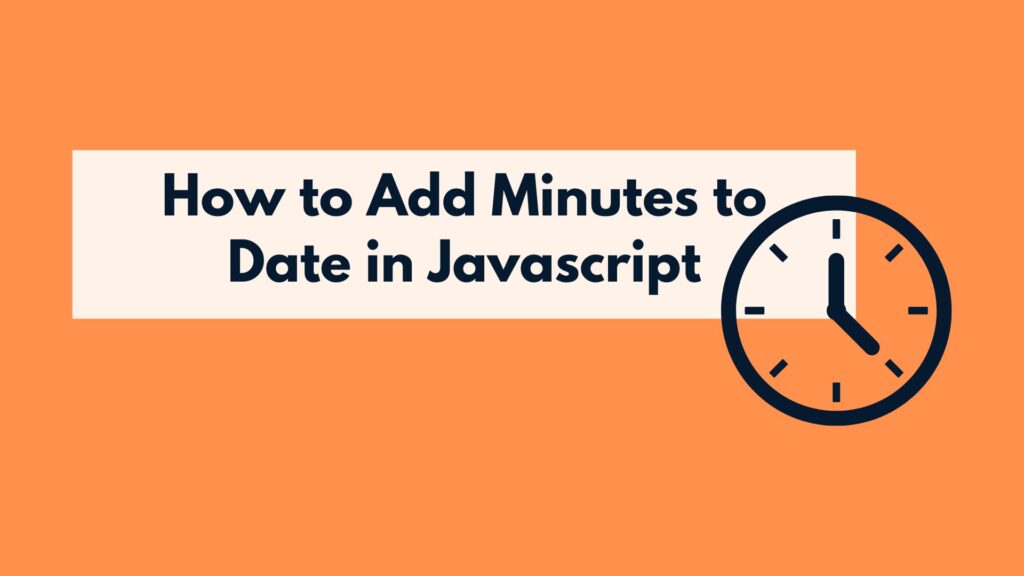
In JavaScript, working with dates and time is a common task. One specific requirement that often arises is the need to add minutes to a given date. This article will provide you with a step-by-step guide on how to achieve this using JavaScript. We will explore various examples and explain each one in detail. So, let’s dive in!
To add minutes to a date in JavaScript, you can use the setMinutes()
method to modify the minute value of a Date object. Here’s a quick example:
const currentDate = new Date(); currentDate.setMinutes(currentDate.getMinutes() + 30);
To add minutes to a date, we need to follow a few simple steps:
Step 1: Create a Date Object
First, we need to create a Date object that represents the initial date and time. We can use the new Date()
constructor to achieve this. Let’s consider an example where we want to add 10 minutes to the current date:
const currentDate = new Date();
Step 2: Modify the Minutes
Next, we can use the setMinutes()
method to modify the minute value of our Date object. This method takes an integer as an argument, representing the new value for the minutes. By adding the desired number of minutes to the existing minute value, we can effectively add minutes to the date. For instance, if we want to add 10 minutes:
currentDate.setMinutes(currentDate.getMinutes() + 10);
Step 3: Obtain the Updated Date
After modifying the minutes, we can obtain the updated date by accessing the modified Date object. The updated date will reflect the added minutes. You can use this updated date for further calculations or display purposes.
const updatedDate = currentDate;
Examples
Now, let’s explore some examples to solidify our understanding.
Example 1: Adding 15 Minutes
Suppose we have a scenario where we need to add 15 minutes to the current date. Here’s how you can achieve it:
const currentDate = new Date(); currentDate.setMinutes(currentDate.getMinutes() + 15); const updatedDate = currentDate;
In this example, we create a Date object representing the current date and time. Then, we use setMinutes()
to add 15 minutes. Finally, we obtain the updated date.
Example 2: Adding Custom Minutes
Let’s consider a case where we want to add a custom number of minutes to a specific date and time. Here’s an example:
const customDate = new Date("2023-12-31T23:59:00"); customDate.setMinutes(customDate.getMinutes() + 45); const updatedDate = customDate;
In this example, we create a Date object with a specific date and time. Then, we add 45 minutes using setMinutes()
. The resulting updated date will reflect the addition of the specified minutes.
Conclusion
In this article, we have explored how to add minutes to a date in JavaScript. We learned to create a Date object, modify the minutes using setMinutes()
, and obtain the updated date. By following these steps, you can easily add minutes to any given date and time in your JavaScript applications.
Remember, working with dates and times is essential in many applications, so mastering these concepts will greatly enhance your JavaScript skills.