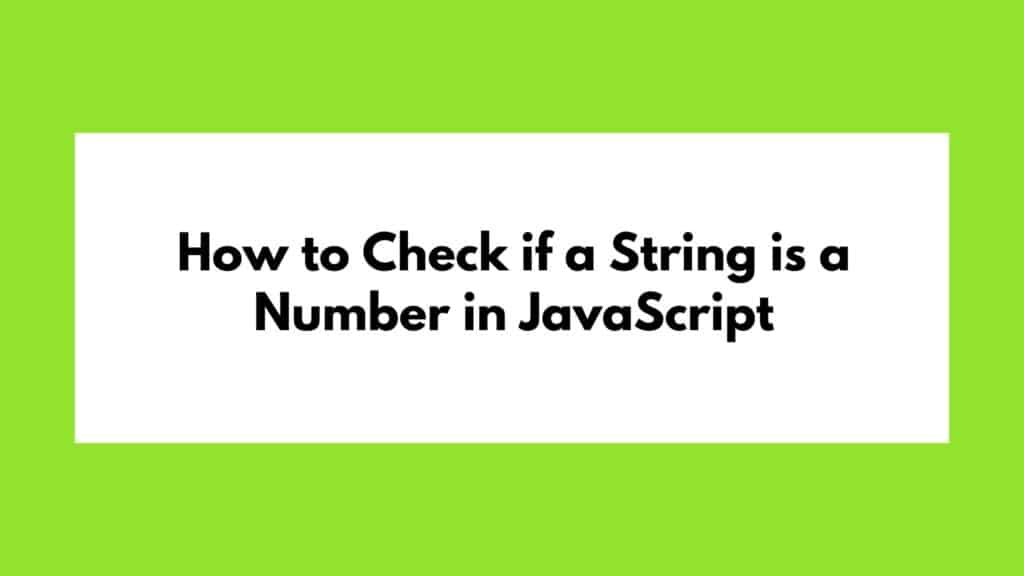
When working with JavaScript, it’s common to encounter scenarios where you need to determine whether a given string represents a numeric value. In this article, we will explore various methods to achieve this check effectively. Checking if a string is a number involves considering different aspects, such as handling edge cases and ensuring accuracy in validations.
To Check If A String Is A Number In Javascript We Can Follow These Methods:
Method 1: Using isNaN()
The isNaN()
function in JavaScript is a built-in method that returns true
if the argument provided is Not-A-Number. While this function can be helpful, it has some caveats when it comes to checking if a string is a valid number.
const isNumber = (value) => !isNaN(value); console.log(isNumber('123')); // true console.log(isNumber('hello')); // false
In the above code snippet, the isNumber
function utilizes isNaN()
to determine if the input value is a number. However, it’s essential to note that this method may not handle all cases accurately, especially when dealing with non-numeric strings.
Method 2: Regular Expression Approach
Another approach to validate if a string is a number is by using regular expressions. Regular expressions provide a flexible and robust way to match patterns within strings.
const isNumeric = (value) => /^\d+$/.test(value); console.log(isNumeric('123')); // true console.log(isNumeric('hello')); // false
In the above code snippet, the isNumeric
function uses a regular expression \d+
to match one or more digits in the input string. This method offers more precise control over what constitutes a valid numeric string.
Method 3: Parsing as Float
Parsing a string as a float using parseFloat()
is another technique to verify if it represents a number. This method is particularly useful when decimal values are involved.
const isFloat = (value) => !isNaN(parseFloat(value)); console.log(isFloat('123.45')); // true console.log(isFloat('hello')); // false
By utilizing parseFloat()
and checking for NaN
, we can ascertain if the input string can be successfully converted into a floating-point number.
Conclusion
In this article, we discussed various methods to check if a string is a number in JavaScript. Understanding these techniques will enable you to handle numeric validations effectively in your applications. Remember to consider edge cases and the specific requirements of your use case when implementing these checks.