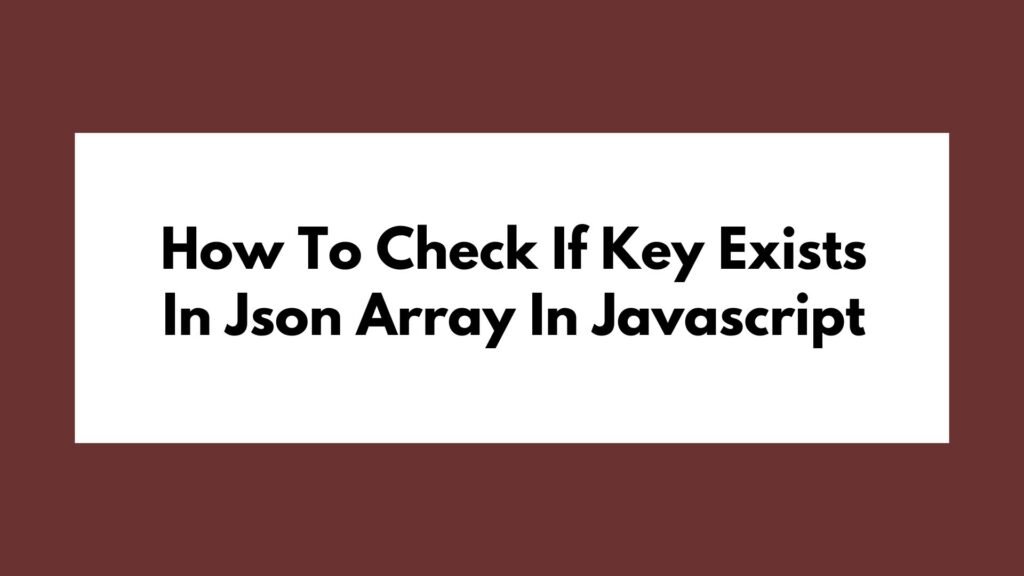
In JavaScript, working with JSON arrays is a common task. One frequent requirement is to check if a specific key exists within the JSON array. In this article, we will explore how to achieve this efficiently. Let’s dive into the details step by step.
Table of Contents
Understanding JSON Arrays
Before we proceed, let’s clarify what JSON arrays are. JSON (JavaScript Object Notation) is a lightweight data interchange format. An array in JSON is a collection of key-value pairs enclosed in curly braces {}
. Each element in the array is separated by a comma.
Checking for Key Existence
To check if a key exists in a JSON array, we can iterate through the array and examine each object for the presence of the desired key. Here’s a simple function that accomplishes this:
function isKeyInArray(array, key) { for (let obj of array) { if (obj.hasOwnProperty(key)) { return true; } } return false; }
In the isKeyInArray
function above, we iterate through each object in the array using a for...of
loop. We then use the hasOwnProperty
method to check if the object contains the specified key. If found, the function returns true
; otherwise, it returns false
.
Example Usage
Let’s illustrate the usage of the isKeyInArray
function with an example JSON array:
const jsonArray = [ { id: 1, name: 'Alice' }, { id: 2, age: 30 }, { id: 3, name: 'Bob', city: 'New York' } ]; console.log(isKeyInArray(jsonArray, 'name')); // Output: true console.log(isKeyInArray(jsonArray, 'city')); // Output: true console.log(isKeyInArray(jsonArray, 'email')); // Output: false
In the example above, we have a JSON array jsonArray
with three objects. We then use the isKeyInArray
function to check for the existence of keys 'name'
, 'city'
, and 'email'
.
Conclusion
Checking if a key exists in a JSON array in JavaScript is a fundamental operation when working with data structures. By following the simple approach outlined in this article, you can efficiently determine the presence of keys within JSON arrays. Remember to consider edge cases and tailor the function to suit your specific requirements.