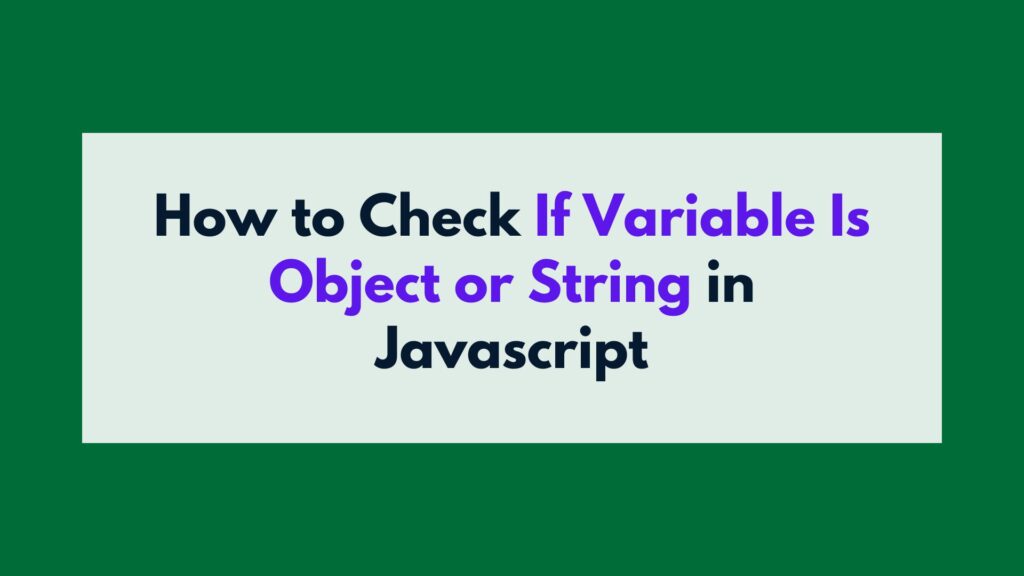
In JavaScript, it is often necessary to determine the type of a variable to perform appropriate operations or validations. One common scenario is checking whether a variable is an object or a string. In this article, we will explore various methods to achieve this in JavaScript.
To check if a variable is an object or a string in JavaScript, you can use the typeof
operator and the constructor
property. Additionally, the Object.prototype.toString.call()
method provides a reliable approach. We will demonstrate each method with examples.
Methods for Checking if a Variable is an Object or a String in JavaScript
Method 1: Using the typeof
Operator
The typeof
operator is commonly used to determine the primitive type of a variable. However, it also provides information about certain built-in objects. Let’s consider an example:
let variable = {}; // An object let type = typeof variable; console.log(type); // Output: "object"
Here, the typeof
operator returns “object” when applied to an object. However, it is important to note that this method does not differentiate between various object types.
Method 2: Using the constructor
Property
Another approach to check if a variable is an object or a string is by using the constructor
property. This property references the constructor function used to create an object. Consider the following example:
let variable = "Hello"; // A string let isString = variable.constructor === String; let isObject = variable.constructor === Object; console.log(isString); // Output: true console.log(isObject); // Output: false
In this example, we compare the constructor
property of the variable with the String
and Object
constructor functions. If the comparison returns true
, it means the variable is of that specific type.
Method 3: Using Object.prototype.toString.call()
The most reliable way to determine if a variable is an object or a string is by using the Object.prototype.toString.call()
method. This method provides a consistent output across different JavaScript environments. Let’s see an example:
let variable = "Hello"; // A string let type = Object.prototype.toString.call(variable); console.log(type); // Output: "[object String]"
By invoking toString.call()
on an object or a string, we obtain a string representation that includes its type enclosed within square brackets. This allows us to easily identify whether the variable is an object or a string.
Conclusion
In this article, we explored various methods for checking if a variable is an object or a string in JavaScript. We discussed using the typeof
operator, the constructor
property, and the Object.prototype.toString.call()
method. Each method provides an effective way to determine the type of a variable, but the latter two methods are more reliable in differentiating between objects and strings.
Remember to choose the appropriate method based on your specific requirements. Happy coding!