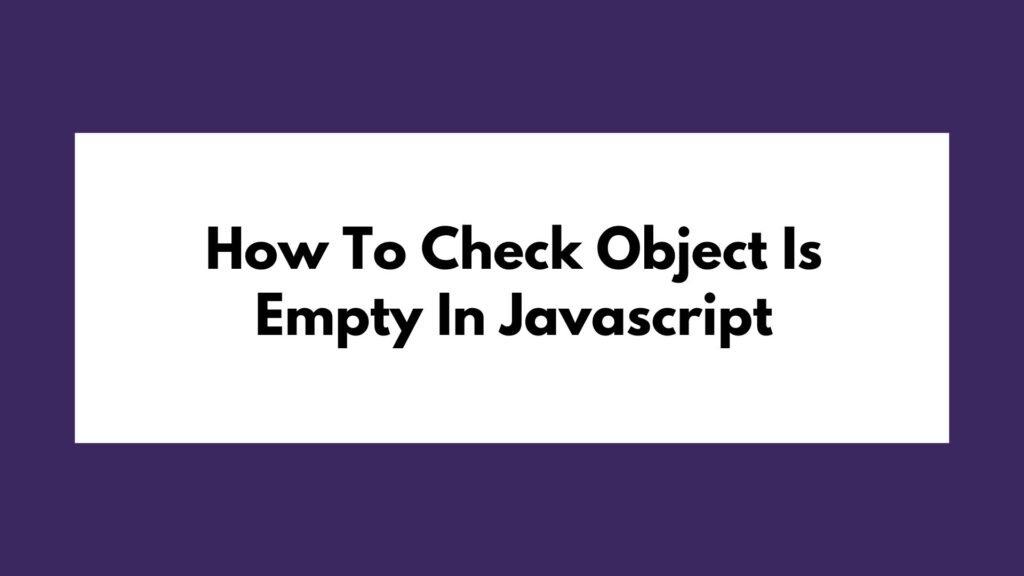
In JavaScript development, determining whether an object is empty is a common task that developers often encounter. This article will provide you with a step-by-step guide on how to check if an object is empty in JavaScript. Understanding this concept is crucial for writing efficient and error-free code.
Table of Contents
What is an Empty Object?
An empty object in JavaScript is an object that does not contain any properties. It means that the object has no key-value pairs defined within it.
Method 1: Using the Object.keys() Method
One of the simplest ways to check if an object is empty is by using the Object.keys()
method. This method returns an array of a given object’s own enumerable property names, which can then be used to determine if the object is empty.
Here’s a sample code snippet demonstrating this method:
const isEmpty = (obj) => { return Object.keys(obj).length === 0; }; // Example Usage const myObject = {}; console.log(isEmpty(myObject)); // Output: true
Method 2: Using a For…In Loop
Another approach to check for an empty object is by iterating over the object’s properties using a for...in
loop. By checking if the loop ever runs, we can determine if the object has any properties.
Let’s see how this method can be implemented:
const isEmpty = (obj) => { for (let key in obj) { if (obj.hasOwnProperty(key)) { return false; } } return true; }; // Example Usage const myObject = {}; console.log(isEmpty(myObject)); // Output: true
Conclusion
In this article, we’ve explored two methods to check if an object is empty in JavaScript. By utilizing the Object.keys()
method or a for...in
loop, you can efficiently determine whether an object contains any properties or not. Incorporating these techniques into your code will help you write more robust and reliable JavaScript applications.
Happy coding!