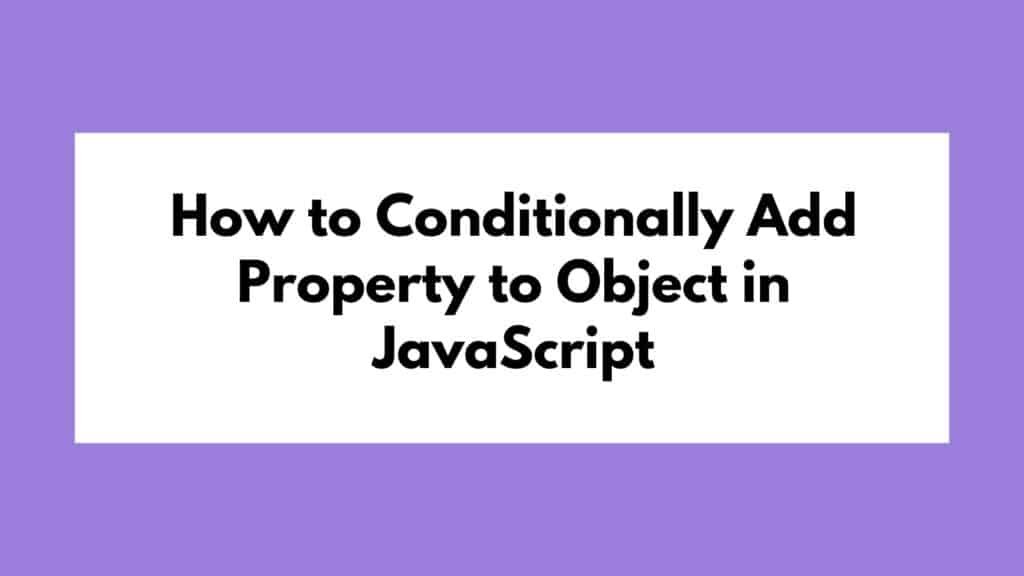
In JavaScript, the ability to conditionally add properties to objects is a powerful feature that allows developers to dynamically manipulate objects based on certain conditions. This article will walk you through the process of conditionally adding properties to objects in JavaScript, providing step-by-step explanations and multiple code examples for better understanding.
Understanding the Concept
Before diving into the implementation, let’s first grasp the basic idea. When we talk about conditionally adding a property to an object, it means that we want to add a new property to an object only if certain conditions are met. This approach helps keep our code concise and more efficient.
To Conditionally Add Property to Object in JavaScript We Can Follow These Methods:
Step 1: Create the Object
Let’s start by creating an object to which we will conditionally add properties based on specific conditions. For this example, we’ll use an object named person
:
let person = { name: 'Alice', age: 30 };
Step 2: Conditionally Add Property
Now, let’s see how we can conditionally add a new property to the person
object based on a certain condition. In this case, we will add the isAdmin
property if the person is over 18 years old:
if (person.age > 18) { person.isAdmin = true; }
In this code snippet, we check if the person’s age is greater than 18. If the condition is met, we add a new property isAdmin
to the person
object and set it to true
.
Step 3: Complete Example
To demonstrate the full implementation, let’s put all the steps together:
let person = { name: 'Alice', age: 30 }; if (person.age > 18) { person.isAdmin = true; } console.log(person);
When you run this code, you should see the updated person
object with the isAdmin
property added if the age condition is satisfied.
Conclusion
In conclusion, conditionally adding properties to objects in JavaScript provides a flexible way to manage object data based on specific criteria. By following the steps outlined in this article and experimenting with different conditions, you can enhance your JavaScript coding skills and create more dynamic applications.