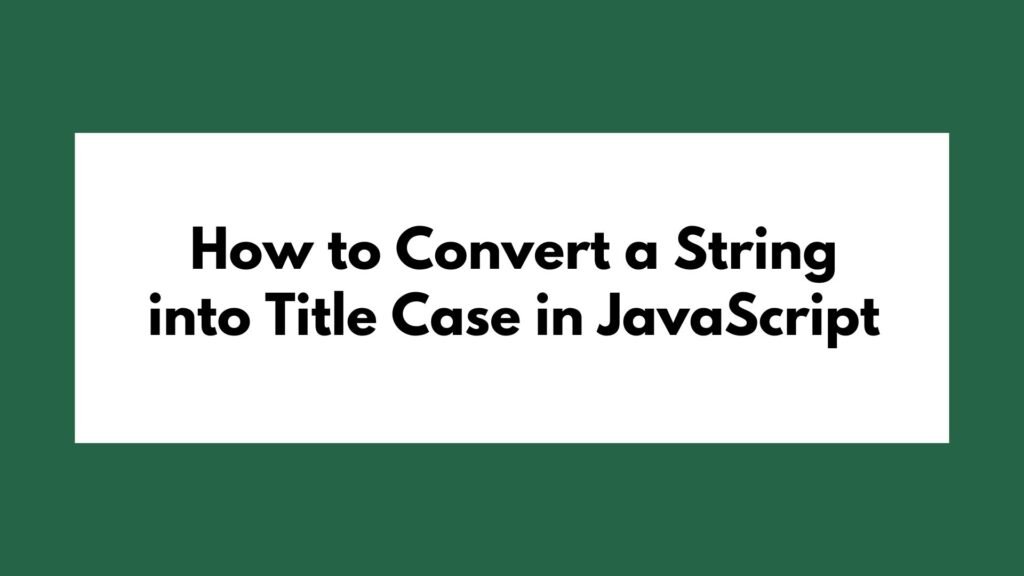
In the realm of programming tasks, converting a string into title case is a common requirement. This operation involves capitalizing the first letter of each word in a string. In this article, we will explore a concise and efficient way to achieve this using JavaScript.
Table of Contents
Understanding the Problem
Before we delve into the code implementation, let’s first grasp the concept of converting a string into title case. This process requires iterating through each word in the string and ensuring that the first letter of each word is capitalized while keeping the rest of the word in lowercase.
The Code Implementation
Let’s explore a simple JavaScript function that accomplishes the task of converting a string into title case:
function toTitleCase(str) { return str.toLowerCase().replace(/\b\w/g, function(char) { return char.toUpperCase(); }); } // Example Usage const inputString = "convert string to title case"; const titleCaseString = toTitleCase(inputString); console.log(titleCaseString); // Output: "Convert String To Title Case"
In the code snippet above:
- We define a function
toTitleCase
that takes a stringstr
as input. - We first convert the entire string to lowercase using
toLowerCase()
method. - We utilize a regular expression
/\b\w/g
to match the first character of each word. - The
replace()
method is employed to capitalize the matched characters.
Breaking Down the Code
- The
toLowerCase()
method ensures uniformity by converting all characters in the string to lowercase. - The regular expression
/\b\w/g
selectively targets the first character of each word. - By utilizing the
replace()
method with a callback function, we effectively capitalize each matched character.
Additional Examples
Let’s further solidify our understanding with additional examples:
Example 1:
console.log(toTitleCase("hello world")); // Output: "Hello World"
Example 2:
console.log(toTitleCase("javascript is awesome")); // Output: "Javascript Is Awesome"
Conclusion
In this comprehensive guide, we have explored how to convert a string into title case in JavaScript efficiently. By following the outlined approach, you can seamlessly implement this functionality in your projects. Remember to maintain code conciseness and readability for enhanced maintainability.