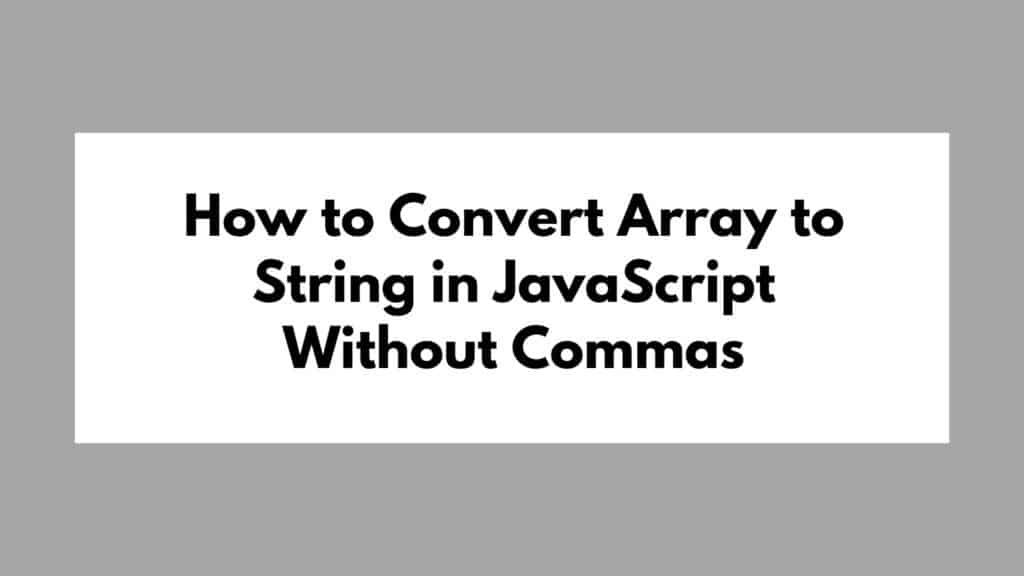
In the vast landscape of JavaScript programming, the need to convert an array to a string without commas arises frequently. This article aims to provide a concise yet comprehensive guide on achieving this task efficiently. We will explore various methods and custom functions to cater to different programming needs.
Table of Contents
Why Convert Array to String Without Commas?
Understanding the importance of converting an array to a string without commas is crucial in scenarios where a specific format is required. By eliminating commas, we can control the output format and enhance readability in our applications.
Method 1: Utilizing the join()
Method
The join()
method in JavaScript offers a straightforward solution for converting an array to a string without commas. By specifying a custom separator, we can achieve the desired output format.
const array = ['apple', 'banana', 'orange']; const stringWithoutCommas = array.join(' '); console.log(stringWithoutCommas);
In this example, we use a space character as the separator to concatenate the array elements into a string.
Method 2: Creating a Custom Function
For more flexibility and customization options, we can create a custom function that converts an array to a string without commas. This approach provides greater control over the output format.
function arrayToString(array, separator) { return array.reduce((acc, curr) => acc + separator + curr); } const fruits = ['apple', 'banana', 'orange']; const stringFormatted = arrayToString(fruits, ' | '); console.log(stringFormatted);
By defining our function, we can specify any desired separator, such as a pipe symbol (|
), to concatenate the array elements.
Conclusion
Converting an array to a string without commas in JavaScript is a versatile operation that can be achieved through different methods. Whether using built-in functions like join()
or creating custom functions for specific formatting needs, mastering this skill enhances data manipulation capabilities in JavaScript.