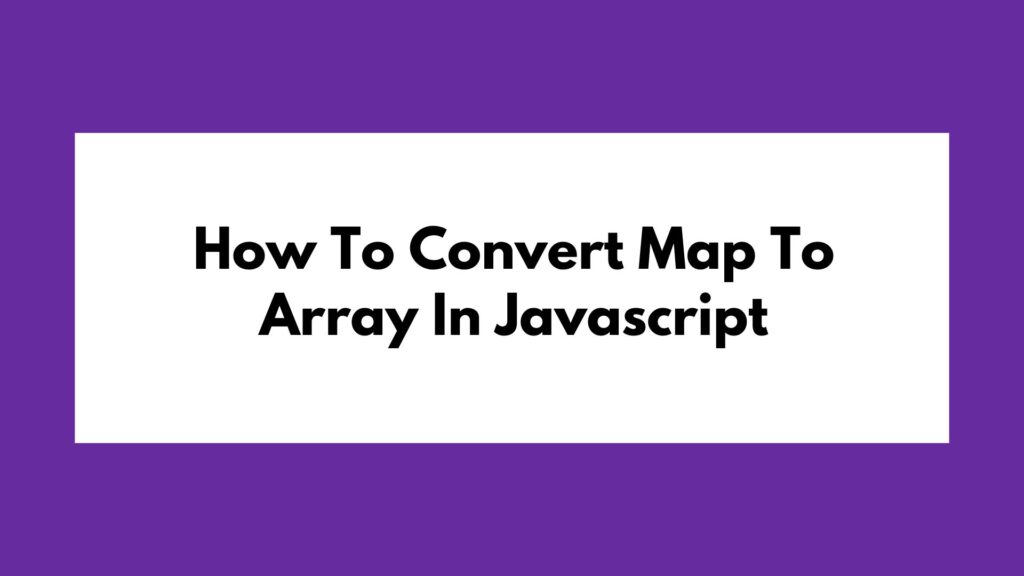
In JavaScript, converting a Map to an Array can be a useful operation when you need to work with the data in a different format. This guide will walk you through the process step by step, providing clear explanations and multiple code examples to help you understand and implement this conversion effectively.
Understanding Maps and Arrays in JavaScript
Before we dive into the conversion process, let’s briefly discuss Maps and Arrays in JavaScript.
Maps
A Map object in JavaScript holds key-value pairs and remembers the original insertion order of the keys. It allows both objects and primitive values as keys and values.
Arrays
Arrays are ordered collections of elements that allow storing multiple values under a single variable. Each element in an array has a numeric index.
Converting a Map to an Array
To convert a Map to an Array in JavaScript, we need to iterate over the Map and extract its key-value pairs into an array format. Here’s how you can achieve this:
// Create a new Map let myMap = new Map(); myMap.set('key1', 'value1'); myMap.set('key2', 'value2'); // Convert Map to Array let myArray = Array.from(myMap);
Code Explanation
- Creating a Map: We start by creating a new Map using the
new Map()
constructor. - Setting Key-Value Pairs: We add key-value pairs to the Map using the
set()
method. - Converting to Array: The
Array.from(myMap)
method converts the Map into an Array.
Example Usage
Let’s consider a more detailed example to illustrate the conversion process further:
let myMap = new Map(); myMap.set('name', 'John'); myMap.set('age', 30); myMap.set('country', 'USA'); let myArray = Array.from(myMap); console.log(myArray);
Breakdown
In this example:
- We create a Map
myMap
with key-value pairs representing a person’s information. - We convert the Map into an Array
myArray
usingArray.from()
. - Finally, we log the Array to the console, which will display
[["name", "John"], ["age", 30], ["country", "USA"]]
.
By following these steps, you can effectively convert a Map to an Array in JavaScript.
Conclusion
Converting a Map to an Array in JavaScript is a straightforward process that involves iterating over the Map and extracting its key-value pairs into an array format. By understanding the concepts behind Maps and Arrays, as well as following the provided code examples and explanations, you can seamlessly perform this conversion in your JavaScript projects.