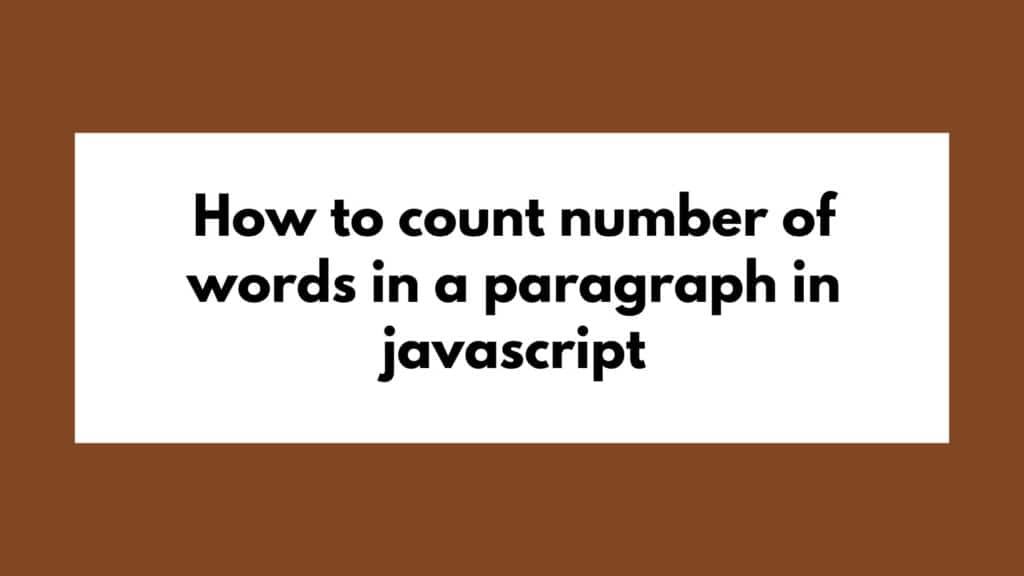
In the realm of web development, the ability to count the number of words in a paragraph using JavaScript is a fundamental skill. This task may seem simple on the surface, but it involves various considerations and techniques to ensure accurate results. In this article, we will delve into step-by-step coding guidelines, provide multiple example code snippets, and explain each in detail to help you master this essential concept.
To count number of words in a paragraph in javascript we can follow these methods:
Understanding the Problem
Before we dive into the coding aspects, let’s first understand the problem at hand. Counting the number of words in a paragraph involves parsing the text, identifying word boundaries, and handling various edge cases such as punctuation marks, special characters, and white spaces. By breaking down the paragraph into individual words, we can accurately determine the word count.
1. Using Regular Expressions
One of the most efficient ways to count words in a paragraph is by leveraging regular expressions. Regular expressions allow us to define patterns for matching words within the text. Let’s consider an example snippet using regular expressions:
const paragraph = "Lorem ipsum dolor sit amet, consectetur adipiscing elit."; const wordCount = paragraph.match(/\w+/g).length; console.log(wordCount);
Explanation:
\w+
matches one or more word characters (alphanumeric or underscore).- The
.match()
method returns an array of matched words. - Finally, we retrieve the length of the array to obtain the word count.
2. Handling Edge Cases
It’s essential to consider edge cases to ensure accurate word counting. For instance, dealing with hyphenated words or contractions requires special attention. Let’s enhance our code snippet to handle such cases:
const paragraph = "Word-counting can be challenging, isn't it?"; const wordCount = paragraph.match(/\b\w+\b/g).length; console.log(wordCount);
Explanation:
\b
denotes a word boundary, ensuring accurate word extraction.- Including
\b
at the beginning and end of the pattern captures complete words.
3. Implementing a Word Count Function
To encapsulate the word counting logic into a reusable function, we can define a function like this:
function countWords(paragraph) { return paragraph.match(/\b\w+\b/g).length; } const text = "Counting words in JavaScript is fascinating!"; const wordCount = countWords(text); console.log(wordCount);
Explanation:
- The
countWords
function takes a paragraph as input and returns the word count. - By encapsulating the logic in a function, we promote code reusability and maintainability.
For a more advanced word analysis tool, you might consider utilizing a free word frequency counter that offers additional features beyond basic word counting.
Conclusion
In conclusion, mastering the art of counting words in a paragraph using JavaScript requires an understanding of regular expressions, handling edge cases, and structuring reusable functions. By following the step-by-step guidelines and exploring example code snippets provided in this article, you are well-equipped to tackle this task effectively in your web development projects.