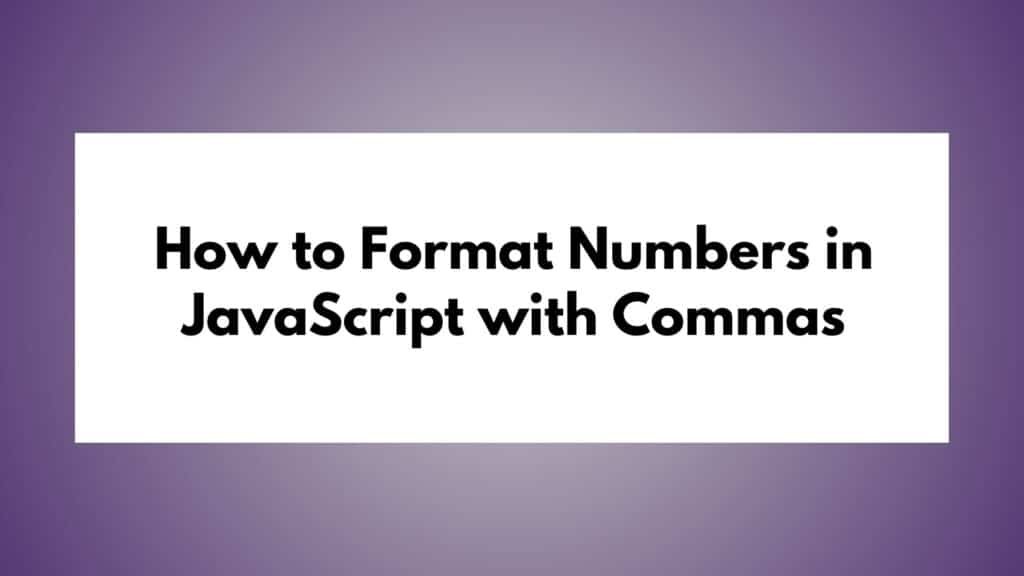
In this article, we will explore how to format numbers in JavaScript with commas. Formatting numbers is a common requirement in web development, especially when dealing with large numbers. By adding commas to numbers, we can enhance readability and make the data more user-friendly. This step-by-step guide will walk you through the process of formatting numbers with commas in JavaScript.
Table of Contents
What is Number Formatting?
Number formatting refers to the manipulation and presentation of numeric data in a desired format. It involves modifying the appearance of numbers to improve readability and comprehension. One common number formatting requirement is the inclusion of commas in large numbers, which helps users quickly grasp the magnitude of the value.
The Importance of Number Formatting
Properly formatted numbers play a crucial role in user experience. They make numerical data more accessible, easier to understand, and visually appealing. When it comes to displaying large numbers, adding commas can significantly enhance readability. By following the steps outlined below, you can easily format numbers with commas using JavaScript.
Formatting Numbers with Commas in JavaScript
To format numbers with commas in JavaScript, follow these steps:
Step 1: Converting the Number to a String
The first step is to convert the number to a string using the toString()
method. This allows us to manipulate the number as a string and add commas at appropriate positions.
let number = 1000000; let formattedNumber = number.toString();
Step 2: Adding Commas at Appropriate Positions
Next, we need to add commas at appropriate positions within the string representation of the number. We can achieve this by using regular expressions and the replace()
method.
formattedNumber = formattedNumber.replace(/\B(?=(\d{3})+(?!\d))/g, ",");
Step 3: Returning the Formatted Number
Finally, we can return the formatted number as a string with commas.
return formattedNumber;
Example Code: Formatting Numbers with Commas
function formatNumberWithCommas(number) { let formattedNumber = number.toString(); formattedNumber = formattedNumber.replace(/\B(?=(\d{3})+(?!\d))/g, ","); return formattedNumber; } let number = 10000005588; let formattedNumber = formatNumberWithCommas(number); console.log( "Output: " formattedNumber);
Result:
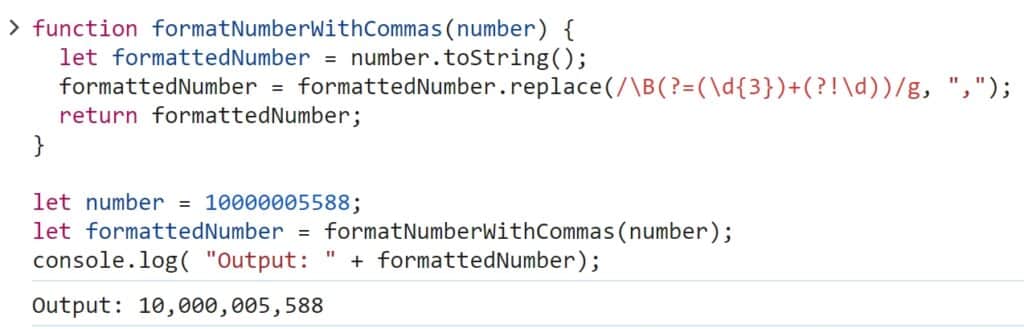
Conclusion
In this article, we have explored how to format numbers in JavaScript with commas. By following the step-by-step guide and example code provided, you can easily incorporate number formatting into your JavaScript projects. Adding commas to large numbers improves readability and enhances the user experience. Now you can effectively display numeric data with clarity and precision.