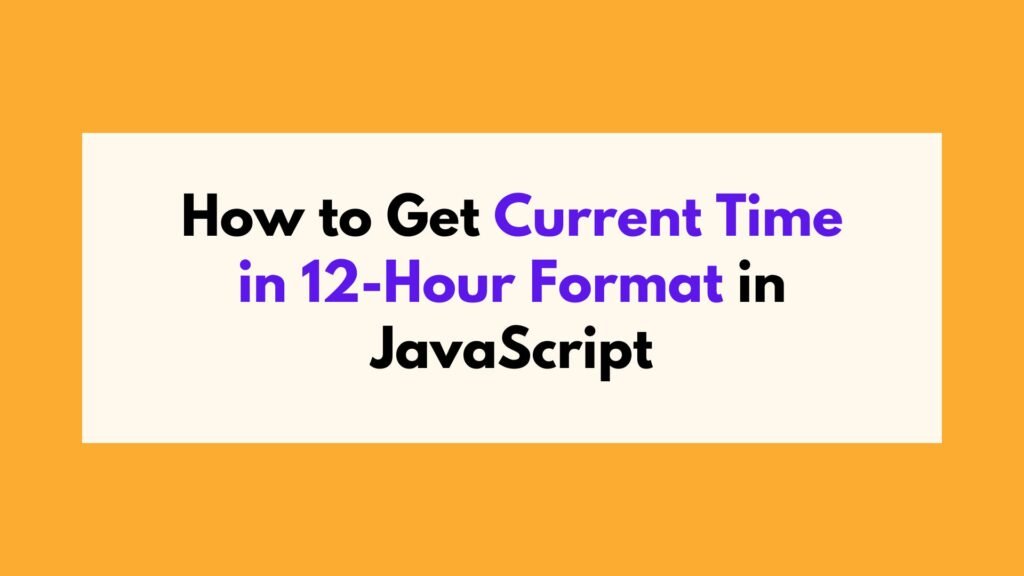
This article provides a comprehensive guide on how to obtain the current time in 12-hour format using JavaScript. It covers various techniques and examples to help you understand and implement this functionality in your projects.
When working with JavaScript, it is often necessary to retrieve the current time in different formats. In this article, we will focus on obtaining the current time in the 12-hour format. We will explore various methods and provide detailed explanations along with code examples to assist you in achieving this task
Table of Contents
Understanding the Concept of Time Formatting
Before we delve into the specific implementation details, let’s briefly discuss the concept of time formatting. In JavaScript, the Date
object provides numerous methods to work with dates and times. To obtain the current time, we can create a new Date
object and utilize its various functions to format the time according to our needs.
Getting the Current Time in 12-Hour Format
To get the current time in the 12-hour format, we can follow different approaches. Let’s explore a few popular methods:
Method 1: Utilizing the toLocaleTimeString()
Method
One way to obtain the current time in the desired format is by using the toLocaleTimeString()
method. This method returns a string representing the time portion of a Date
object according to the local time zone conventions.
const currentDate = new Date(); const currentTime = currentDate.toLocaleTimeString([], { hour: 'numeric', minute: 'numeric', hour12: true });

In this example, we create a new Date
object called currentDate
. We then call the toLocaleTimeString()
method on this object, passing an empty array as the first argument and an options object as the second argument. The hour
and minute
properties of the options object are set to 'numeric'
, which instructs JavaScript to display those values as numbers. The hour12
property is set to true
to enable 12-hour format.
Method 2: Using the getHours()
and getMinutes()
Methods
Another approach involves utilizing the getHours()
and getMinutes()
methods available on the Date
object. Let’s see how we can combine these methods to achieve our goal:
const currentDate = new Date(); let hours = currentDate.getHours(); let minutes = currentDate.getMinutes(); let meridiem = hours >= 12 ? 'PM' : 'AM'; // Adjusting hours to 12-hour format hours = hours % 12; hours = hours ? hours : 12; const currentTime = `${hours}:${minutes} ${meridiem}`;
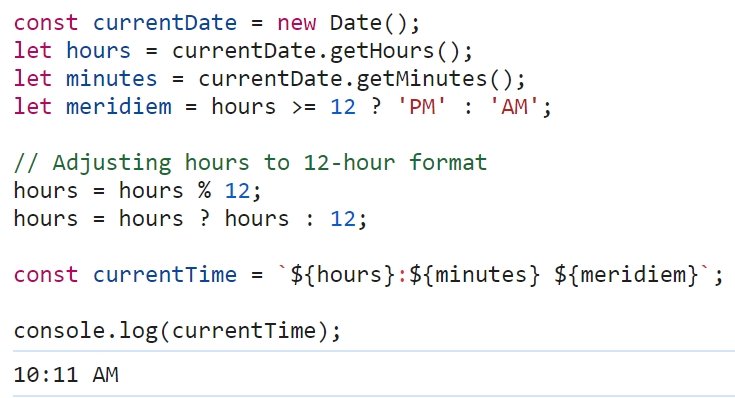
In this example, we create a new Date
object called currentDate
. We then extract the current hours and minutes using the getHours()
and getMinutes()
methods, respectively. Next, we determine whether it is before or after noon (12 o’clock) by checking if the hours are greater than or equal to 12. Based on this condition, we assign either 'PM'
or 'AM'
to the meridiem
variable. Finally, we adjust the hours to the 12-hour format by calculating the remainder when divided by 12. If the result is 0, we set it to 12.
Conclusion
Obtaining the current time in the 12-hour format using JavaScript is a common requirement in many applications. In this article, we explored two methods to achieve this: utilizing the toLocaleTimeString()
method and combining the getHours()
and getMinutes()
methods. We provided detailed explanations along with code examples for each method.
By following these techniques, you can easily incorporate the functionality of retrieving the current time in 12-hour format into your JavaScript projects. Remember to consider your specific requirements and choose the method that best suits your needs.
Start implementing these approaches today and enhance your applications with accurate and formatted time display!
Remember, understanding time formatting and leveraging JavaScript’s built-in functions will empower you to handle various date and time-related tasks efficiently.
Happy coding!