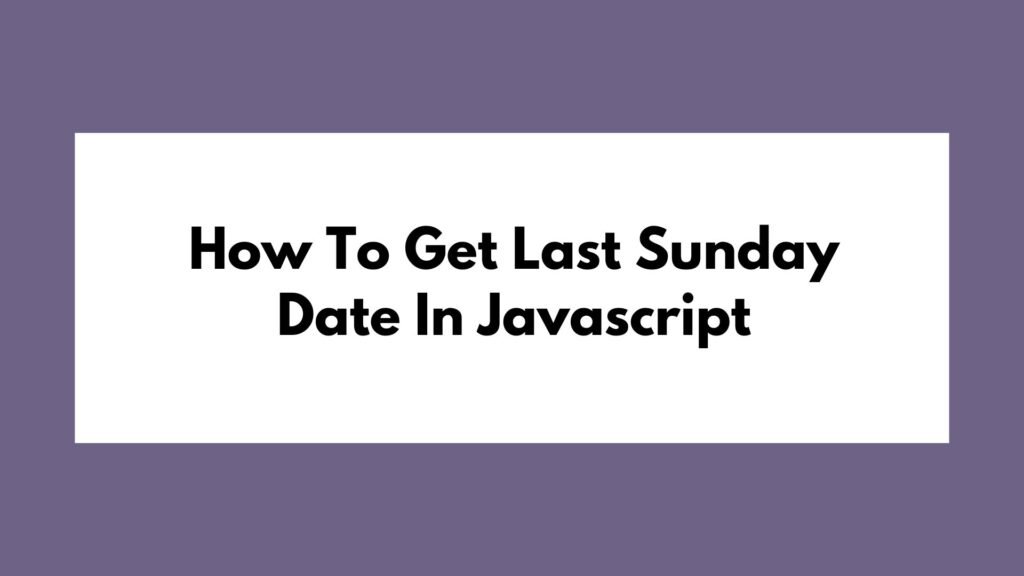
In JavaScript programming, working with dates is a common task. One specific scenario that may arise is the need to find the date of the last Sunday. This article will guide you through the process of obtaining the last Sunday date in JavaScript efficiently.
Steps to Get Last Sunday Date in JavaScript
To obtain the date of the last Sunday in JavaScript, follow these steps:
Step 1: Determine the Current Date
The first step is to get the current date using the Date
object in JavaScript.
const currentDate = new Date();
Step 2: Calculate the Day of the Week
Next, we need to determine the day of the week for the current date. In JavaScript, the getDay()
method returns the day of the week (0 for Sunday, 1 for Monday, and so on).
const currentDay = currentDate.getDay();
Step 3: Calculate the Offset to Last Sunday
To find the date of the last Sunday, we calculate the offset by subtracting the current day of the week from 0 (for Sunday) and add 7.
const offsetToLastSunday = (currentDay + 7) % 7;
Step 4: Calculate Last Sunday Date
Finally, we subtract the offset from the current date to get the date of the last Sunday.
const lastSundayDate = new Date(currentDate); lastSundayDate.setDate(currentDate.getDate() - offsetToLastSunday);
Full Code Snippet
Here is the complete code snippet to get the last Sunday date in JavaScript:
const currentDate = new Date(); const currentDay = currentDate.getDay(); const offsetToLastSunday = (currentDay + 7) % 7; const lastSundayDate = new Date(currentDate); lastSundayDate.setDate(currentDate.getDate() - offsetToLastSunday); console.log(lastSundayDate);
By following these steps and using the provided code snippet, you can accurately determine the date of the last Sunday in JavaScript.
Conclusion
In conclusion, obtaining the last Sunday date in JavaScript involves simple calculations based on the current date. By understanding these concepts and implementing the provided code snippets, you can efficiently retrieve the desired date. Incorporating such functionalities adds value and versatility to your JavaScript applications.
Happy coding!