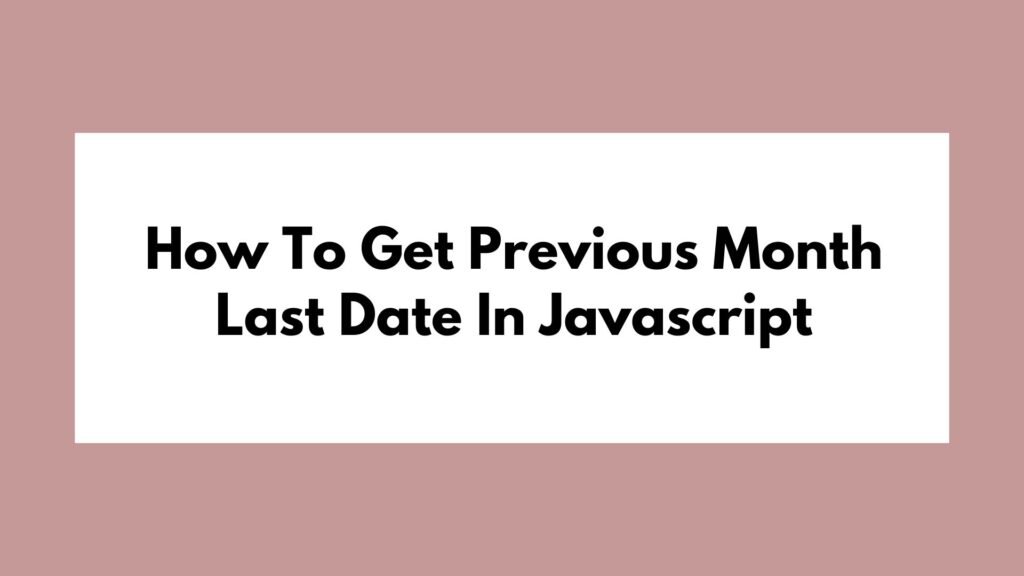
In JavaScript, retrieving the last date of the previous month can be a handy task in various scenarios. Whether you’re working on a financial application, scheduling system, or any other project that requires date manipulation, knowing how to get the last date of the previous month programmatically is crucial. In this article, we will explore different approaches to achieve this in JavaScript.
One straightforward method to obtain the last date of the previous month is by utilizing the JavaScript Date
object. Here’s a step-by-step guide to achieving this:
Step 1: Create a Date Object for the Current Date
Firstly, we need to create a Date
object for the current date:
const currentDate = new Date();
Step 2: Set the Date to 0 of the Current Date
Next, we set the date to 0 of the current date. This action effectively moves us back to the last day of the previous month:
currentDate.setDate(0);
Step 3: Format the Date with Day Name
Format the date to include the day name using JavaScript’s toLocaleString
method:
const options = { weekday: 'long', year: 'numeric', month: 'long', day: 'numeric' }; const formattedDate = currentDate.toLocaleString('en-US', options);
Now, formattedDate
will store the last date of the previous month with the day name in a human-readable format.
By following these steps, you can accurately determine the last date of the previous month in JavaScript.
Example Usage
Let’s put this method into practice with a practical example:
const currentDate = new Date(); currentDate.setDate(0); const options = { weekday: 'long', year: 'numeric', month: 'long', day: 'numeric' }; const formattedDate = currentDate.toLocaleString('en-US', options); console.log(`The last date of the previous month with day name is: ${formattedDate}`);
In this example, formattedDate
will showcase the last date of the month before the current one along with the respective day name.
Result:

Conclusion
In conclusion, obtaining the last date of the previous month in JavaScript can be achieved through simple manipulations using the Date
object. By following the steps outlined in this article, you can seamlessly integrate this functionality into your projects, enhancing date-related operations.